6 Essential Service Worker Techniques for Offline-Capable Progressive Web Apps
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world! Service workers represent one of the most powerful tools in modern web development, enabling progressive web applications with offline capabilities, push notifications, and background synchronization. I've spent years implementing service workers across various projects and have discovered that mastering these six techniques dramatically improves their effectiveness. Service Worker Lifecycle Management Proper lifecycle management forms the foundation of any robust service worker implementation. The lifecycle consists of registration, installation, activation, and update phases—each requiring careful handling. Registration should occur after the page has loaded to prevent competition for resources: if ('serviceWorker' in navigator) { window.addEventListener('load', () => { navigator.serviceWorker.register('/sw.js') .then(registration => { console.log('Service Worker registered with scope:', registration.scope); }) .catch(error => { console.error('Service Worker registration failed:', error); }); }); } The installation phase is critical for caching essential assets. I always design this stage to be as quick as possible: const CACHE_NAME = 'app-shell-v1'; const ASSETS = [ '/', '/index.html', '/styles.css', '/app.js', '/offline.html' ]; self.addEventListener('install', event => { event.waitUntil( caches.open(CACHE_NAME) .then(cache => cache.addAll(ASSETS)) .then(() => self.skipWaiting()) ); }); The activation phase provides an opportunity to clean up outdated caches: self.addEventListener('activate', event => { event.waitUntil( caches.keys() .then(cacheNames => { return Promise.all( cacheNames .filter(name => name !== CACHE_NAME) .map(name => caches.delete(name)) ); }) .then(() => self.clients.claim()) ); }); I've learned that using skipWaiting() and clients.claim() ensures the new service worker takes control immediately, but this approach requires careful consideration of cache compatibility between versions. Cache Strategies Implementation Different resources benefit from different caching strategies. I've found that implementing a strategy factory pattern allows for flexible, resource-specific caching: // Strategy factory const strategies = { cacheFirst: async (request) => { const cachedResponse = await caches.match(request); return cachedResponse || fetch(request).then(response => { const responseClone = response.clone(); caches.open(CACHE_NAME).then(cache => { cache.put(request, responseClone); }); return response; }); }, networkFirst: async (request) => { try { const networkResponse = await fetch(request); const responseClone = networkResponse.clone(); caches.open(CACHE_NAME).then(cache => { cache.put(request, responseClone); }); return networkResponse; } catch (error) { const cachedResponse = await caches.match(request); return cachedResponse || caches.match('/offline.html'); } }, staleWhileRevalidate: async (request) => { const cachedResponse = await caches.match(request); const fetchPromise = fetch(request).then(response => { const responseClone = response.clone(); caches.open(CACHE_NAME).then(cache => { cache.put(request, responseClone); }); return response; }); return cachedResponse || fetchPromise; } }; In my fetch event handler, I apply these strategies based on URL patterns: self.addEventListener('fetch', event => { const url = new URL(event.request.url); // Apply strategies based on resource type if (event.request.mode === 'navigate') { event.respondWith(strategies.networkFirst(event.request)); } else if (url.pathname.startsWith('/api/')) { event.respondWith(strategies.networkFirst(event.request)); } else if (url.pathname.startsWith('/images/')) { event.respondWith(strategies.staleWhileRevalidate(event.request)); } else { event.respondWith(strategies.cacheFirst(event.request)); } }); This approach has significantly improved performance in my applications, particularly for content that benefits from immediate display while updating in the background. Navigation Preload On several projects, I've noticed that service worker startup can delay the initial network request. Navigation preload solves this by allowing the browser to start the request before the service worker initializes. I enable it during the activation phase: self.addEventListener('activate', event => { event.waitUntil( Promise.all([ self.clients.claim(), // Enable navigation preload if supported self.registr
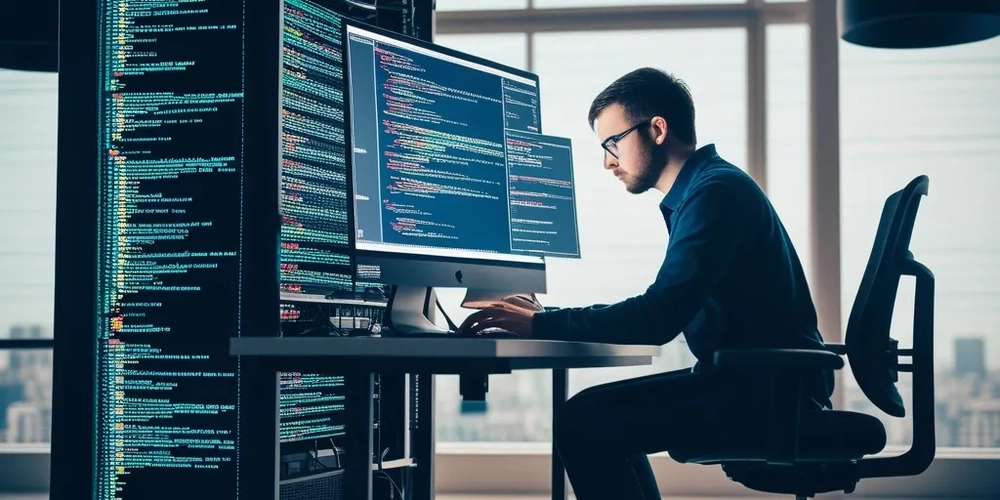
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Service workers represent one of the most powerful tools in modern web development, enabling progressive web applications with offline capabilities, push notifications, and background synchronization. I've spent years implementing service workers across various projects and have discovered that mastering these six techniques dramatically improves their effectiveness.
