Mastering Structured Logging in Go: A Developer's Guide to Better Observability
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world! Structured logging in Golang provides critical observability for applications. As applications grow in complexity, effective logging becomes essential for debugging, performance monitoring, and security auditing. I've spent years refining logging strategies in production systems, and I'm excited to share what I've learned about implementing structured logging in Go. Understanding Structured Logging Structured logging moves beyond traditional text-based logs to create machine-parseable data with consistent fields. Instead of arbitrary strings, logs become searchable, filterable JSON objects containing rich metadata. Traditional logging looks like this: INFO: User john.doe logged in from 192.168.1.1 at 2023-05-15T14:32:10Z While structured logging resembles: { "level": "info", "timestamp": "2023-05-15T14:32:10Z", "message": "User login successful", "user_id": "john.doe", "ip_address": "192.168.1.1", "service": "auth", "request_id": "req-123abc" } This approach offers significant advantages for analysis, especially at scale. Popular Logging Libraries in Go Several libraries support structured logging in Go, each with unique strengths: Zap: Developed by Uber, offering exceptional performance with minimal allocations Zerolog: Focused on zero-allocation logging for high-performance scenarios Logrus: Feature-rich with extensive middleware support slog: Standard library structured logging introduced in Go 1.21 I'll focus primarily on Zap for its balance of performance and features. Setting Up Zap Logger First, let's implement a basic Zap logger: package main import ( "go.uber.org/zap" "go.uber.org/zap/zapcore" ) func initLogger(environment string) (*zap.Logger, error) { var config zap.Config if environment == "production" { // Production uses JSON format config = zap.NewProductionConfig() // Customize timestamp format config.EncoderConfig.TimeKey = "timestamp" config.EncoderConfig.EncodeTime = zapcore.ISO8601TimeEncoder } else { // Development uses console format with colors config = zap.NewDevelopmentConfig() config.EncoderConfig.EncodeLevel = zapcore.CapitalColorLevelEncoder } return config.Build() } func main() { logger, err := initLogger("development") if err != nil { panic("Failed to initialize logger: " + err.Error()) } defer logger.Sync() // Basic logging logger.Info("Application started", zap.String("app_version", "1.0.0"), zap.Int("port", 8080), ) // Error logging with stack trace logger.Error("Connection failed", zap.String("database", "users_db"), zap.Error(errors.New("connection timeout")), ) } This setup creates an environment-aware logger that outputs colored, readable logs during development and JSON logs in production. Implementing a Logger Wrapper To ensure consistency across your application, create a logger wrapper: package logger import ( "context" "os" "go.uber.org/zap" "go.uber.org/zap/zapcore" ) type Logger struct { zap *zap.Logger } func New() *Logger { environment := os.Getenv("GO_ENV") var config zap.Config if environment == "production" { config = zap.NewProductionConfig() config.EncoderConfig.TimeKey = "timestamp" config.EncoderConfig.EncodeTime = zapcore.ISO8601TimeEncoder } else { config = zap.NewDevelopmentConfig() config.EncoderConfig.EncodeLevel = zapcore.CapitalColorLevelEncoder } logger, _ := config.Build() return &Logger{zap: logger} } func (l *Logger) With(fields ...zap.Field) *Logger { return &Logger{zap: l.zap.With(fields...)} } func (l *Logger) WithContext(ctx context.Context) *Logger { if requestID, ok := ctx.Value("request_id").