Understanding JavaScript Promises: A Friendly Guide
Hey there! If you've been diving into JavaScript and stumbled upon promises, you might feel a mix of curiosity and a bit of dread. Don't worry—you're not alone. I once felt overwhelmed by the idea of asynchronous code, but let me share with you how promises can actually make your life a lot easier. So, What's a Promise Anyway? Imagine ordering your favorite pizza. When you call for delivery, you don't just sit on your phone waiting for the pizza guy to show up. Instead, you carry on with your day. A Promise in JavaScript works in the same way: it's an object that represents the future result of an asynchronous task. It might succeed, it might fail, but either way, it’s out there doing its thing while your code keeps running. In technical terms, a promise has three possible states: Pending: The task is still in progress. Fulfilled: The task completed successfully. Rejected: The task failed. Here's a quick example: const promise = new Promise((resolve, reject) => { // Imagine some asynchronous operation here const isSuccess = true; // you can change this to false to see what happens if (isSuccess) { resolve("Pizza delivered on time!"); } else { reject("Pizza delivery failed."); } }); Working With Promises: .then() and .catch() Once you have a promise, you need to handle what happens next. That’s where .then() and .catch() come into play: .then(): This is your “happy path” handler. It runs when the promise is fulfilled. .catch(): This handles any errors if the promise gets rejected. Check out this example: promise .then(result => { console.log(result); // Should log: "Pizza delivered on time!" }) .catch(error => { console.error(error); // Or log: "Pizza delivery failed." }); Pretty neat, right? It’s like having a plan for every scenario without getting tangled up in callbacks. Promise Chaining: Keeping It Clean Before promises came along, dealing with multiple asynchronous tasks often led us down the dark path of callback hell—where each new asynchronous action ended up nested inside another. With promises, you can chain actions together in a much cleaner way: getUser() .then(user => getPosts(user.id)) .then(posts => console.log(posts)) .catch(error => console.error("Something went wrong:", error)); Each .then() returns a new promise, so you can line up asynchronous tasks like a neat row of dominoes. Real-World Example: Fetching Data Let’s say you need to fetch some data from an API. The built-in fetch function returns a promise, which you can handle like this: fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log("Data received:", data)) .catch(error => console.error("Error fetching data:", error)); Here, fetch does its job in the background while your code handles the eventual response or any potential errors gracefully. Advanced Techniques: Promise.all() and Promise.race() Promises really shine when you need to coordinate multiple asynchronous tasks. Here are a couple of handy techniques: Promise.all() This method takes an array of promises and resolves when all of them complete, or rejects if any one of them fails. It’s great for tasks that can run in parallel: Promise.all([fetchData1(), fetchData2()]) .then(([data1, data2]) => { console.log("Both data sets received:", data1, data2); }) .catch(error => console.error("One of the promises failed:", error)); Promise.race() If you need the result of the fastest promise, you can use Promise.race(). This returns the result or error of the first promise that settles: Promise.race([fetchFromSource1(), fetchFromSource2()]) .then(result => { console.log("The fastest data:", result); }) .catch(error => console.error("Error in race:", error)); Wrapping It Up JavaScript promises are a powerful way to handle asynchronous operations. They not only clean up your code by avoiding deeply nested callbacks but also provide flexible tools for dealing with multiple operations at once. Once you get comfortable using them, you'll find your code is more robust and easier to manage. So next time you see a promise in your code, think of it as your friendly little assistant, taking care of tasks behind the scenes while you build amazing functionality.
