Building Your Own Web Server: Part 1 — Theory and Foundations
Introduction This is Part 1 of a multi-part series where we will build a complete HTTP web server from scratch. In this series of articles, I want to show how to create an HTTP web server from scratch. The goal isn’t to build a production-ready system — it’s to explore different approaches to implementing a server: starting from a simple, naive, single-threaded server and moving toward asynchronous, non-blocking designs similar to those used by modern high-performance servers like Nginx. At each step, I’ll measure performance and share the results with you. The main motivation behind this article is to take a deep look under the hood of how web servers work and to truly understand the fundamental concepts involved. When I started writing, I had two goals in mind: Understand it thoroughly myself. Explain it clearly to you, step-by-step. However, as I dove deeper, I realized that building a server also touches on many other foundational topics — like sockets, the HTTP protocol, and concurrency. This led me down a bit of a rabbit hole! I had to make some choices about how deep to go into each topic without overwhelming you or oversimplifying important concepts. Because of this, I’ve structured the article into two main parts: Theory — covering everything we need to know before we start coding. Implementation — where we’ll build different versions of our server. Feel free to skim the theory if you’re already familiar with some topics, but I highly recommend at least glancing through it, as I’ll refer back to key concepts throughout the implementation stages. In theory section we will learn: What a web server is and what it does How HTTP defines the rules of communication Why sockets are essential for network connections The structure of an HTTP message How persistent connections impact parsing What functionality we need to implement in our server
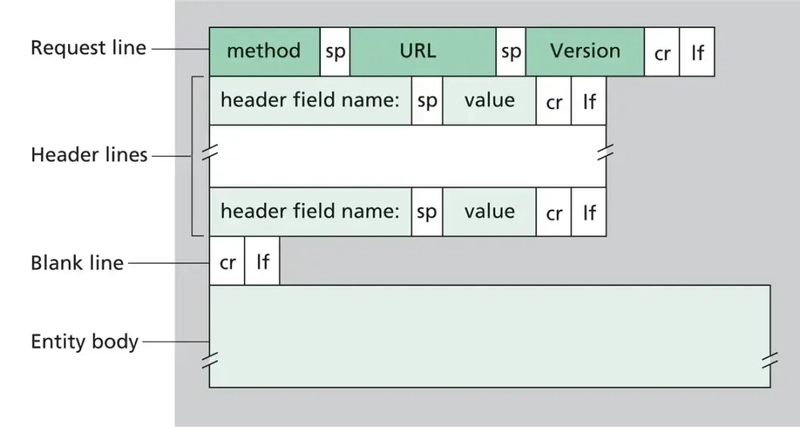
Introduction
This is Part 1 of a multi-part series where we will build a complete HTTP web server from scratch.
In this series of articles, I want to show how to create an HTTP web server from scratch.
The goal isn’t to build a production-ready system — it’s to explore different approaches to implementing a server: starting from a simple, naive, single-threaded server and moving toward asynchronous, non-blocking designs similar to those used by modern high-performance servers like Nginx.
At each step, I’ll measure performance and share the results with you.
The main motivation behind this article is to take a deep look under the hood of how web servers work and to truly understand the fundamental concepts involved.
When I started writing, I had two goals in mind:
- Understand it thoroughly myself.
- Explain it clearly to you, step-by-step.
However, as I dove deeper, I realized that building a server also touches on many other foundational topics — like sockets, the HTTP protocol, and concurrency.
This led me down a bit of a rabbit hole! I had to make some choices about how deep to go into each topic without overwhelming you or oversimplifying important concepts.
Because of this, I’ve structured the article into two main parts:
- Theory — covering everything we need to know before we start coding.
- Implementation — where we’ll build different versions of our server.
Feel free to skim the theory if you’re already familiar with some topics, but I highly recommend at least glancing through it, as I’ll refer back to key concepts throughout the implementation stages.
In theory section we will learn:
- What a web server is and what it does
- How HTTP defines the rules of communication
- Why sockets are essential for network connections
- The structure of an HTTP message
- How persistent connections impact parsing
- What functionality we need to implement in our server