After a Year of Wanting a Typed Rails and Starting Development, I Released a Framework for TypeScript
I released version 1.0 of Accella, a TypeScript-oriented web framework, in February 2025. In this article, I’ll provide a brief introduction to Accella, followed by a chronological overview of its development process and the design intentions behind it. What is Accella? Accella is a server-side web application framework designed for TypeScript. Below is an excerpt of its features from the opening section of the official website (https://accella.dev): Server-First Accella follows the tradition of full-stack MVC frameworks. Based on Astro, it returns pre-rendered HTML to the client from the server. By keeping the architecture simple, it enhances both development efficiency and user experience. ORM Integration Accella utilizes Accel Record, an ORM implemented with the Active Record pattern. This integration enhances development efficiency, particularly for database CRUD operations. Type Safety Accella provides a type-safe development environment with TypeScript, covering everything from table operations to template rendering. For more details about the framework, check out the official website. It includes sample code for application development and comparisons with other frameworks. Design Intentions and Development Journey I’ll outline the journey from the start of Accella’s development to its release, focusing particularly on aspects related to its design intentions. Starting with an ORM Library Development of Accella began in January 2024. At the time, I wanted to contribute to open-source software (OSS) while also thinking, “I wish TypeScript had a framework as efficient as Ruby on Rails.” The elements I sought in this “Rails-like development efficiency” included: Development based on SSR (Server-Side Rendering) for MPA (Multi-Page Applications), not SPA (Single-Page Applications). The ability to implement RDB (Relational Database) CRUD functionality with minimal code. A framework covering the MVC (Model-View-Controller) domains. After researching existing frameworks, I couldn’t find one that sufficiently met these criteria. Further analysis led me to conclude that a powerful ORM like Rails’ Active Record was necessary, so I started the project by developing an ORM for TypeScript. I wrote about the motivations behind this in more detail in the following article: Seeking a Type-Safe Ruby on Rails in TypeScript, I Started Developing an ORM Using Prisma for Table Definitions and Migrations While developing the ORM, I considered how to handle table definitions and migrations. I discovered that Prisma’s generator feature allows arbitrary code generation from Prisma’s table definition files. Even query builder libraries like Kysely can use Prisma generators to output necessary type definition files. For this ORM, I decided to use Prisma for table definitions and migrations, automatically generating required files with Prisma’s generator. While Rails’ migrations involve creating differential files, I personally find Prisma’s approach—automatically generating migration files from schema files—more convenient, which was one reason for this choice. Adopting a Synchronous API for the ORM Typically, JavaScript/TypeScript libraries use asynchronous APIs for external access like database operations. However, as development progressed, I began to think that designing the ORM’s interface as a synchronous API would be better. With asynchronous APIs that return Promises, the common approach is to use await to wait for completion: const user = await User.first(); // Fetch the first User from the database I found that asynchronous APIs limited the library’s interface, making it difficult to achieve the usability of Rails’ Active Record. So, I adopted a synchronous API that allows calling operations without await. This was the most challenging part of the ORM’s design and implementation, but it’s also where its uniqueness shines. I’ve written more about synchronous APIs in the following articles, so feel free to check them out if you’re interested: Why We Adopted a Synchronous API for the New TypeScript ORM Even Server-Side TypeScript Needs the Option to Avoid Asynchronous Processing Techniques for Synchronous DB Access in TypeScript Released a Library for Synchronous Execution of Asynchronous Processes in JS/TS Releasing the ORM as Accel Record In April 2024, I released version 1.0 of the ORM library “Accel Record.” It implements core CRUD operations for tables, featuring a synchronous API and type safety, such as changing model types before and after persistence. Introduction to "Accel Record": A TypeScript ORM Using the Active Record Pattern Afterward, I added more features to the ORM, heavily inspired by Rails’ Active Record: Validation Factories Bulk Inserts Serialization Transactions Internationalization (I18n) Callbacks PostgreSQL Support Password Authentication Scopes Advanced Search Locking Composite
