The Hidden Power of Bitwise Operations: A Programmer’s Secret Weapon
Hey there, fellow code enthusiasts! Today, let’s dive into a corner of programming that’s often overlooked but packs a surprising punch: bitwise operations. These little tricks operate at the binary level, manipulating the very bits that make up our data. They’re fast, elegant, and can solve problems in ways you’d never expect. This is going to be short, sweet, and packed with insight. What Are Bitwise Operations? At their core, bitwise operations work directly on the binary representations of numbers. The main players are: AND (&): Compares bits, outputs 1 only if both are 1. OR (|): Outputs 1 if at least one bit is 1. XOR (^): Outputs 1 if bits differ. NOT (~): Flips all bits. Left Shift (): Shifts bits right, dividing by powers of 2. Sounds basic, right? But their real magic shines in unexpected use cases. Why Should You Care? Speed: Bitwise ops are lightning-fast because they’re handled directly by the CPU—no loops, no overhead. Space: They can compress logic that would otherwise take multiple lines or data structures. Clever Solutions: Some problems that seem complex unravel beautifully with a bit-level twist. A Real-World Gem: Checking Odd or Even Forget modulo (% 2). Want to check if a number is odd? Use n & 1. Why? The least significant bit of any odd number is 1, and even is 0. AND it with 1, and you’ve got your answer in a single operation. Try it: 5 (binary 101) & 1 (binary 001) = 1 → Odd 4 (binary 100) & 1 (binary 001) = 0 → Even The XOR Swap Trick Need to swap two integers without a temp variable? XOR has your back: a ^= b; b ^= a; a ^= b; Why it works: XORing a number with itself cancels it out, and doing this dance preserves the values. It’s niche, but when memory’s tight, it’s a lifesaver. Bit Flags: Packing Data Like a Pro Imagine storing multiple boolean states (e.g., permissions: read, write, execute) in one integer: Read = 1 (001) Write = 2 (010) Execute = 4 (100) Combine them with OR: flags = 1 | 2 | 4 → 7 (111). Check with AND: flags & 2 → 2 (write is set). This is how operating systems and games squeeze efficiency out of every byte. Final Thought Bitwise operations are like the Swiss Army knife you forgot you had. They’re not glamorous, but once you start seeing the world in bits, you’ll spot opportunities everywhere—optimization, algorithms, even interviews. Next time you’re coding, ask: “Can I solve this with bits?” You might surprise yourself. Until next time, keep exploring the edges of code. Follow me for more bite-sized wisdom!
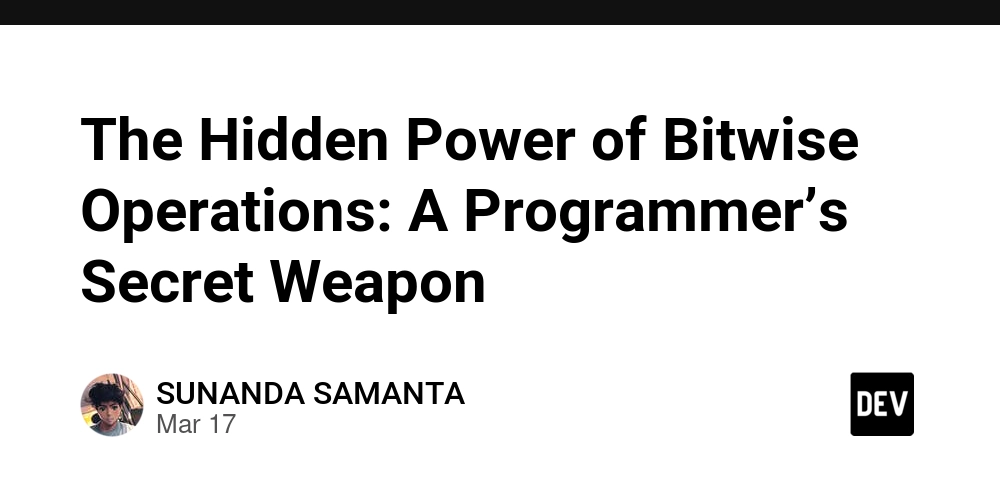
Hey there, fellow code enthusiasts! Today, let’s dive into a corner of programming that’s often overlooked but packs a surprising punch: bitwise operations. These little tricks operate at the binary level, manipulating the very bits that make up our data. They’re fast, elegant, and can solve problems in ways you’d never expect.
This is going to be short, sweet, and packed with insight.
What Are Bitwise Operations?
At their core, bitwise operations work directly on the binary representations of numbers. The main players are:
- AND (&): Compares bits, outputs 1 only if both are 1.
- OR (|): Outputs 1 if at least one bit is 1.
- XOR (^): Outputs 1 if bits differ.
- NOT (~): Flips all bits.
- Left Shift (<<): Shifts bits left, multiplying by powers of 2.
- Right Shift (>>): Shifts bits right, dividing by powers of 2.
Sounds basic, right? But their real magic shines in unexpected use cases.
Why Should You Care?
- Speed: Bitwise ops are lightning-fast because they’re handled directly by the CPU—no loops, no overhead.
- Space: They can compress logic that would otherwise take multiple lines or data structures.
- Clever Solutions: Some problems that seem complex unravel beautifully with a bit-level twist.
A Real-World Gem: Checking Odd or Even
Forget modulo (% 2). Want to check if a number is odd? Use n & 1. Why? The least significant bit of any odd number is 1, and even is 0. AND it with 1, and you’ve got your answer in a single operation. Try it:
5 (binary 101) & 1 (binary 001) = 1 → Odd
4 (binary 100) & 1 (binary 001) = 0 → Even
The XOR Swap Trick
Need to swap two integers without a temp variable? XOR has your back:
a ^= b;
b ^= a;
a ^= b;
Why it works: XORing a number with itself cancels it out, and doing this dance preserves the values. It’s niche, but when memory’s tight, it’s a lifesaver.
Bit Flags: Packing Data Like a Pro
Imagine storing multiple boolean states (e.g., permissions: read, write, execute) in one integer:
Read = 1 (001)
Write = 2 (010)
Execute = 4 (100)
Combine them with OR: flags = 1 | 2 | 4 → 7 (111). Check with AND: flags & 2 → 2 (write is set). This is how operating systems and games squeeze efficiency out of every byte.
Final Thought
Bitwise operations are like the Swiss Army knife you forgot you had. They’re not glamorous, but once you start seeing the world in bits, you’ll spot opportunities everywhere—optimization, algorithms, even interviews.
Next time you’re coding, ask: “Can I solve this with bits?” You might surprise yourself.
Until next time, keep exploring the edges of code. Follow me for more bite-sized wisdom!