Complete Interview
C++ 1. C vs C++ C++ is a superset of C, offering OOP, exception handling, and better memory management,STL making it more versatile 2. Signed vs Unsigned Overflow in signed integers results in undefined behavior. Overflow in unsigned integers wraps back to zero. Signed integers: Support negative values. Unsigned integers: Only support positive values but have higher range 3. What is reference variable? Unlike pointers, references cannot be null and must be initialized. int* ptr = nullptr; // Valid int& ref = nullptr; // Invalid (won't compile) int x = 10; int& ref = x; // Valid int& ref2; // Invalid (must be initialized) 4. sizeof Operator Returns the size (in bytes) of a variable or data type. 5. typedef in C++ typedef is a keyword in C++ (and C) used to create type aliases. It allows you to define a new name for an existing type, making code more readable and maintainable. typedef unsigned int uint; uint age = 25; // Equivalent to unsigned int age = 25; 6. OOPs A way of writing programs by creating objects. An object is an instance of class that has properties (data) and behavior (actions) A class is like a template or blueprint that defines the structure of an object. Objects are created from classes. We use oops to make programs simple & organized, make the code reusable, Better for big projects Pillars: Inheritance - Inheritance allows one class to take features (properties & actions) from another class. A "Vehicle" class has wheels and engine. A "Car" class can inherit this and add extra features like doors, air conditioning, etc.
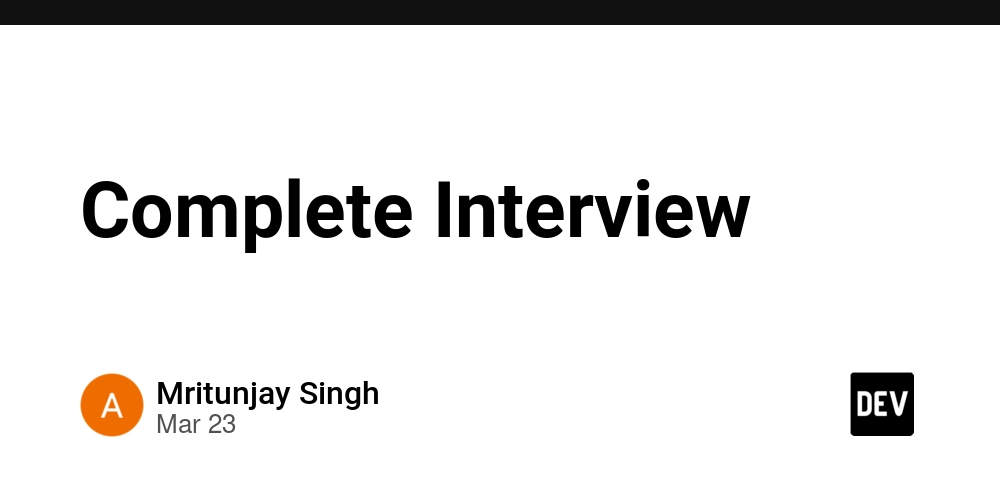
C++
1. C vs C++
C++ is a superset of C, offering OOP, exception handling, and better memory management,STL making it more versatile
2. Signed vs Unsigned
Overflow in signed integers results in undefined behavior.
Overflow in unsigned integers wraps back to zero.
Signed integers: Support negative values.
Unsigned integers: Only support positive values but have higher range
3. What is reference variable?
Unlike pointers, references cannot be null and must be initialized.
int* ptr = nullptr; // Valid
int& ref = nullptr; // Invalid (won't compile)
int x = 10;
int& ref = x; // Valid
int& ref2; // Invalid (must be initialized)
4. sizeof Operator
Returns the size (in bytes) of a variable or data type.
5. typedef in C++
typedef is a keyword in C++ (and C) used to create type aliases. It allows you to define a new name for an existing type, making code more readable and maintainable.
typedef unsigned int uint;
uint age = 25; // Equivalent to unsigned int age = 25;
6. OOPs
A way of writing programs by creating objects. An object is an instance of class that has properties (data) and behavior (actions)
A class is like a template or blueprint that defines the structure of an object. Objects are created from classes.
We use oops to make programs simple & organized, make the code reusable, Better for big projects
Pillars:
Inheritance - Inheritance allows one class to take features (properties & actions) from another class.
A "Vehicle" class has wheels and engine. A "Car" class can inherit this and add extra features like doors, air conditioning, etc.