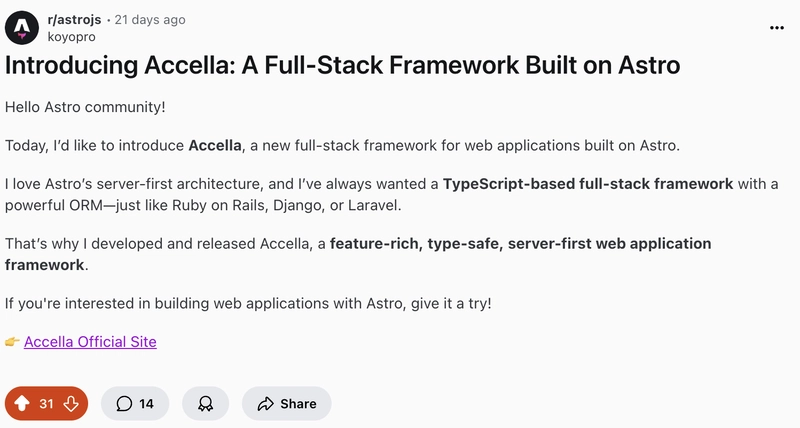
I released version 1.0 of Accella, a TypeScript-oriented web framework, in February 2025. In this article, I’ll provide a brief introduction to Accella, followed by a chronological overview of its development process and the design intentions behind it.
What is Accella?
Accella is a server-side web application framework designed for TypeScript. Below is an excerpt of its features from the opening section of the official website (https://accella.dev):
- Server-First
- Accella follows the tradition of full-stack MVC frameworks. Based on Astro, it returns pre-rendered HTML to the client from the server. By keeping the architecture simple, it enhances both development efficiency and user experience.
- ORM Integration
- Accella utilizes Accel Record, an ORM implemented with the Active Record pattern. This integration enhances development efficiency, particularly for database CRUD operations.
- Type Safety
- Accella provides a type-safe development environment with TypeScript, covering everything from table operations to template rendering.
For more details about the framework, check out the official website. It includes sample code for application development and comparisons with other frameworks.
Design Intentions and Development Journey
I’ll outline the journey from the start of Accella’s development to its release, focusing particularly on aspects related to its design intentions.
Starting with an ORM Library
Development of Accella began in January 2024. At the time, I wanted to contribute to open-source software (OSS) while also thinking, “I wish TypeScript had a framework as efficient as Ruby on Rails.”
The elements I sought in this “Rails-like development efficiency” included:
- Development based on SSR (Server-Side Rendering) for MPA (Multi-Page Applications), not SPA (Single-Page Applications).
- The ability to implement RDB (Relational Database) CRUD functionality with minimal code.
- A framework covering the MVC (Model-View-Controller) domains.
After researching existing frameworks, I couldn’t find one that sufficiently met these criteria. Further analysis led me to conclude that a powerful ORM like Rails’ Active Record was necessary, so I started the project by developing an ORM for TypeScript.
I wrote about the motivations behind this in more detail in the following article:
Seeking a Type-Safe Ruby on Rails in TypeScript, I Started Developing an ORM
Using Prisma for Table Definitions and Migrations
While developing the ORM, I considered how to handle table definitions and migrations.
I discovered that Prisma’s generator feature allows arbitrary code generation from Prisma’s table definition files. Even query builder libraries like Kysely can use Prisma generators to output necessary type definition files. For this ORM, I decided to use Prisma for table definitions and migrations, automatically generating required files with Prisma’s generator. While Rails’ migrations involve creating differential files, I personally find Prisma’s approach—automatically generating migration files from schema files—more convenient, which was one reason for this choice.
Adopting a Synchronous API for the ORM
Typically, JavaScript/TypeScript libraries use asynchronous APIs for external access like database operations. However, as development progressed, I began to think that designing the ORM’s interface as a synchronous API would be better.
With asynchronous APIs that return Promises, the common approach is to use await
to wait for completion:
const user = await User.first(); // Fetch the first User from the database
I found that asynchronous APIs limited the library’s interface, making it difficult to achieve the usability of Rails’ Active Record. So, I adopted a synchronous API that allows calling operations without await
. This was the most challenging part of the ORM’s design and implementation, but it’s also where its uniqueness shines.
