Tutorial 18: Using Combine for Reactive Programming in Swift
Reactive programming is a powerful paradigm for managing asynchronous and event-driven code in Swift. Apple's Combine framework provides a declarative Swift API for processing values over time. In this tutorial, we'll explore Combine in-depth and build a Funny Soundboard App to showcase its practical applications. What is Combine? Combine is a framework introduced in iOS 13 that provides tools for handling asynchronous events using publishers and subscribers. It allows you to write cleaner, more maintainable, and more expressive code for handling streams of values. Key Concepts in Combine Publisher: Emits a sequence of values over time. Subscriber: Receives values from a publisher and reacts accordingly. Operator: Modifies or transforms values from a publisher. Subject: A type of publisher that allows manual value emission. Cancellable: A token that represents an active subscription. Setting Up Our Project To follow along, create a new iOS project in Xcode and select SwiftUI as the interface. We’ll use Combine to manage event-driven interactions. Step 1: Adding Sound Effects First, let's gather some funny sound effects (e.g., laughter, buzzer sounds, animal noises). You can find royalty-free sounds online and add them to your Assets folder. Step 2: Creating the Soundboard Model import Combine import AVFoundation class SoundboardViewModel: ObservableObject { @Published var selectedSound: String? = nil private var player: AVAudioPlayer? func playSound(named soundName: String) { guard let url = Bundle.main.url(forResource: soundName, withExtension: "mp3") else { print("Sound not found") return } do { player = try AVAudioPlayer(contentsOf: url) player?.play() } catch { print("Error playing sound: \(error.localizedDescription)") } } } Step 3: Building the SwiftUI View import SwiftUI struct SoundboardView: View { @StateObject private var viewModel = SoundboardViewModel() let sounds = ["laugh", "buzzer", "meow", "applause"] var body: some View { VStack { Text("Funny Soundboard") .font(.largeTitle) .padding() ForEach(sounds, id: \ .self) { sound in Button(action: { viewModel.playSound(named: sound) }) { Text(sound.capitalized) .font(.headline) .padding() .frame(maxWidth: .infinity) .background(Color.blue) .foregroundColor(.white) .cornerRadius(10) } .padding(.horizontal) } } } } Step 4: Integrating Combine for Reactive Updates We want to observe and react to changes in selectedSound. Let’s add a Combine Publisher to our ViewModel: import Combine class SoundboardViewModel: ObservableObject { @Published var selectedSound: String? = nil private var player: AVAudioPlayer? private var cancellables = Set() init() { $selectedSound .compactMap { $0 } .sink { [weak self] soundName in self?.playSound(named: soundName) } .store(in: &cancellables) } func selectSound(_ soundName: String) { selectedSound = soundName } } Step 5: Updating the UI to Use Reactive Selection Modify SoundboardView to use selectedSound: struct SoundboardView: View { @StateObject private var viewModel = SoundboardViewModel() let sounds = ["laugh", "buzzer", "meow", "applause"] var body: some View { VStack { Text("Funny Soundboard") .font(.largeTitle) .padding() ForEach(sounds, id: \ .self) { sound in Button(action: { viewModel.selectSound(sound) }) { Text(sound.capitalized) .font(.headline) .padding() .frame(maxWidth: .infinity) .background(Color.blue) .foregroundColor(.white) .cornerRadius(10) } .padding(.horizontal) } } } } Wrapping Up In this tutorial, we covered: The basics of Combine (Publishers, Subscribers, Operators, Subjects, Cancellables) How to use @Published to automatically react to changes How to build a Funny Soundboard App with SwiftUI and Combine This is just the beginning—Combine is a vast framework that can be used for networking, data binding, and complex event handling. Try extending this app with more sounds, animations, and effects! Happy coding!
