WebRTC for Peer-to-Peer Communication
WebRTC for Peer-to-Peer Communication: An Exhaustive Guide WebRTC (Web Real-Time Communication) is a technology that enables peer-to-peer communication directly between web browsers, allowing audio, video, and data to be shared without the need for additional plugins or third-party applications. This comprehensive article delves deeply into the intricate workings, historical context, implementation techniques, and advanced use cases of WebRTC, providing a detailed guide that serves as a reference for senior developers. Historical Context WebRTC originated from a project backed by Google, first announced in 2011, with a mission to simplify real-time communication for web applications. The standardization effort quickly gained momentum, and by 2012, the W3C and IETF began working on the specifications that would eventually culminate in a fully functional suite of APIs. By 2017, WebRTC 1.0 was officially recommended as a stable standard. Key Historical Milestones: 2011: Introduction of WebRTC project by Google aiming to facilitate video and voice calling through browsers. 2012: W3C initiates discussions, and the first drafts of the WebRTC specifications are published. 2015: WebRTC 1.0 undergoes further updates, leading to broader support across major web browsers. 2020: The emergence of WebRTC 1.0 as an official recommendation. Technical Context WebRTC encompasses three core APIs which provide functionalities for real-time communication: MediaStream: Facilitates access to audio and video streams sourced from local devices. RTCPeerConnection: Manages the communication and transmission of media streams between peers. RTCDataChannel: Permits bi-directional data transfer between peers. Core Technologies: Signaling: WebRTC does not define signaling protocols, but commonly used methods include WebSockets and HTTP-based solutions. NAT Traversal: Utilizing STUN (Session Traversal Utilities for NAT) and TURN (Traversal Using Relays around NAT) servers for overcoming Network Address Translation (NAT) barriers. Underlying Protocols: Protocol Usage: WebRTC primarily employs UDP (User Datagram Protocol) for low-latency and real-time communication, complemented by protocols like SRTP (Secure Real-time Transport Protocol) for encrypting RTP payload. ICE (Interactive Connectivity Establishment): Assists in establishing peer-to-peer connections by discovering the best path for communication. In-Depth Code Examples To grasp WebRTC's functionality, it is imperative to examine code implementations that encapsulate real-world scenarios. Below is an example of a simplified WebRTC application. Initial Peer Connection Setup // Get references to HTML elements const localVideo = document.getElementById('localVideo'); const remoteVideo = document.getElementById('remoteVideo'); const startButton = document.getElementById('startButton'); const callButton = document.getElementById('callButton'); // Global variables let localStream; let pc; // Function to start local video stream async function startLocalStream() { localStream = await navigator.mediaDevices.getUserMedia({ video: true, audio: true }); localVideo.srcObject = localStream; } // Create Peer Connection function createPeerConnection() { pc = new RTCPeerConnection(); // When local stream is added to peer connection localStream.getTracks().forEach(track => pc.addTrack(track, localStream)); // Event listener for receiving remote stream pc.ontrack = (event) => { remoteVideo.srcObject = event.streams[0]; }; // ICE candidate handling pc.onicecandidate = ({ candidate }) => { if (candidate) { // Send the candidate to peer sendMessage('candidate', candidate); } }; } // Example signaling message function function sendMessage(type, data) { // Placeholder function console.log(`Sending message: ${type}`, data); } Establishing a Connection Establishing a connection requires two steps: creating an offer and setting it on each peer. // Initiate call async function initiateCall() { createPeerConnection(); const offer = await pc.createOffer(); await pc.setLocalDescription(offer); sendMessage('offer', offer); } // Receiving an offer async function receiveOffer(offer) { await pc.setRemoteDescription(new RTCSessionDescription(offer)); const answer = await pc.createAnswer(); await pc.setLocalDescription(answer); sendMessage('answer', answer); } // Handling answers async function receiveAnswer(answer) { await pc.setRemoteDescription(new RTCSessionDescription(answer)); } Advanced Scenarios and Edge Cases In practice, WebRTC applications must handle more sophisticated scenarios and edge cases. Handling Network Changes WebRTC is designed to respond to varying network conditions. Implementing event l
