CI/CD with Azure DevOps and git submodule
This setup is designed for Azure DevOps Pipelines, leveraging its built-in tasks and authentication mechanisms to manage Git submodules efficiently. Managing Git submodules in CI/CD pipelines can be challenging. One moment, everything is running smoothly, and the next, you're troubleshooting broken dependencies and failed builds. If you've ever struggled to keep submodules synchronized while maintaining a reliable Maven build, you're not alone. To address these challenges, I’ve implemented a structured approach that ensures consistency, minimizes manual intervention, and enhances automation in our CI/CD workflows. Let’s explore the solution. The Challenge When working with a monorepo or a collection of interdependent repositories, handling Git submodules efficiently is crucial. The key requirements for an optimal workflow include: Ensuring the correct branch is checked out for the main repository. Keeping submodules updated with a stable reference (defaulting to the develop branch) unless a matching branch exists. Executing a complete Maven build, including tests and code coverage. Automating the process to eliminate manual updates and reduce the risk of human error. Updating Submodules Dynamically To dynamically align submodules with the appropriate branch, we check whether a branch exists in the submodule that corresponds to the main repository's branch. If it does, we use it; otherwise, we default to develop. - task: Bash@3 displayName: 'Update submodules' inputs: targetType: 'inline' workingDirectory: $(Build.SourcesDirectory) script: | echo "Repository structure details:" echo "--------------------------" echo "Main repository:" cd $(Build.SourcesDirectory)/primary-repo git status echo "--------------------------" export MY_PAT=$(System.AccessToken) export HEADER_VALUE=$(echo -n "Authorization: Basic "$(printf ":%s" "$MY_PAT" | base64)) git config --global http.extraheader "$HEADER_VALUE" git config --global pull.rebase false FULL_BRANCH_NAME=$(echo $(Build.SourceBranch) | sed 's|refs/heads/||') echo "Full branch name: $FULL_BRANCH_NAME" echo "--------------------------" cd $(Build.SourcesDirectory)/common-module if git fetch origin $FULL_BRANCH_NAME && git rev-parse --verify origin/$FULL_BRANCH_NAME >/dev/null 2>&1; then echo "Using branch: $FULL_BRANCH_NAME" git reset --hard origin/$FULL_BRANCH_NAME else echo "Branch $FULL_BRANCH_NAME not found, using develop instead" git fetch origin develop git reset --hard origin/develop fi cd $(Build.SourcesDirectory)/module-a git fetch origin develop git reset --hard origin/develop cd $(Build.SourcesDirectory)/module-b git fetch origin develop git reset --hard origin/develop cd $(Build.SourcesDirectory)/primary-repo echo "Submodule Status:" git submodule status Key Benefits of This Approach Automatic branch detection: Ensures submodules follow the correct branch when available. Graceful fallback: Defaults to develop when the expected branch is missing. Reliable synchronization: Prevents inconsistencies in submodule states. Improved automation: Reduces manual intervention, leading to a more efficient development workflow. Final Thoughts By implementing this automated submodule management strategy, we achieve: Consistent and predictable builds, minimizing unexpected failures. Seamless integration of submodules, ensuring they remain in sync with the main repository. Reduced manual intervention, allowing developers to focus on feature development rather than submodule maintenance. Improved CI/CD efficiency, enhancing reliability and maintainability of our pipelines. This structured approach streamlines the handling of Git submodules, reinforcing best practices in modern CI/CD workflows. With these enhancements, our build process becomes more resilient, scalable, and aligned with industry standards.