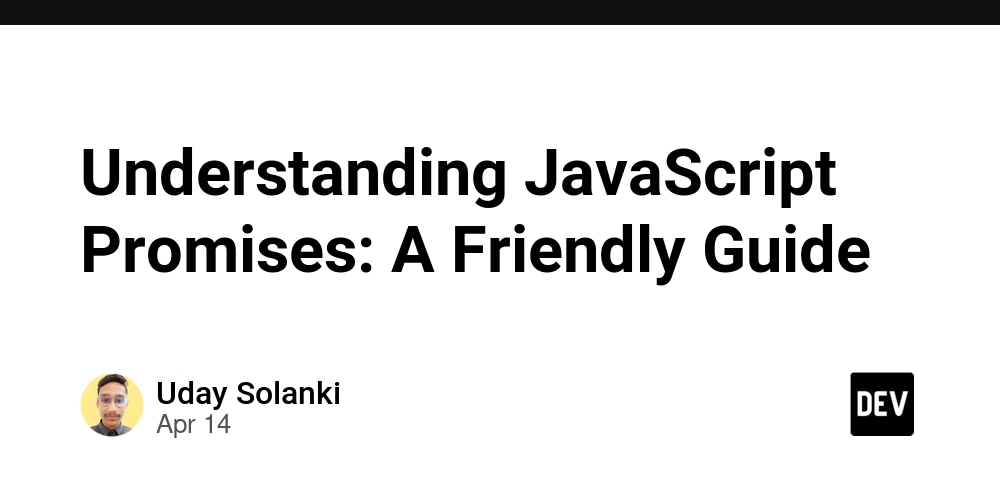
Hey there! If you've been diving into JavaScript and stumbled upon promises, you might feel a mix of curiosity and a bit of dread. Don't worry—you're not alone. I once felt overwhelmed by the idea of asynchronous code, but let me share with you how promises can actually make your life a lot easier.
So, What's a Promise Anyway?
Imagine ordering your favorite pizza. When you call for delivery, you don't just sit on your phone waiting for the pizza guy to show up. Instead, you carry on with your day. A Promise in JavaScript works in the same way: it's an object that represents the future result of an asynchronous task. It might succeed, it might fail, but either way, it’s out there doing its thing while your code keeps running.
In technical terms, a promise has three possible states:
- Pending: The task is still in progress.
- Fulfilled: The task completed successfully.
- Rejected: The task failed.
Here's a quick example:
const promise = new Promise((resolve, reject) => {
// Imagine some asynchronous operation here
const isSuccess = true; // you can change this to false to see what happens
if (isSuccess) {
resolve("Pizza delivered on time!");
} else {
reject("Pizza delivery failed.");
}
});
Working With Promises: .then()
and .catch()
Once you have a promise, you need to handle what happens next. That’s where .then()
and .catch()
come into play:
-
.then()
: This is your “happy path” handler. It runs when the promise is fulfilled. -
.catch()
: This handles any errors if the promise gets rejected.
Check out this example:
promise
.then(result => {
console.log(result); // Should log: "Pizza delivered on time!"
})
.catch(error => {
console.error(error); // Or log: "Pizza delivery failed."
});
Pretty neat, right? It’s like having a plan for every scenario without getting tangled up in callbacks.
Promise Chaining: Keeping It Clean
Before promises came along, dealing with multiple asynchronous tasks often led us down the dark path of callback hell—where each new asynchronous action ended up nested inside another. With promises, you can chain actions together in a much cleaner way:
getUser()
.then(user => getPosts(user.id))
.then(posts => console.log(posts))
.catch(error => console.error("Something went wrong:", error));
Each .then()
returns a new promise, so you can line up asynchronous tasks like a neat row of dominoes.
Real-World Example: Fetching Data
Let’s say you need to fetch some data from an API. The built-in fetch
function returns a promise, which you can handle like this:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log("Data received:", data))
.catch(error => console.error("Error fetching data:", error));
Here, fetch
does its job in the background while your code handles the eventual response or any potential errors gracefully.
Advanced Techniques: Promise.all()
and Promise.race()
Promises really shine when you need to coordinate multiple asynchronous tasks. Here are a couple of handy techniques:
Promise.all()
This method takes an array of promises and resolves when all of them complete, or rejects if any one of them fails. It’s great for tasks that can run in parallel:
Promise.all([fetchData1(), fetchData2()])
.then(([data1, data2]) => {
console.log("Both data sets received:", data1, data2);
})
.catch(error => console.error("One of the promises failed:", error));
Promise.race()
If you need the result of the fastest promise, you can use Promise.race()
. This returns the result or error of the first promise that settles:
Promise.race([fetchFromSource1(), fetchFromSource2()])
.then(result => {
console.log("The fastest data:", result);
})
.catch(error => console.error("Error in race:", error));
Wrapping It Up
JavaScript promises are a powerful way to handle asynchronous operations. They not only clean up your code by avoiding deeply nested callbacks but also provide flexible tools for dealing with multiple operations at once. Once you get comfortable using them, you'll find your code is more robust and easier to manage.
So next time you see a promise in your code, think of it as your friendly little assistant, taking care of tasks behind the scenes while you build amazing functionality.