(string); ok { return l.With(zap.String("request_id", requestID)) } return l } func (l *Logger) Info(msg string, fields ...zap.Field) { l.zap.Info(msg, fields...) } func (l *Logger) Error(msg string, err error, fields ...zap.Field) { fieldsCopy := make([]zap.Field, 0, len(fields)+1) fieldsCopy = append(fieldsCopy, fields...) fieldsCopy = append(fieldsCopy, zap.Error(err)) l.zap.Error(msg, fieldsCopy...) } func (l *Logger) Debug(msg string, fields ...zap.Field) { l.zap.Debug(msg, fields...) } func (l *Logger) Warn(msg string, fields ...zap.Field) { l.zap.Warn(msg, fields...) } func (l *Logger) Fatal(msg string, fields ...zap.Field) { l.zap.Fatal(msg, fields...) } func (l *Logger) Sync() error { return l.zap.Sync() } This wrapper simplifies logging usage while ensuring consistent log structure throughout your application. Context Pr
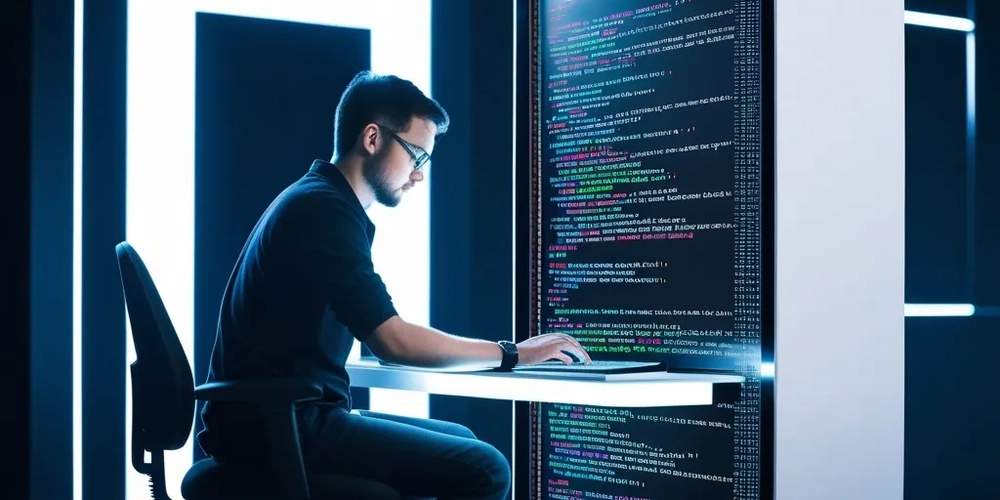
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Structured logging in Golang provides critical observability for applications. As applications grow in complexity, effective logging becomes essential for debugging, performance monitoring, and security auditing. I've spent years refining logging strategies in production systems, and I'm excited to share what I've learned about implementing structured logging in Go.
Understanding Structured Logging
Structured logging moves beyond traditional text-based logs to create machine-parseable data with consistent fields. Instead of arbitrary strings, logs become searchable, filterable JSON objects containing rich metadata.
Traditional logging looks like this:
INFO: User john.doe logged in from 192.168.1.1 at 2023-05-15T14:32:10Z
While structured logging resembles:
{
"level": "info",
"timestamp": "2023-05-15T14:32:10Z",
"message": "User login successful",
"user_id": "john.doe",
"ip_address": "192.168.1.1",
"service": "auth",
"request_id": "req-123abc"
}
This approach offers significant advantages for analysis, especially at scale.
Popular Logging Libraries in Go
Several libraries support structured logging in Go, each with unique strengths:
- Zap: Developed by Uber, offering exceptional performance with minimal allocations
- Zerolog: Focused on zero-allocation logging for high-performance scenarios
- Logrus: Feature-rich with extensive middleware support
- slog: Standard library structured logging introduced in Go 1.21
I'll focus primarily on Zap for its balance of performance and features.
Setting Up Zap Logger
First, let's implement a basic Zap logger:
package main
import (
"go.uber.org/zap"
"go.uber.org/zap/zapcore"
)
func initLogger(environment string) (*zap.Logger, error) {
var config zap.Config
if environment == "production" {
// Production uses JSON format
config = zap.NewProductionConfig()
// Customize timestamp format
config.EncoderConfig.TimeKey = "timestamp"
config.EncoderConfig.EncodeTime = zapcore.ISO8601TimeEncoder
} else {
// Development uses console format with colors
config = zap.NewDevelopmentConfig()
config.EncoderConfig.EncodeLevel = zapcore.CapitalColorLevelEncoder
}
return config.Build()
}
func main() {
logger, err := initLogger("development")
if err != nil {
panic("Failed to initialize logger: " + err.Error())
}
defer logger.Sync()
// Basic logging
logger.Info("Application started",
zap.String("app_version", "1.0.0"),
zap.Int("port", 8080),
)
// Error logging with stack trace
logger.Error("Connection failed",
zap.String("database", "users_db"),
zap.Error(errors.New("connection timeout")),
)
}
This setup creates an environment-aware logger that outputs colored, readable logs during development and JSON logs in production.