I’ve written more about synchronous APIs in the following articles, so feel free to check them out if you’re interested:
- Why We Adopted a Synchronous API for the New TypeScript ORM
- Even Server-Side TypeScript Needs the Option to Avoid Asynchronous Processing
- Techniques for Synchronous DB Access in TypeScript
- Released a Library for Synchronous Execution of Asynchronous Processes in JS/TS
Releasing the ORM as Accel Record
In April 2024, I released version 1.0 of the ORM library “Accel Record.” It implements core CRUD operations for tables, featuring a synchronous API and type safety, such as changing model types before and after persistence.
Introduction to "Accel Record": A TypeScript ORM Using the Active Record Pattern
Afterward, I added more features to the ORM, heavily inspired by Rails’ Active Record:
- Validation
- Factories
- Bulk Inserts
- Serialization
- Transactions
- Internationalization (I18n)
- Callbacks
- PostgreSQL Support
- Password Authentication
- Scopes
- Advanced Search
- Locking
- Composite Key Support
- Form Objects
Using Astro Components for Rendering
In Rails, pagination and forms can be easily created based on Active Record data. I wanted to achieve similar functionality in Accella. I also wanted the View templates (like erb in Rails) to be type-safe. Traditional server-side JS template engines (EJS, Pug, etc.) didn’t seem capable of this. That’s when Astro came up as a candidate. I found that Astro components allow type-safe server-side template rendering, similar to React or Vue.
By August 2024, the ORM’s features were fairly robust, so I began implementing functionalities such as form building integrated with the ORM using Astro components:
- Pagination
- Forms
- Request Parameter Parsing
- Session Management
- CSRF Protection
Contributing to Astro
To use Astro components as a template engine, Astro needed to serve as Accella’s base. In MVC terms, the Model is handled by Accella’s custom ORM, while the View and Controller are managed by Astro’s features.
While developing features, I frequently worked with Astro and contributed to its community by addressing issues I encountered.
In Rails, session data is stored in cookies by default. I created a library for Astro to store sessions in cookies and shared it on Reddit, receiving a lot of positive feedback:
I just published "Astro Cookie Session", middleware for managing sessions using cookies on Astro. : r/astrojs
I also submitted two bug-fix pull requests to Astro’s main repository, which were merged.
Polishing it as a Framework
Around November 2024, I worked on refining the features I’d built into a usable framework. Like Rails’ rails new
, I aimed for an environment where application development could start immediately with npm create
.
I ensured that SQLite could be used for development right after setup and that tests involving table read/write operations could run, focusing on completing database-related setup. Accella uses Vitest as its testing framework, but since Vitest runs tests in multiple processes (or threads), I had to devise ways to properly prepare multiple test databases.
Ultimately, running npm create accella
sets up an environment where database-driven development can begin immediately.
Documentation and Version 1.0 Release
Starting in January 2025, I prepared Accella’s documentation site, creating a starter guide while fixing usability issues and bugs. I built the documentation with Astro Starlight and hosted it on Netlify, which made the process quite smooth.
On February 26, 2025, I released version 1.0 of Accella.
I posted about it on Reddit and received a lot of responses:
Introducing Accella: A Full-Stack Framework Built on Astro : r/astrojs
Future Development
With version 1.0 released and documentation prepared, Accella is now in a state where others can try it out. I believe I’ve created one direction for my initial motivation: “I want a TypeScript framework as efficient as Ruby on Rails.” In particular, the ORM featuring the “Active Record pattern” and “synchronous API” could offer a new option for server-side JavaScript.
Currently, I’m developing a CarrierWave-like library that integrates with ORM models to easily upload files to S3.
In the future, I’d like to work on:
- A library for efficiently managing initial (seed) database data (like Seed Fu).
- A library for easily creating internal admin panels (like Active Admin).
If you’re interested, I’d be delighted if you’d star the repository:
https://github.com/koyopro/accella
Thank you for reading to the end!