Service Worker Lifecycle Management
Proper lifecycle management forms the foundation of any robust service worker implementation. The lifecycle consists of registration, installation, activation, and update phases—each requiring careful handling.
Registration should occur after the page has loaded to prevent competition for resources:
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/sw.js')
.then(registration => {
console.log('Service Worker registered with scope:', registration.scope);
})
.catch(error => {
console.error('Service Worker registration failed:', error);
});
});
}
The installation phase is critical for caching essential assets. I always design this stage to be as quick as possible:
const CACHE_NAME = 'app-shell-v1';
const ASSETS = [
'/',
'/index.html',
'/styles.css',
'/app.js',
'/offline.html'
];
self.addEventListener('install', event => {
event.waitUntil(
caches.open(CACHE_NAME)
.then(cache => cache.addAll(ASSETS))
.then(() => self.skipWaiting())
);
});
The activation phase provides an opportunity to clean up outdated caches:
self.addEventListener('activate', event => {
event.waitUntil(
caches.keys()
.then(cacheNames => {
return Promise.all(
cacheNames
.filter(name => name !== CACHE_NAME)
.map(name => caches.delete(name))
);
})
.then(() => self.clients.claim())
);
});
I've learned that using skipWaiting()
and clients.claim()
ensures the new service worker takes control immediately, but this approach requires careful consideration of cache compatibility between versions.
Cache Strategies Implementation
Different resources benefit from different caching strategies. I've found that implementing a strategy factory pattern allows for flexible, resource-specific caching:
// Strategy factory
const strategies = {
cacheFirst: async (request) => {
const cachedResponse = await caches.match(request);
return cachedResponse || fetch(request).then(response => {
const responseClone = response.clone();
caches.open(CACHE_NAME).then(cache => {
cache.put(request, responseClone);
});
return response;
});
},
networkFirst: async (request) => {
try {
const networkResponse = await fetch(request);
const responseClone = networkResponse.clone();
caches.open(CACHE_NAME).then(cache => {
cache.put(request, responseClone);
});
return networkResponse;
} catch (error) {
const cachedResponse = await caches.match(request);
return cachedResponse || caches.match('/offline.html');
}
},
staleWhileRevalidate: async (request) => {
const cachedResponse = await caches.match(request);
const fetchPromise = fetch(request).then(response => {
const responseClone = response.clone();
caches.open(CACHE_NAME).then(cache => {
cache.put(request, responseClone);
});
return response;
});
return cachedResponse || fetchPromise;
}
};
In my fetch event handler, I apply these strategies based on URL patterns:
self.addEventListener('fetch', event => {
const url = new URL(event.request.url);
// Apply strategies based on resource type
if (event.request.mode === 'navigate') {
event.respondWith(strategies.networkFirst(event.request));
} else if (url.pathname.startsWith('/api/')) {
event.respondWith(strategies.networkFirst(event.request));
} else if (url.pathname.startsWith('/images/')) {
event.respondWith(strategies.staleWhileRevalidate(event.request));
} else {
event.respondWith(strategies.cacheFirst(event.request));
}
});
This approach has significantly improved performance in my applications, particularly for content that benefits from immediate display while updating in the background.
Navigation Preload
On several projects, I've noticed that service worker startup can delay the initial network request. Navigation preload solves this by allowing the browser to start the request before the service worker initializes.
