A Deep Dive into FormData and sendBeacon
In the previous blog, we discussed the fundamentals of form elements in JavaScript. Now, let's dive deeper into how data is transferred between the client side and the server, and explore how to enhance features for better analysis. JavaScript can send network requests to a server to fetch information, post new data, update existing data, or delete data. There are several ways to achieve this, but Promise-based methods like fetch() and .then() are commonly used. When sending data to the server in the request body, the data can be formatted as a string, a Blob (binary data), or FormData. FormData The FormData object makes it easier to send HTML form data to a server. By using the header Content-Type: multipart/form-data, the server can process it as a form submission. Constructor let formData = new FormData([form]); When an HTML form is passed as an argument, the FormData object automatically gathers its fields. Example: formElem.onsubmit = async (e) => { e.preventDefault(); let response = await fetch('/article/formdata/post/user', { method: 'POST', body: new FormData(formElem) }); let result = await response.json(); alert(result.message); }; The Modern JavaScript Tutorial Modifying Fields with FormData The FormData object provides several methods for managing its fields: formData.append(name, value) formData.append(name, blob, fileName) The append() method appends a new value to the existing key or adds the key if it doesn't exist. formData.set(name, value) formData.set(name, blob, fileName) The set() method removes fields with the same name and sets a new value for an existing key or adds the key-value pair if the key doesn't exist. formData.delete(name) The delete() method deletes the key-value pair in the FormData object. formData.get(name) The get() method returns the first value associated with a key. formData.getAll(name) The getAll() method returns an array of all values associated with a key. formData.has(name) The has() method returns a boolean indicating whether the FormData object contains the key. formData.keys() The keys() method returns an iterator for all keys in the FormData object. formData.values() The values() method returns an iterator for all values in the FormData object. formData.entries() The entries() method returns an iterator for all key-value pairs in the FormData object. The difference between append and set is that if there is a field with the same name, set will replace the existing data, while append doesn't. Sending Other Types of Data with FormData You may notice the method formData.set(name, blob, fileName) --- interestingly, FromData can send not only form data but also files and blobs. File Picture: formElem.onsubmit = async (e) => { e.preventDefault(); let response = await fetch('/article/formdata/post/user-avatar', { method: 'POST', body: new FormData(formElem) }); let result = await response.json(); alert(result.message); }; Blob canvasElem.onmousemove = function(e) { let ctx = canvasElem.getContext('2d'); ctx.lineTo(e.clientX, e.clientY); ctx.stroke(); }; async function submit() { let imageBlob = await new Promise(resolve => canvasElem.toBlob(resolve, 'image/png')); let formData = new FormData(); formData.append("firstName", "John"); formData.append("image", imageBlob, "image.png"); let response = await fetch('/article/formdata/post/image-form', { method: 'POST', body: formData }); let result = await response.json(); alert(result.message); } sendBeacon The sendBeacon() method is used to transmit data to a server asynchronously. It takes two parameters: the data to be sent and the target URL. This method is designed for sending small amounts of data, typically for analytics or diagnostics, without delaying page unloading events. If the user agent (e.g., a browser or bot) successfully queued the data for transfer, the method returns true. Syntax sendBeacon(url) sendBeacon(url, data) MDN Forms are a vital part of web pages, as they serve as a primary point of interaction with users. There's still much more to explore about forms, including topics like validation and accessibility, which I'll cover in future posts.
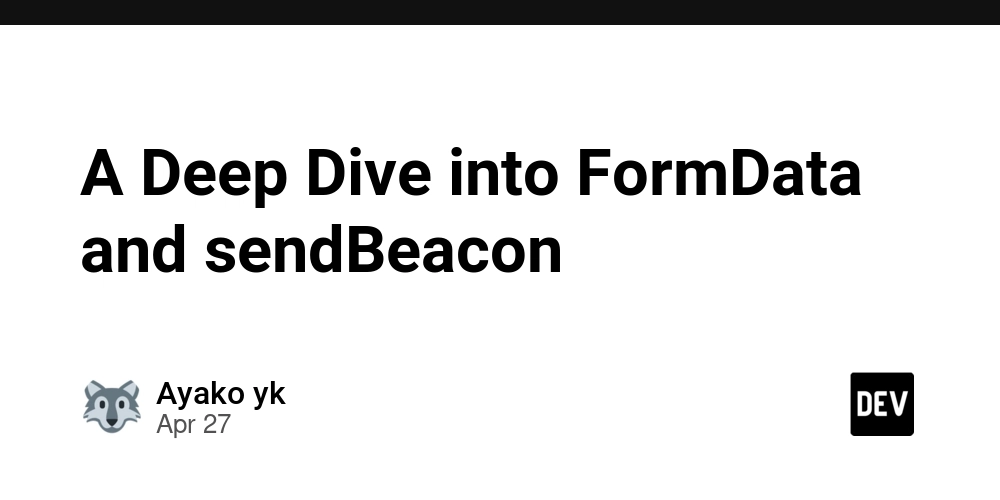
In the previous blog, we discussed the fundamentals of form elements in JavaScript. Now, let's dive deeper into how data is transferred between the client side and the server, and explore how to enhance features for better analysis.
JavaScript can send network requests to a server to fetch information, post new data, update existing data, or delete data. There are several ways to achieve this, but Promise-based methods like fetch()
and .then()
are commonly used.
When sending data to the server in the request body, the data can be formatted as a string, a Blob (binary data), or FormData.
FormData
The FormData
object makes it easier to send HTML form data to a server. By using the header Content-Type: multipart/form-data
, the server can process it as a form submission.
Constructor
let formData = new FormData([form]);
When an HTML form is passed as an argument, the FormData
object automatically gathers its fields.
Example:
The Modern JavaScript Tutorial
Modifying Fields with FormData
The FormData
object provides several methods for managing its fields:
formData.append(name, value)
formData.append(name, blob, fileName)
The append()
method appends a new value to the existing key or adds the key if it doesn't exist.
formData.set(name, value)
formData.set(name, blob, fileName)
The set()
method removes fields with the same name and sets a new value for an existing key or adds the key-value pair if the key doesn't exist.
formData.delete(name)
The delete()
method deletes the key-value pair in the FormData object.
formData.get(name)
The get()
method returns the first value associated with a key.
formData.getAll(name)
The getAll()
method returns an array of all values associated with a key.
formData.has(name)
The has()
method returns a boolean indicating whether the FormData object contains the key.
formData.keys()
The keys()
method returns an iterator for all keys in the FormData object.
formData.values()
The values()
method returns an iterator for all values in the FormData object.
formData.entries()
The entries()
method returns an iterator for all key-value pairs in the FormData object.
The difference between append
and set
is that if there is a field with the same name, set
will replace the existing data, while append
doesn't.
Sending Other Types of Data with FormData
You may notice the method formData.set(name, blob, fileName)
--- interestingly, FromData
can send not only form data but also files and blobs.
File
Blob
sendBeacon
The sendBeacon()
method is used to transmit data to a server asynchronously. It takes two parameters: the data to be sent and the target URL. This method is designed for sending small amounts of data, typically for analytics or diagnostics, without delaying page unloading events. If the user agent (e.g., a browser or bot) successfully queued the data for transfer, the method returns true.
Syntax
sendBeacon(url)
sendBeacon(url, data)
Forms are a vital part of web pages, as they serve as a primary point of interaction with users. There's still much more to explore about forms, including topics like validation and accessibility, which I'll cover in future posts.