day-19: Java Access Modifiers - Simplified
Encapsulation is one of the four fundamental OOP principles (along with Inheritance, Polymorphism, and Abstraction). It refers to bundling data (variables) and methods (functions) that operate on that data into a single unit (class), while restricting direct access to some of the object's components. Types of Encapsulation: Java Access Modifiers - Simplified 1). private Access Level: Only within the same class Example: private int salary; // Only accessible in this class Use Case: Hide sensitive data (e.g., passwords, internal calculations). 2). default (No Modifier) Access Level: Only within the same package Example: String department; // Accessible only in this package 3). protected Access Level: Same package + Subclasses (even in different packages) Example: protected String projectName; // Accessible in package + child classes 4). public Access Level: Everywhere (global access) Example: public String companyName = "TechCorp"; // Accessible by any class Use Case: APIs, constants, or methods needed globally. Note: public static void main(String[] args) - //Method Signature Access Specifier not for local variables When to Use Which? private: Hide internal implementation details. default: Share code within a package only. protected: Allow controlled inheritance. public: Expose APIs/constants. Example: public class Employee { private int id; // Only this class String name; // Package-only (default) protected String role; // Package + child classes public static final String COMPANY = "TechCorp"; // Global } Class Example: public class Google { private int emp_salary; public static String ho_address = "Mountain View"; public static boolean working = true; private void get_user_details() { int no = 10; System.out.println("All Users Details"); } public void search_Results() { System.out.println("Searching Results"); } } public class User { public static void main(String[] args) { //Method Signature Google googleObj = new Google() ; //System.out.println(googleObj.emp_salary); System.out.println(Google.working); System.out.println(Google.ho_address); //googleObj.get_user_details(); googleObj.search_Results(); User.main(); } public static void main(){ System.out.println("Overloaded Main Method"); } } Key Rules Class-level: Only public or default (no private/protected). Variables/Methods: All 4 modifiers allowed. Security Tip: Start with private, widen access only when needed. This ensures encapsulation while providing flexibility.
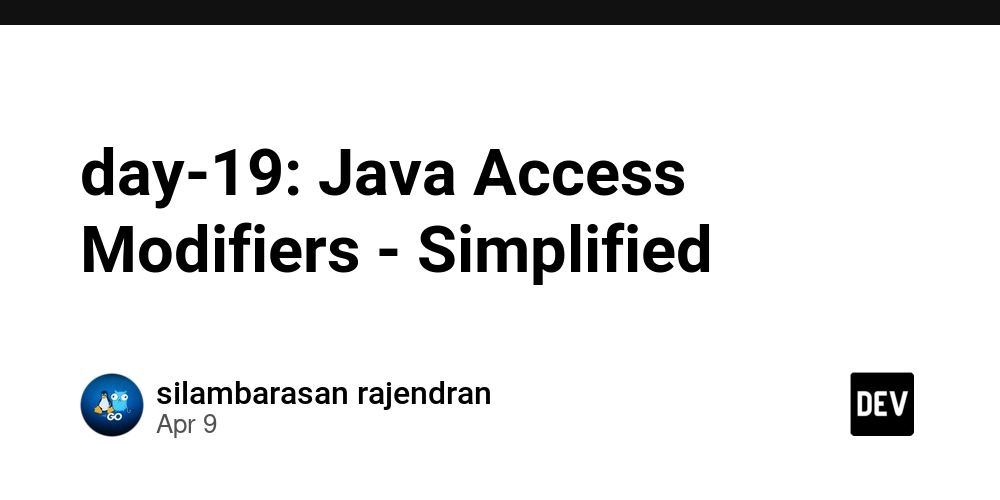
Encapsulation is one of the four fundamental OOP principles (along with Inheritance, Polymorphism, and Abstraction). It refers to bundling data (variables) and methods (functions) that operate on that data into a single unit (class), while restricting direct access to some of the object's components.
Types of Encapsulation:
Java Access Modifiers - Simplified
1). private
Access Level: Only within the same class
Example:
private int salary; // Only accessible in this class
Use Case: Hide sensitive data (e.g., passwords, internal calculations).
2). default (No Modifier)
Access Level: Only within the same package
Example:
String department; // Accessible only in this package
3). protected
Access Level:
Same package +
Subclasses (even in different packages)
Example:
protected String projectName; // Accessible in package + child classes
4). public
Access Level: Everywhere (global access)
Example:
public String companyName = "TechCorp"; // Accessible by any class
Use Case: APIs, constants, or methods needed globally.
Note:
public static void main(String[] args) - //Method Signature
Access Specifier not for local variables
When to Use Which?
private: Hide internal implementation details.
default: Share code within a package only.
protected: Allow controlled inheritance.
public: Expose APIs/constants.
Example:
public class Employee {
private int id; // Only this class
String name; // Package-only (default)
protected String role; // Package + child classes
public static final String COMPANY = "TechCorp"; // Global
}
Class Example:
public class Google {
private int emp_salary;
public static String ho_address = "Mountain View";
public static boolean working = true;
private void get_user_details() {
int no = 10;
System.out.println("All Users Details");
}
public void search_Results() {
System.out.println("Searching Results");
}
}
public class User {
public static void main(String[] args) { //Method Signature
Google googleObj = new Google() ;
//System.out.println(googleObj.emp_salary);
System.out.println(Google.working);
System.out.println(Google.ho_address);
//googleObj.get_user_details();
googleObj.search_Results();
User.main();
}
public static void main(){
System.out.println("Overloaded Main Method");
}
}
Key Rules
Class-level: Only public or default (no private/protected).
Variables/Methods: All 4 modifiers allowed.
Security Tip: Start with private, widen access only when needed.
This ensures encapsulation while providing flexibility.