Day -24:Types of Inheritance
Types of Inheritance in Java Inheritance is the most powerful feature of object-oriented programming. It allows us to inherit the properties of one class into another class. In this section, we will discuss types of inheritance in Java in-depth with real-life examples. Also, we will create Java programs to implement the concept of different types of inheritance. Inheritance is a mechanism of driving a new class from an existing class. The existing (old) class is known as base class or super class or parent class. The new class is known as a derived class or sub class or child class. It allows us to use the properties and behavior of one class (parent) in another class (child). Types of Inheritance Java supports the following four types of inheritance: Single Inheritance Multi-level Inheritance Hierarchical Inheritance Hybrid Inheritance Single Inheritance In single inheritance, a sub-class is derived from only one super class. It inherits the properties and behavior of a single-parent class. Sometimes it is also known as simple inheritance. class Employee { float salary=34534*12; } public class Executive extends Employee { float bonus=3000*6; public static void main(String args[]) { Executive obj=new Executive(); System.out.println("Total salary credited: "+obj.salary); System.out.println("Bonus of six months: "+obj.bonus); } } output Total salary credited: 414408.0 Bonus of six months: 18000.0 Multi-level Inheritance In multi-level inheritance, a class is derived from a class which is also derived from another class is called multi-level inheritance. In simple words, we can say that a class that has more than one parent class is called multi-level inheritance. Note that the classes must be at different levels. Hence, there exists a single base class and single derived class but multiple intermediate base classes. // Base class class Animal { void eat() { System.out.println("This animal eats food."); } } // Derived class class Dog extends Animal { void bark() { System.out.println("The dog barks."); } } // Another derived class (Multilevel) class Puppy extends Dog { void weep() { System.out.println("The puppy weeps."); } } // Main class to run the program public class Main { public static void main(String[] args) { Puppy myPuppy = new Puppy(); myPuppy.eat(); // from Animal class myPuppy.bark(); // from Dog class myPuppy.weep(); // from Puppy class } } output This animal eats food. The dog barks. The puppy weeps. Hierarchical Inheritance If a number of classes are derived from a single base class, it is called hierarchical inheritance. // Base class class Animal { void eat() { System.out.println("This animal eats food."); } } // First subclass class Dog extends Animal { void bark() { System.out.println("The dog barks."); } } // Second subclass class Cat extends Animal { void meow() { System.out.println("The cat meows."); } } // Main class public class Main { public static void main(String[] args) { Dog dog = new Dog(); Cat cat = new Cat(); dog.eat(); // from Animal dog.bark(); // from Dog cat.eat(); // from Animal cat.meow(); // from Cat } } Output: This animal eats food. The dog barks. This animal eats food. The cat meows. Hybrid Inheritance Hybrid means consist of more than one. Hybrid inheritance is the combination of two or more types of inheritance. Multilevel Inheritance Hierarchical Inheritance // Interface 1 interface Printable { void print(); } // Interface 2 interface Showable { void show(); } // Base class class Base { void display() { System.out.println("Display from Base class."); } } // Child class implementing multiple interfaces and inheriting from Base class class Hybrid extends Base implements Printable, Showable { public void print() { System.out.println("Printing..."); } public void show() { System.out.println("Showing..."); } } // Main class public class Main { public static void main(String[] args) { Hybrid obj = new Hybrid(); obj.display(); // from Base class obj.print(); // from Printable interface obj.show(); // from Showable interface } } Output: Display from Base class. Printing... Showing... Reference link: https://www.tpointtech.com/types-of-inheritance-in-java
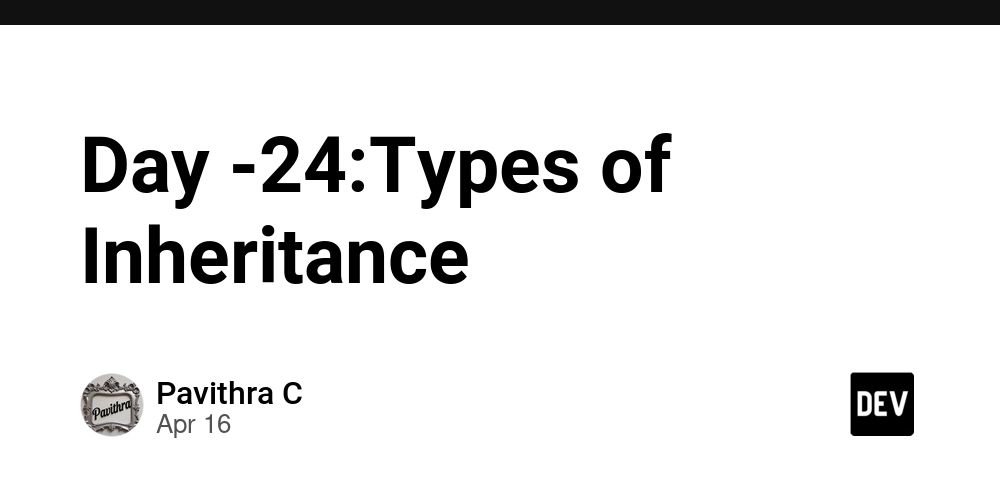
Types of Inheritance in Java
Inheritance is the most powerful feature of object-oriented programming. It allows us to inherit the properties of one class into another class. In this section, we will discuss types of inheritance in Java in-depth with real-life examples. Also, we will create Java programs to implement the concept of different types of inheritance.