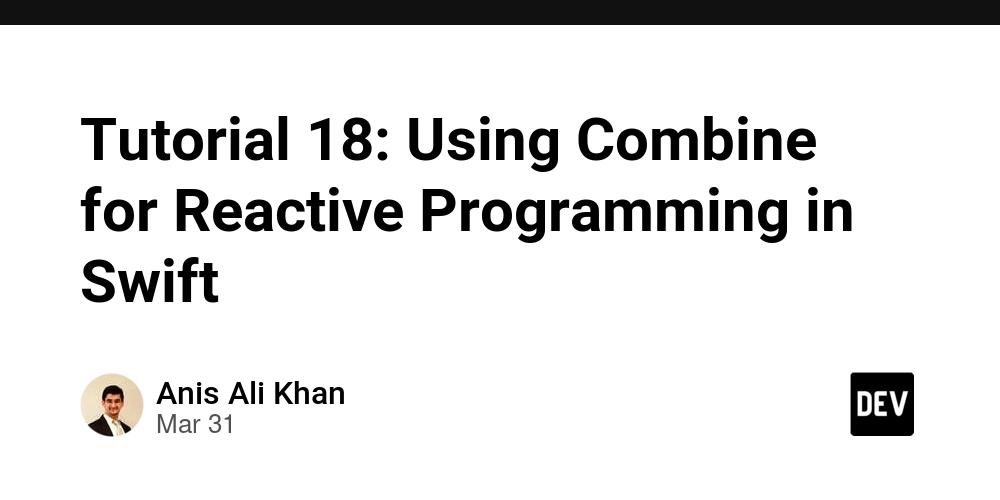
Reactive programming is a powerful paradigm for managing asynchronous and event-driven code in Swift. Apple's Combine framework provides a declarative Swift API for processing values over time. In this tutorial, we'll explore Combine in-depth and build a Funny Soundboard App to showcase its practical applications.
What is Combine?
Combine is a framework introduced in iOS 13 that provides tools for handling asynchronous events using publishers and subscribers. It allows you to write cleaner, more maintainable, and more expressive code for handling streams of values.
Key Concepts in Combine
- Publisher: Emits a sequence of values over time.
- Subscriber: Receives values from a publisher and reacts accordingly.
- Operator: Modifies or transforms values from a publisher.
- Subject: A type of publisher that allows manual value emission.
- Cancellable: A token that represents an active subscription.
Setting Up Our Project
To follow along, create a new iOS project in Xcode and select SwiftUI as the interface. We’ll use Combine to manage event-driven interactions.
Step 1: Adding Sound Effects
First, let's gather some funny sound effects (e.g., laughter, buzzer sounds, animal noises). You can find royalty-free sounds online and add them to your Assets folder.
Step 2: Creating the Soundboard Model
import Combine
import AVFoundation
class SoundboardViewModel: ObservableObject {
@Published var selectedSound: String? = nil
private var player: AVAudioPlayer?
func playSound(named soundName: String) {
guard let url = Bundle.main.url(forResource: soundName, withExtension: "mp3") else {
print("Sound not found")
return
}
do {
player = try AVAudioPlayer(contentsOf: url)
player?.play()
} catch {
print("Error playing sound: \(error.localizedDescription)")
}
}
}
Step 3: Building the SwiftUI View
import SwiftUI
struct SoundboardView: View {
@StateObject private var viewModel = SoundboardViewModel()
let sounds = ["laugh", "buzzer", "meow", "applause"]
var body: some View {
VStack {
Text("Funny Soundboard")
.font(.largeTitle)
.padding()
ForEach(sounds, id: \ .self) { sound in
Button(action: {
viewModel.playSound(named: sound)
}) {
Text(sound.capitalized)
.font(.headline)
.padding()
.frame(maxWidth: .infinity)
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
}
.padding(.horizontal)
}
}
}
}
Step 4: Integrating Combine for Reactive Updates
We want to observe and react to changes in selectedSound
. Let’s add a Combine Publisher to our ViewModel:
import Combine
class SoundboardViewModel: ObservableObject {
@Published var selectedSound: String? = nil
private var player: AVAudioPlayer?
private var cancellables = Set<AnyCancellable>()
init() {
$selectedSound
.compactMap { $0 }
.sink { [weak self] soundName in
self?.playSound(named: soundName)
}
.store(in: &cancellables)
}
func selectSound(_ soundName: String) {
selectedSound = soundName
}
}
Step 5: Updating the UI to Use Reactive Selection
Modify SoundboardView
to use selectedSound
:
struct SoundboardView: View {
@StateObject private var viewModel = SoundboardViewModel()
let sounds = ["laugh", "buzzer", "meow", "applause"]
var body: some View {
VStack {
Text("Funny Soundboard")
.font(.largeTitle)
.padding()
ForEach(sounds, id: \ .self) { sound in
Button(action: {
viewModel.selectSound(sound)
}) {
Text(sound.capitalized)
.font(.headline)
.padding()
.frame(maxWidth: .infinity)
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(10)
}
.padding(.horizontal)
}
}
}
}
Wrapping Up
In this tutorial, we covered:
- The basics of Combine (Publishers, Subscribers, Operators, Subjects, Cancellables)
- How to use
@Published
to automatically react to changes - How to build a Funny Soundboard App with SwiftUI and Combine
This is just the beginning—Combine is a vast framework that can be used for networking, data binding, and complex event handling. Try extending this app with more sounds, animations, and effects!
Happy coding!