Custom Error Pages in .Net Core
1. Enable Custom Error Pages in Startup.cs Configure Custom Error Handling Middleware In your Startup.cs file, configure the error handling middleware to show custom error pages for different status codes (e.g., 404, 500). You’ll typically use the UseStatusCodePagesWithReExecute middleware for custom error pages. public void Configure(IApplicationBuilder app, IHostingEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); // Detailed error in development } else { // Custom error pages for non-development environments app.UseExceptionHandler("/Error/GeneralError"); app.UseStatusCodePagesWithReExecute("/Error/{0}"); } app.UseStaticFiles(); // Serve static files app.UseMvcWithDefaultRoute(); // MVC routing } Custom Error Controller Create an ErrorController that will handle different error types. The {0} in the path passed to UseStatusCodePagesWithReExecute corresponds to the HTTP status code, such as 404 or 500. public class ErrorController : Controller { public IActionResult GeneralError() { return View("GeneralError"); } public IActionResult Error404() { return View("404"); } public IActionResult Error500() { return View("500"); } } Create the corresponding views under Views/Error for each error page (e.g., 404.cshtml, 500.cshtml, and GeneralError.cshtml). Example Folder Structure /Views/Error/ ├── 404.cshtml ├── 500.cshtml └── GeneralError.cshtml
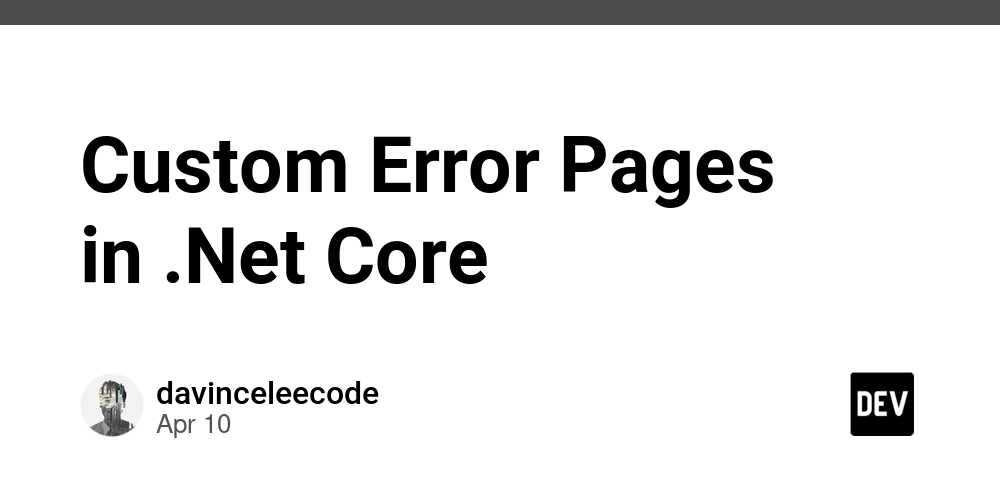
1. Enable Custom Error Pages in Startup.cs
Configure Custom Error Handling Middleware
In your Startup.cs
file, configure the error handling middleware to show custom error pages for different status codes (e.g., 404, 500). You’ll typically use the UseStatusCodePagesWithReExecute
middleware for custom error pages.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage(); // Detailed error in development
}
else
{
// Custom error pages for non-development environments
app.UseExceptionHandler("/Error/GeneralError");
app.UseStatusCodePagesWithReExecute("/Error/{0}");
}
app.UseStaticFiles(); // Serve static files
app.UseMvcWithDefaultRoute(); // MVC routing
}
Custom Error Controller
Create an ErrorController
that will handle different error types. The {0}
in the path passed to UseStatusCodePagesWithReExecute
corresponds to the HTTP status code, such as 404 or 500.
public class ErrorController : Controller
{
public IActionResult GeneralError()
{
return View("GeneralError");
}
public IActionResult Error404()
{
return View("404");
}
public IActionResult Error500()
{
return View("500");
}
}
Create the corresponding views under Views/Error
for each error page (e.g., 404.cshtml
, 500.cshtml
, and GeneralError.cshtml
).
Example Folder Structure
/Views/Error/
├── 404.cshtml
├── 500.cshtml
└── GeneralError.cshtml