Inheritance is a mechanism of driving a new class from an existing class. The existing (old) class is known as base class or super class or parent class. The new class is known as a derived class or sub class or child class. It allows us to use the properties and behavior of one class (parent) in another class (child).
Types of Inheritance
Java supports the following four types of inheritance:
- Single Inheritance
- Multi-level Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Single Inheritance
In single inheritance, a sub-class is derived from only one super class. It inherits the properties and behavior of a single-parent class. Sometimes it is also known as simple inheritance.
class Employee
{
float salary=34534*12;
}
public class Executive extends Employee
{
float bonus=3000*6;
public static void main(String args[])
{
Executive obj=new Executive();
System.out.println("Total salary credited: "+obj.salary);
System.out.println("Bonus of six months: "+obj.bonus);
}
}
output
Total salary credited: 414408.0
Bonus of six months: 18000.0
Multi-level Inheritance
In multi-level inheritance, a class is derived from a class which is also derived from another class is called multi-level inheritance. In simple words, we can say that a class that has more than one parent class is called multi-level inheritance. Note that the classes must be at different levels. Hence, there exists a single base class and single derived class but multiple intermediate base classes.
// Base class
class Animal {
void eat() {
System.out.println("This animal eats food.");
}
}
// Derived class
class Dog extends Animal {
void bark() {
System.out.println("The dog barks.");
}
}
// Another derived class (Multilevel)
class Puppy extends Dog {
void weep() {
System.out.println("The puppy weeps.");
}
}
// Main class to run the program
public class Main {
public static void main(String[] args) {
Puppy myPuppy = new Puppy();
myPuppy.eat(); // from Animal class
myPuppy.bark(); // from Dog class
myPuppy.weep(); // from Puppy class
}
}
output
This animal eats food.
The dog barks.
The puppy weeps.
Hierarchical Inheritance
If a number of classes are derived from a single base class, it is called hierarchical inheritance.
// Base class
class Animal {
void eat() {
System.out.println("This animal eats food.");
}
}
// First subclass
class Dog extends Animal {
void bark() {
System.out.println("The dog barks.");
}
}
// Second subclass
class Cat extends Animal {
void meow() {
System.out.println("The cat meows.");
}
}
// Main class
public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
Cat cat = new Cat();
dog.eat(); // from Animal
dog.bark(); // from Dog
cat.eat(); // from Animal
cat.meow(); // from Cat
}
}
Output:
This animal eats food.
The dog barks.
This animal eats food.
The cat meows.
Hybrid Inheritance
Hybrid means consist of more than one. Hybrid inheritance is the combination of two or more types of inheritance.
- Multilevel Inheritance
- Hierarchical Inheritance
// Interface 1
interface Printable {
void print();
}
// Interface 2
interface Showable {
void show();
}
// Base class
class Base {
void display() {
System.out.println("Display from Base class.");
}
}
// Child class implementing multiple interfaces and inheriting from Base class
class Hybrid extends Base implements Printable, Showable {
public void print() {
System.out.println("Printing...");
}
public void show() {
System.out.println("Showing...");
}
}
// Main class
public class Main {
public static void main(String[] args) {
Hybrid obj = new Hybrid();
obj.display(); // from Base class
obj.print(); // from Printable interface
obj.show(); // from Showable interface
}
}
Output:
Display from Base class.
Printing...
Showing...
Reference link:
https://www.tpointtech.com/types-of-inheritance-in-java