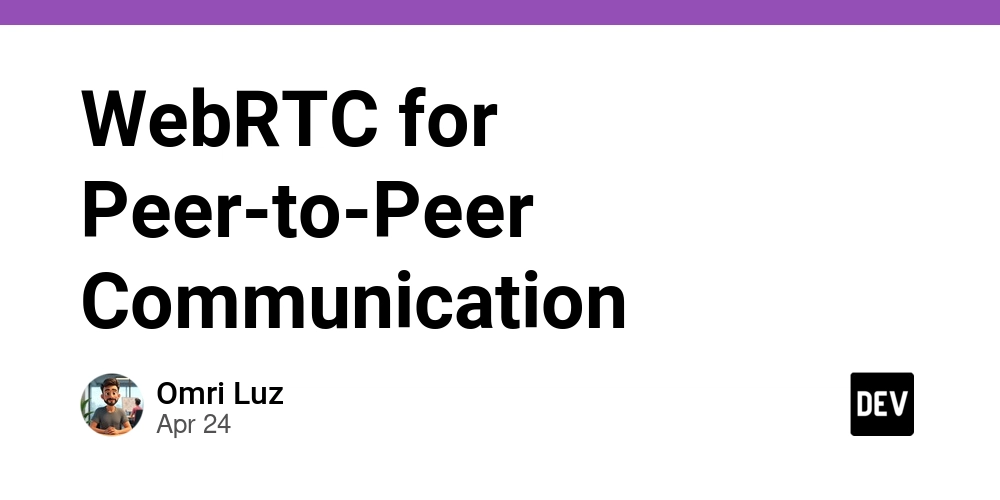
WebRTC for Peer-to-Peer Communication: An Exhaustive Guide
WebRTC (Web Real-Time Communication) is a technology that enables peer-to-peer communication directly between web browsers, allowing audio, video, and data to be shared without the need for additional plugins or third-party applications. This comprehensive article delves deeply into the intricate workings, historical context, implementation techniques, and advanced use cases of WebRTC, providing a detailed guide that serves as a reference for senior developers.
Historical Context
WebRTC originated from a project backed by Google, first announced in 2011, with a mission to simplify real-time communication for web applications. The standardization effort quickly gained momentum, and by 2012, the W3C and IETF began working on the specifications that would eventually culminate in a fully functional suite of APIs. By 2017, WebRTC 1.0 was officially recommended as a stable standard.
Key Historical Milestones:
- 2011: Introduction of WebRTC project by Google aiming to facilitate video and voice calling through browsers.
- 2012: W3C initiates discussions, and the first drafts of the WebRTC specifications are published.
- 2015: WebRTC 1.0 undergoes further updates, leading to broader support across major web browsers.
- 2020: The emergence of WebRTC 1.0 as an official recommendation.
Technical Context
WebRTC encompasses three core APIs which provide functionalities for real-time communication:
- MediaStream: Facilitates access to audio and video streams sourced from local devices.
- RTCPeerConnection: Manages the communication and transmission of media streams between peers.
- RTCDataChannel: Permits bi-directional data transfer between peers.
Core Technologies:
- Signaling: WebRTC does not define signaling protocols, but commonly used methods include WebSockets and HTTP-based solutions.
- NAT Traversal: Utilizing STUN (Session Traversal Utilities for NAT) and TURN (Traversal Using Relays around NAT) servers for overcoming Network Address Translation (NAT) barriers.
Underlying Protocols:
- Protocol Usage: WebRTC primarily employs UDP (User Datagram Protocol) for low-latency and real-time communication, complemented by protocols like SRTP (Secure Real-time Transport Protocol) for encrypting RTP payload.
- ICE (Interactive Connectivity Establishment): Assists in establishing peer-to-peer connections by discovering the best path for communication.
In-Depth Code Examples
To grasp WebRTC's functionality, it is imperative to examine code implementations that encapsulate real-world scenarios. Below is an example of a simplified WebRTC application.
Initial Peer Connection Setup
// Get references to HTML elements
const localVideo = document.getElementById('localVideo');
const remoteVideo = document.getElementById('remoteVideo');
const startButton = document.getElementById('startButton');
const callButton = document.getElementById('callButton');
// Global variables
let localStream;
let pc;
// Function to start local video stream
async function startLocalStream() {
localStream = await navigator.mediaDevices.getUserMedia({ video: true, audio: true });
localVideo.srcObject = localStream;
}
// Create Peer Connection
function createPeerConnection() {
pc = new RTCPeerConnection();
// When local stream is added to peer connection
localStream.getTracks().forEach(track => pc.addTrack(track, localStream));
// Event listener for receiving remote stream
pc.ontrack = (event) => {
remoteVideo.srcObject = event.streams[0];
};
// ICE candidate handling
pc.onicecandidate = ({ candidate }) => {
if (candidate) {
// Send the candidate to peer
sendMessage('candidate', candidate);
}
};
}
// Example signaling message function
function sendMessage(type, data) {
// Placeholder function
console.log(`Sending message: ${type}`, data);
}
Establishing a Connection
Establishing a connection requires two steps: creating an offer and setting it on each peer.
