Building LitFlip: An Immersive Book Reading Experience
Learn how we built an immersive digital reading platform using React, Three.js, and Next.js Building LitFlip: A 3D Book Reading Experience with React Three Fiber Have you ever wished digital books could feel more like physical ones? That's exactly what we set out to achieve with LitFlip - a web application that brings the tactile joy of reading physical books to the digital world. The Vision LitFlip transforms the way people read digital books by providing an immersive, real-life reading experience. Using modern web technologies, we've created a platform that lets readers: Experience books in a realistic 3D interface Customize their reading environment Track reading progress with detailed analytics Enjoy audio companions while reading Technical Stack Our tech stack was carefully chosen to deliver a smooth, performant experience: Next.js for the frontend framework React Three Fiber for 3D rendering Tailwind CSS for styling Radix UI for accessible components The 3D Book Experience One of our core features is the realistic 3D book rendering. Here's a simplified version of how we implemented it: import { useRef } from 'react' import { useFrame } from '@react-three/fiber' import { Mesh, Group } from 'three' export function Book() { const groupRef = useRef(null) useFrame((state) => { if (groupRef.current) { // Gentle animation groupRef.current.rotation.y = Math.sin(state.clock.elapsedTime * 0.5) * 0.2 } }) return ( {/* Book pages */} {[...Array(15)].map((_, index) => ( ))} {/* Cover */} ) }
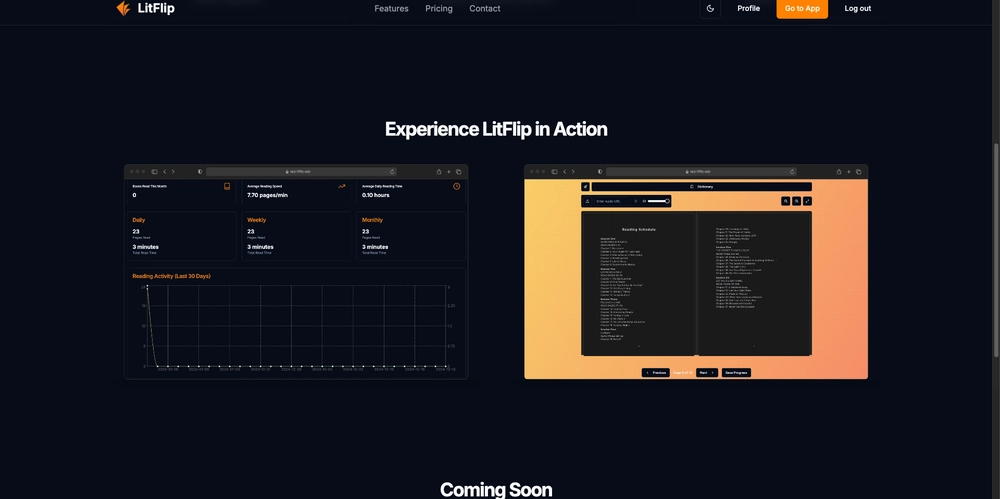
Learn how we built an immersive digital reading platform using React, Three.js, and Next.js
Building LitFlip: A 3D Book Reading Experience with React Three Fiber
Have you ever wished digital books could feel more like physical ones? That's exactly what we set out to achieve with LitFlip - a web application that brings the tactile joy of reading physical books to the digital world.
The Vision
LitFlip transforms the way people read digital books by providing an immersive, real-life reading experience. Using modern web technologies, we've created a platform that lets readers:
- Experience books in a realistic 3D interface
- Customize their reading environment
- Track reading progress with detailed analytics
- Enjoy audio companions while reading
Technical Stack
Our tech stack was carefully chosen to deliver a smooth, performant experience:
- Next.js for the frontend framework
- React Three Fiber for 3D rendering
- Tailwind CSS for styling
- Radix UI for accessible components
The 3D Book Experience
One of our core features is the realistic 3D book rendering. Here's a simplified version of how we implemented it:
import { useRef } from 'react'
import { useFrame } from '@react-three/fiber'
import { Mesh, Group } from 'three'
export function Book() {
const groupRef = useRef<Group>(null)
useFrame((state) => {
if (groupRef.current) {
// Gentle animation
groupRef.current.rotation.y = Math.sin(state.clock.elapsedTime * 0.5) * 0.2
}
})
return (
<group ref={groupRef}>
{/* Book pages */}
{[...Array(15)].map((_, index) => (
<mesh key={index} position={[0, 0, -0.09 + index * 0.01]}>
<boxGeometry args={[1.95, 2.95, 0.001]} />
<meshStandardMaterial color="#f8fafc" />
mesh>
))}
{/* Cover */}
<mesh position={[0, 0, 0.1]}>
<boxGeometry args={[2, 3, 0.02]} />
<meshStandardMaterial color="#1e293b" />
mesh>
group>
)
}