I enable it during the activation phase:
self.addEventListener('activate', event => {
event.waitUntil(
Promise.all([
self.clients.claim(),
// Enable navigation preload if supported
self.registration.navigationPreload &&
self.registration.navigationPreload.enable()
])
);
});
Then I leverage the preloaded response in my fetch handler:
self.addEventListener('fetch', event => {
if (event.request.mode === 'navigate') {
event.respondWith(async function() {
try {
// Try to use the preloaded response
const preloadResponse = await event.preloadResponse;
if (preloadResponse) {
return preloadResponse;
}
// Otherwise, use the network
const networkResponse = await fetch(event.request);
return networkResponse;
} catch (error) {
// If both fail, show cached offline page
const cache = await caches.open(CACHE_NAME);
const cachedResponse = await cache.match('/offline.html');
return cachedResponse;
}
}());
}
});
This technique has reduced my application's time-to-content by hundreds of milliseconds, particularly on slower devices.
Background Sync
For forms and data submission in unstable network environments, background sync has proven invaluable. I implement a queue system that stores failed requests and retries them when connectivity returns:
// In the service worker
self.addEventListener('sync', event => {
if (event.tag === 'outbox') {
event.waitUntil(processOutbox());
}
});
async function processOutbox() {
const db = await openDatabase();
const outbox = db.transaction('outbox').objectStore('outbox');
const requests = await getAll(outbox);
return Promise.all(requests.map(async record => {
try {
await fetch(record.url, {
method: record.method,
headers: record.headers,
body: record.body
});
// If successful, remove from outbox
const transaction = db.transaction('outbox', 'readwrite');
transaction.objectStore('outbox').delete(record.id);
return transaction.complete;
} catch (error) {
// Keep in outbox to retry later
console.error('Sync failed:', error);
return Promise.reject(error);
}
}));
}
// Helper functions for IndexedDB interactions
function openDatabase() {
return new Promise((resolve, reject) => {
const request = indexedDB.open('OfflineData', 1);
request.onupgradeneeded = event => {
const db = event.target.result;
if (!db.objectStoreNames.contains('outbox')) {
db.createObjectStore('outbox', { keyPath: 'id', autoIncrement: true });
}
};
request.onsuccess = event => resolve(event.target.result);
request.onerror = event => reject(event.target.error);
});
}
function getAll(objectStore) {
return new Promise((resolve, reject) => {
const request = objectStore.getAll();
request.onsuccess = event => resolve(event.target.result);
request.onerror = event => reject(event.target.error);
});
}
On the client side, I queue failed requests and register for sync:
async function sendData(url, method, data) {
if (!navigator.onLine) {
await saveToOutbox(url, method, data);
await registerSync();
return { offline: true, message: 'Request queued for sync' };
}
try {
const response = await fetch(url, {
method,
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(data)
});
return await response.json();
} catch (error) {
await saveToOutbox(url, method, data);
await registerSync();
return { offline: true, message: 'Request queued for sync' };
}
}
async function saveToOutbox(url, method, data) {
const db = await openDatabase();
const transaction = db.transaction('outbox', 'readwrite');
transaction.objectStore('outbox').add({
url,
method,
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(data),
timestamp: Date.now()
});
return transaction.complete;
}
async function registerSync() {
if ('serviceWorker' in navigator && 'SyncManager' in window) {
const registration = await navigator.serviceWorker.ready;
try {
await registration.sync.register('outbox');
} catch (error) {
console.error('Sync registration failed:', error);
}
}
}
This pattern has significantly improved user experience in my applications, especially for mobile users with spotty connections.