// Initiate call
async function initiateCall() {
createPeerConnection();
const offer = await pc.createOffer();
await pc.setLocalDescription(offer);
sendMessage('offer', offer);
}
// Receiving an offer
async function receiveOffer(offer) {
await pc.setRemoteDescription(new RTCSessionDescription(offer));
const answer = await pc.createAnswer();
await pc.setLocalDescription(answer);
sendMessage('answer', answer);
}
// Handling answers
async function receiveAnswer(answer) {
await pc.setRemoteDescription(new RTCSessionDescription(answer));
}
Advanced Scenarios and Edge Cases
In practice, WebRTC applications must handle more sophisticated scenarios and edge cases.
Handling Network Changes
WebRTC is designed to respond to varying network conditions. Implementing event listeners to manage connection state is essential.
// Connection state changes
pc.onconnectionstatechange = () => {
switch (pc.connectionState) {
case 'connected':
console.log('Peers are connected.');
break;
case 'disconnected':
console.log('Connection lost. Attempting reconnection...');
break;
case 'failed':
console.error('Connection failed, changes needed.');
break;
case 'closed':
console.log('Peer connection closed.');
break;
}
};
Overcoming NAT Traversal Difficulties
When developing peer-to-peer applications, commonly faced issues are NAT traversal and firewall restrictions. This requires careful handling of STUN and TURN servers.
const iceServers = {
iceServers: [
{ urls: 'stun:stun.l.google.com:19302' }, // Google Public STUN Server
{
urls: 'turn:TURN_SERVER_IP',
username: 'USERNAME',
credential: 'CREDENTIAL'
}
],
};
const pc = new RTCPeerConnection(iceServers);
Comparisons with Alternative Approaches
WebSockets vs. WebRTC
While both enable real-time communication, WebSockets operate over TCP, which can introduce latency due to retransmission, while WebRTC leverages UDP, ensuring lower latency and improved performance.
Use Case Comparison:
- WebSocket: Ideal for lightweight, event-driven applications that require low-latency exchanges, such as chat applications.
- WebRTC: Preferable for applications requiring high-quality audio and video, such as video conferencing.
Real-World Use Cases
Industry Application Examples
- Google Meet: Uses WebRTC for real-time video conferencing with low latency and high-quality audio/video.
- Discord: Implements WebRTC for voice communication in gaming.
- Browser-based File Sharing: Utilizes RTCDataChannel for transferring files directly between users without a server.
Performance Considerations and Optimization Strategies
Performance optimization in WebRTC applications is critical for maintaining quality communication.
- Stun/TURN Server Optimization: Choose geographically distributed servers to minimize latency and improve connectivity.
-
Bitrate Management: Dynamically adjust video/audio quality using the
setParameters
method on media tracks to conserve bandwidth. - Adaptive Video Encoding: Use codecs like VP8 or H.264 for encoding video to enhance performance based on network conditions.
Debugging Techniques and Potential Pitfalls
Common Pitfalls
- Firewall Blocking: Ensure TURN servers are utilized correctly to bypass client-side firewalls.
- ICE Candidate Exchange: Properly managing the collection and exchange of ICE candidates is crucial; failure can cause connection issues.
Debugging Tools
- WebRTC Internals: A Chrome tool to visualize the state of RTC connections.
- Network Logs: Enable detailed logs to investigate connection issues or performance bottlenecks.
Example Debugging Output
pc.getStats(null).then(stats => {
stats.forEach(report => {
console.log(`Report: ${report.type}`, report);
});
});
Additional Resources and Documentation
By integrating the in-depth explanations, elaborate coding examples, and a comprehensive overview of performance considerations, this article serves as an essential guide for senior developers looking to harness the full potential of WebRTC for peer-to-peer communications in modern web applications. The ongoing evolution of web standards and technologies makes continuous learning an imperative pursuit in the rapidly changing landscape of real-time web communication.