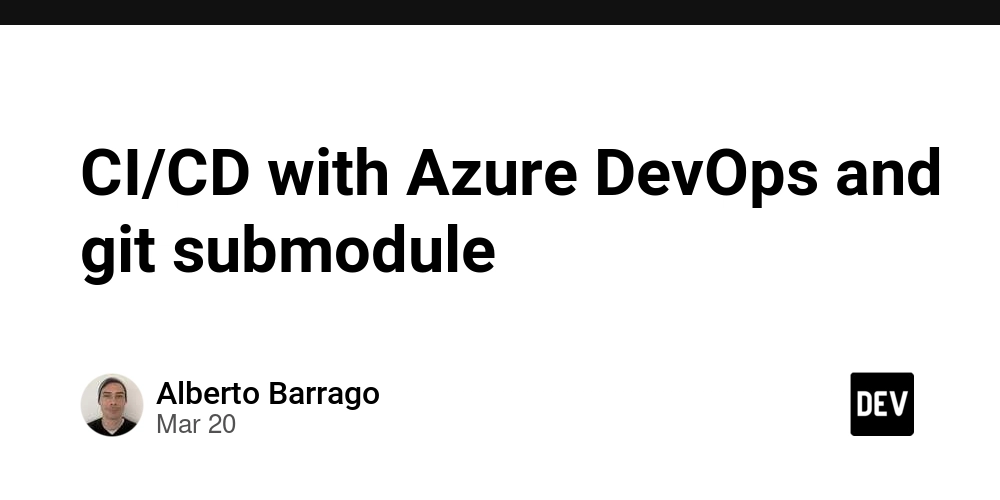
This setup is designed for Azure DevOps Pipelines, leveraging its built-in tasks and authentication mechanisms to manage Git submodules efficiently.
Managing Git submodules in CI/CD pipelines can be challenging. One moment, everything is running smoothly, and the next, you're troubleshooting broken dependencies and failed builds. If you've ever struggled to keep submodules synchronized while maintaining a reliable Maven build, you're not alone.
To address these challenges, I’ve implemented a structured approach that ensures consistency, minimizes manual intervention, and enhances automation in our CI/CD workflows. Let’s explore the solution.
The Challenge
When working with a monorepo or a collection of interdependent repositories, handling Git submodules efficiently is crucial. The key requirements for an optimal workflow include:
Ensuring the correct branch is checked out for the main repository.
Keeping submodules updated with a stable reference (defaulting to the develop branch) unless a matching branch exists.
Executing a complete Maven build, including tests and code coverage.
Automating the process to eliminate manual updates and reduce the risk of human error.
Updating Submodules Dynamically
To dynamically align submodules with the appropriate branch, we check whether a branch exists in the submodule that corresponds to the main repository's branch. If it does, we use it; otherwise, we default to develop.
- task: Bash@3
displayName: 'Update submodules'
inputs:
targetType: 'inline'
workingDirectory: $(Build.SourcesDirectory)
script: |
echo "Repository structure details:"
echo "--------------------------"
echo "Main repository:"
cd $(Build.SourcesDirectory)/primary-repo
git status
echo "--------------------------"
export MY_PAT=$(System.AccessToken)
export HEADER_VALUE=$(echo -n "Authorization: Basic "$(printf ":%s" "$MY_PAT" | base64))
git config --global http.extraheader "$HEADER_VALUE"
git config --global pull.rebase false
FULL_BRANCH_NAME=$(echo $(Build.SourceBranch) | sed 's|refs/heads/||')
echo "Full branch name: $FULL_BRANCH_NAME"
echo "--------------------------"
cd $(Build.SourcesDirectory)/common-module
if git fetch origin $FULL_BRANCH_NAME && git rev-parse --verify origin/$FULL_BRANCH_NAME >/dev/null 2>&1; then
echo "Using branch: $FULL_BRANCH_NAME"
git reset --hard origin/$FULL_BRANCH_NAME
else
echo "Branch $FULL_BRANCH_NAME not found, using develop instead"
git fetch origin develop
git reset --hard origin/develop
fi
cd $(Build.SourcesDirectory)/module-a
git fetch origin develop
git reset --hard origin/develop
cd $(Build.SourcesDirectory)/module-b
git fetch origin develop
git reset --hard origin/develop
cd $(Build.SourcesDirectory)/primary-repo
echo "Submodule Status:"
git submodule status
Key Benefits of This Approach
Automatic branch detection: Ensures submodules follow the correct branch when available.
Graceful fallback: Defaults to develop when the expected branch is missing.
Reliable synchronization: Prevents inconsistencies in submodule states.
Improved automation: Reduces manual intervention, leading to a more efficient development workflow.
Final Thoughts
By implementing this automated submodule management strategy, we achieve:
- Consistent and predictable builds, minimizing unexpected failures.
- Seamless integration of submodules, ensuring they remain in sync with the main repository.
- Reduced manual intervention, allowing developers to focus on feature development rather than submodule maintenance.
- Improved CI/CD efficiency, enhancing reliability and maintainability of our pipelines.
This structured approach streamlines the handling of Git submodules, reinforcing best practices in modern CI/CD workflows. With these enhancements, our build process becomes more resilient, scalable, and aligned with industry standards.