Push Notification Architecture
I've found that push notifications require careful planning for both technical implementation and user experience. My approach starts with proper permission handling:
async function requestNotificationPermission() {
if (!('Notification' in window)) {
console.log('This browser does not support notifications');
return false;
}
if (Notification.permission === 'granted') {
return true;
}
if (Notification.permission !== 'denied') {
const permission = await Notification.requestPermission();
return permission === 'granted';
}
return false;
}
For subscription management:
async function subscribeUserToPush() {
const registration = await navigator.serviceWorker.ready;
const subscribeOptions = {
userVisibleOnly: true,
applicationServerKey: urlBase64ToUint8Array(
'BKtLD...public_key...YnJx'
)
};
const subscription = await registration.pushManager.subscribe(subscribeOptions);
// Send subscription to server
await fetch('/api/push-subscriptions', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(subscription)
});
return subscription;
}
// Helper to convert base64 string to Uint8Array
function urlBase64ToUint8Array(base64String) {
const padding = '='.repeat((4 - base64String.length % 4) % 4);
const base64 = (base64String + padding)
.replace(/-/g, '+')
.replace(/_/g, '/');
const rawData = window.atob(base64);
const outputArray = new Uint8Array(rawData.length);
for (let i = 0; i < rawData.length; ++i) {
outputArray[i] = rawData.charCodeAt(i);
}
return outputArray;
}
In the service worker, I handle incoming push events and create rich notifications:
self.addEventListener('push', event => {
if (!event.data) return;
const data = event.data.json();
const options = {
body: data.message,
icon: '/images/notification-icon.png',
badge: '/images/notification-badge.png',
vibrate: [100, 50, 100],
data: {
url: data.url,
actionId: data.actionId
},
actions: [
{
action: 'view',
title: 'View'
},
{
action: 'dismiss',
title: 'Dismiss'
}
]
};
event.waitUntil(
self.registration.showNotification(data.title, options)
);
});
self.addEventListener('notificationclick', event => {
event.notification.close();
if (event.action === 'dismiss') return;
const urlToOpen = event.notification.data.url || '/';
event.waitUntil(
clients.matchAll({ type: 'window' })
.then(clientList => {
for (const client of clientList) {
if (client.url === urlToOpen && 'focus' in client) {
return client.focus();
}
}
if (clients.openWindow) {
return clients.openWindow(urlToOpen);
}
})
);
});
This approach creates a seamless notification experience while giving users control over their interaction with the notifications.
Versioned Caching
Managing cache updates can be challenging. I've adopted a versioned caching system that prevents stale content and provides clean transitions between application versions:
const CACHE_VERSION = '2023-10-15v1';
const STATIC_CACHE = `static-${CACHE_VERSION}`;
const DYNAMIC_CACHE = `dynamic-${CACHE_VERSION}`;
const API_CACHE = `api-${CACHE_VERSION}`;
const CACHE_CONFIGS = [
{
name: STATIC_CACHE,
urls: [
'/',
'/index.html',
'/styles.css',
'/app.js',
'/offline.html'
]
},
{
name: API_CACHE,
urls: [] // Will be filled dynamically
}
];
self.addEventListener('install', event => {
event.waitUntil(
Promise.all(
CACHE_CONFIGS.map(config =>
caches.open(config.name)
.then(cache => cache.addAll(config.urls))
)
).then(() => self.skipWaiting())
);
});
self.addEventListener('activate', event => {
event.waitUntil(
caches.keys()
.then(cacheNames => {
return Promise.all(
cacheNames
.filter(name => {
return name.startsWith('static-') && name !== STATIC_CACHE ||
name.startsWith('dynamic-') && name !== DYNAMIC_CACHE ||
name.startsWith('api-') && name !== API_CACHE;
})
.map(name => caches.delete(name))
);
})
.then(() => self.clients.claim())
);
});
For efficient cache management, I also implement cache expiration and size limits:
async function limitCacheSize(cacheName, maxItems) {
const cache = await caches.open(cacheName);
const keys = await cache.keys();
if (keys.length > maxItems) {
// Delete oldest items first
await cache.delete(keys[0]);
// Recursively call until size is under limit
await limitCacheSize(cacheName, maxItems);
}
}
async function deleteExpiredCache(cacheName, maxAgeSeconds) {
const cache = await caches.open(cacheName);
const keys = await cache.keys();
const now = Date.now();
for (const request of keys) {
const response = await cache.match(request);
const responseDate = response.headers.get('date');
if (responseDate) {
const expirationTime = new Date(responseDate).getTime() + (maxAgeSeconds * 1000);
if (now > expirationTime) {
await cache.delete(request);
}
}
}
}
I call these maintenance functions periodically to keep the cache optimized:
self.addEventListener('message', event => {
if (event.data === 'PERFORM_CACHE_MAINTENANCE') {
event.waitUntil(
Promise.all([
limitCacheSize(DYNAMIC_CACHE, 50),
deleteExpiredCache(API_CACHE, 60 * 60 * 24) // 24 hours
])
);
}
});
This versioned approach has eliminated many common caching problems in my applications, particularly during updates.
Service workers have transformed how I build web applications. Through these six techniques—lifecycle management, cache strategies, navigation preload, background sync, push notifications, and versioned caching—I've created applications that work reliably regardless of network conditions. While implementing service workers requires careful planning and testing, the result is a dramatically improved user experience that bridges the gap between native and web applications.
101 Books
101 Books is an AI-driven publishing company co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Our Creations
Be sure to check out our creations:
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva