Optimizing AWS SAM Node.js Lambda with esbuild
In the previous post, we created a basic Node.js Lambda function using AWS SAM. Now, we’re going to take it a step further by optimizing our build process using esbuild. This tool is a lightning-fast bundler and minifier that reduces deployment package size and speeds up cold start times. In this guide, we’ll: Add esbuild to our AWS SAM project Configure SAM to use esbuild as the build method Handle external dependencies Compile TypeScript (optional) Prerequisites Ensure you’ve completed the setup from Part 1, and you have a working AWS SAM project using Node.js. You’ll also need: Node.js installed esbuild (we’ll install it locally in the project) Step 1: Install esbuild Navigate to your Lambda function directory (e.g., hello-world/) and install esbuild: cd hello-world npm install --save-dev esbuild If you’re using TypeScript: npm install typescript Step 2: Update Your template.yml Modify your template.yml to tell AWS SAM to use esbuild for building your Lambda: Resources: HelloWorldFunction: Type: AWS::Serverless::Function Properties: Handler: index.handler Runtime: nodejs18.x Architectures: - x86_64 Events: HelloWorld: Type: Api Properties: Path: /hello Method: get Metadata: BuildMethod: esbuild BuildProperties: Minify: true Target: "es2020" Sourcemap: false EntryPoints: - index.ts External: - "@aws-sdk/*" Breakdown: BuildMethod: esbuild: Enables esbuild as the compiler. Minify: true: Reduces the size of the compiled output. EntryPoints: The main source file(s). External: Keeps packages like @aws-sdk/* out of the final zip (as they're available in AWS Lambda's environment). Conclusion Using esbuild with AWS SAM helps you deploy faster, smaller Lambda functions, and makes TypeScript support super easy. In the next post.
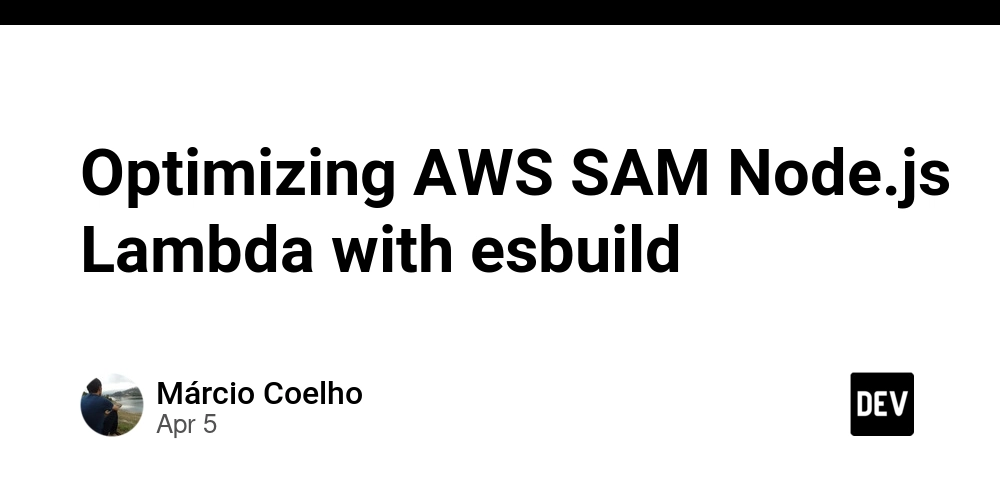
In the previous post, we created a basic Node.js Lambda function using AWS SAM. Now, we’re going to take it a step further by optimizing our build process using esbuild
. This tool is a lightning-fast bundler and minifier that reduces deployment package size and speeds up cold start times.
In this guide, we’ll:
Add
esbuild
to our AWS SAM projectConfigure SAM to use
esbuild
as the build methodHandle external dependencies
Compile TypeScript (optional)
Prerequisites
Ensure you’ve completed the setup from Part 1, and you have a working AWS SAM project using Node.js.
You’ll also need:
Node.js installed
esbuild
(we’ll install it locally in the project)
Step 1: Install esbuild
Navigate to your Lambda function directory (e.g., hello-world/
) and install esbuild
:
cd hello-world
npm install --save-dev esbuild
If you’re using TypeScript:
npm install typescript
Step 2: Update Your template.yml
Modify your template.yml
to tell AWS SAM to use esbuild
for building your Lambda:
Resources:
HelloWorldFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs18.x
Architectures:
- x86_64
Events:
HelloWorld:
Type: Api
Properties:
Path: /hello
Method: get
Metadata:
BuildMethod: esbuild
BuildProperties:
Minify: true
Target: "es2020"
Sourcemap: false
EntryPoints:
- index.ts
External:
- "@aws-sdk/*"
Breakdown:
BuildMethod: esbuild
: Enablesesbuild
as the compiler.Minify: true
: Reduces the size of the compiled output.EntryPoints
: The main source file(s).External
: Keeps packages like@aws-sdk/*
out of the final zip (as they're available in AWS Lambda's environment).
Conclusion
Using esbuild
with AWS SAM helps you deploy faster, smaller Lambda functions, and makes TypeScript support super easy. In the next post.