Custom Theming in Ant Design v5 with Dynamic Theme Switching
Ant Design v5 introduced a powerful theming system built around design tokens, making it easier than ever to apply consistent styling across your entire app from a single configuration. In this blog, we'll explore how to create a custom global theme, override component-specific styles, and implement support for multiple dynamic themes using React. Why Theming Matters A unified design language makes your app look professional and easier to maintain. With v5, AntD replaces LESS variables with Component Tokens and Global Tokens which are configurable via React context using ConfigProvider. Step 1: Set Up Ant Design v5 Install the necessary packages: npm install antd Import styles in your main entry file (e.g., main.tsx or index.tsx): import 'antd/dist/reset.css'; Step 2: Define Global and Component Tokens Create a file to store your theme configuration: // theme.ts export const lightTheme = { token: { colorPrimary: '#00b96b', borderRadius: 8, fontSize: 14, }, components: { Button: { colorPrimary: '#00b96b', controlHeight: 40, }, Checkbox: { colorPrimary: '#1890ff', }, }, }; export const darkTheme = { token: { colorPrimary: '#722ed1', colorBgBase: '#141414', colorText: '#ffffff', }, components: { Button: { colorPrimary: '#722ed1', }, }, }; Step 3: Implement Theme Switcher Use React Context or simple state to toggle themes: // App.tsx import React, { useState } from 'react'; import { ConfigProvider, Button, Checkbox } from 'antd'; import { lightTheme, darkTheme } from './theme'; const App: React.FC = () => { const [isDarkMode, setIsDarkMode] = useState(false); return ( setIsDarkMode(!isDarkMode)}> Toggle Theme Sample Checkbox ); }; export default App; Bonus: Create More Themes Easily Want a high-contrast, pastel, or corporate theme? Just create new theme objects following the same structure and add toggling logic for them. export const pastelTheme = { token: { colorPrimary: '#ff85c0', borderRadius: 16, }, components: { Button: { colorPrimary: '#ff85c0', }, }, }; Final Thoughts Ant Design v5's token system allows for centralized, scalable, and powerful styling management. With dynamic switching, you can offer users light/dark mode or even let them pick their favorite theme. Build your own design system with full control — all from a single place.
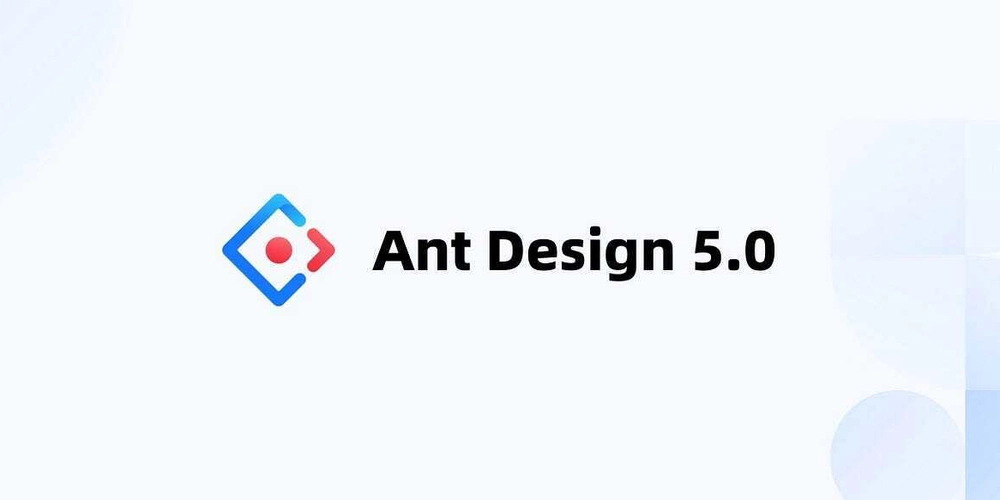
Ant Design v5 introduced a powerful theming system built around design tokens, making it easier than ever to apply consistent styling across your entire app from a single configuration. In this blog, we'll explore how to create a custom global theme, override component-specific styles, and implement support for multiple dynamic themes using React.
Why Theming Matters
A unified design language makes your app look professional and easier to maintain. With v5, AntD replaces LESS variables with Component Tokens and Global Tokens which are configurable via React context using ConfigProvider
.
Step 1: Set Up Ant Design v5
Install the necessary packages:
npm install antd
Import styles in your main entry file (e.g., main.tsx
or index.tsx
):
import 'antd/dist/reset.css';
Step 2: Define Global and Component Tokens
Create a file to store your theme configuration:
// theme.ts
export const lightTheme = {
token: {
colorPrimary: '#00b96b',
borderRadius: 8,
fontSize: 14,
},
components: {
Button: {
colorPrimary: '#00b96b',
controlHeight: 40,
},
Checkbox: {
colorPrimary: '#1890ff',
},
},
};
export const darkTheme = {
token: {
colorPrimary: '#722ed1',
colorBgBase: '#141414',
colorText: '#ffffff',
},
components: {
Button: {
colorPrimary: '#722ed1',
},
},
};
Step 3: Implement Theme Switcher
Use React Context or simple state to toggle themes:
// App.tsx
import React, { useState } from 'react';
import { ConfigProvider, Button, Checkbox } from 'antd';
import { lightTheme, darkTheme } from './theme';
const App: React.FC = () => {
const [isDarkMode, setIsDarkMode] = useState(false);
return (
<ConfigProvider theme={isDarkMode ? darkTheme : lightTheme}>
<div style={{ padding: 20 }}>
<Button onClick={() => setIsDarkMode(!isDarkMode)}>
Toggle Theme
Button>
<div style={{ marginTop: 20 }}>
<Checkbox>Sample CheckboxCheckbox>
div>
div>
ConfigProvider>
);
};
export default App;
Bonus: Create More Themes Easily
Want a high-contrast, pastel, or corporate theme? Just create new theme objects following the same structure and add toggling logic for them.
export const pastelTheme = {
token: {
colorPrimary: '#ff85c0',
borderRadius: 16,
},
components: {
Button: {
colorPrimary: '#ff85c0',
},
},
};
Final Thoughts
Ant Design v5's token system allows for centralized, scalable, and powerful styling management. With dynamic switching, you can offer users light/dark mode or even let them pick their favorite theme.
Build your own design system with full control — all from a single place.