Implementing a Logger Wrapper
To ensure consistency across your application, create a logger wrapper:
package logger
import (
"context"
"os"
"go.uber.org/zap"
"go.uber.org/zap/zapcore"
)
type Logger struct {
zap *zap.Logger
}
func New() *Logger {
environment := os.Getenv("GO_ENV")
var config zap.Config
if environment == "production" {
config = zap.NewProductionConfig()
config.EncoderConfig.TimeKey = "timestamp"
config.EncoderConfig.EncodeTime = zapcore.ISO8601TimeEncoder
} else {
config = zap.NewDevelopmentConfig()
config.EncoderConfig.EncodeLevel = zapcore.CapitalColorLevelEncoder
}
logger, _ := config.Build()
return &Logger{zap: logger}
}
func (l *Logger) With(fields ...zap.Field) *Logger {
return &Logger{zap: l.zap.With(fields...)}
}
func (l *Logger) WithContext(ctx context.Context) *Logger {
if requestID, ok := ctx.Value("request_id").(string); ok {
return l.With(zap.String("request_id", requestID))
}
return l
}
func (l *Logger) Info(msg string, fields ...zap.Field) {
l.zap.Info(msg, fields...)
}
func (l *Logger) Error(msg string, err error, fields ...zap.Field) {
fieldsCopy := make([]zap.Field, 0, len(fields)+1)
fieldsCopy = append(fieldsCopy, fields...)
fieldsCopy = append(fieldsCopy, zap.Error(err))
l.zap.Error(msg, fieldsCopy...)
}
func (l *Logger) Debug(msg string, fields ...zap.Field) {
l.zap.Debug(msg, fields...)
}
func (l *Logger) Warn(msg string, fields ...zap.Field) {
l.zap.Warn(msg, fields...)
}
func (l *Logger) Fatal(msg string, fields ...zap.Field) {
l.zap.Fatal(msg, fields...)
}
func (l *Logger) Sync() error {
return l.zap.Sync()
}
This wrapper simplifies logging usage while ensuring consistent log structure throughout your application.
Context Propagation for Request Tracing
Distributed systems benefit greatly from context-aware logging:
package main
import (
"context"
"net/http"
"github.com/google/uuid"
"go.uber.org/zap"
"yourapp/logger"
)
func main() {
log := logger.New()
defer log.Sync()
http.HandleFunc("/api/users", func(w http.ResponseWriter, r *http.Request) {
// Create request context with unique ID
requestID := uuid.New().String()
ctx := context.WithValue(r.Context(), "request_id", requestID)
// Create logger with request context
reqLogger := log.WithContext(ctx).With(
zap.String("handler", "getUsersHandler"),
)
reqLogger.Info("Processing request",
zap.String("method", r.Method),
zap.String("path", r.URL.Path),
)
// Process request...
if err := processRequest(ctx); err != nil {
reqLogger.Error("Request processing failed", err,
zap.Int("status_code", http.StatusInternalServerError),
)
w.WriteHeader(http.StatusInternalServerError)
return
}
reqLogger.Info("Request completed",
zap.Int("status_code", http.StatusOK),
)
w.WriteHeader(http.StatusOK)
})
log.Info("Server starting", zap.Int("port", 8080))
http.ListenAndServe(":8080", nil)
}
func processRequest(ctx context.Context) error {
// Simulate processing...
return nil
}
This pattern allows tracking requests through different components of your application by consistently propagating the request ID.
Microservice-Oriented Logging
In microservices, add service-specific fields to enhance debugging:
package main
import (
"context"
"net/http"
"github.com/google/uuid"
"go.uber.org/zap"
"yourapp/logger"
)
const serviceName = "auth-service"
const serviceVersion = "1.2.0"
func main() {
log := logger.New().With(
zap.String("service", serviceName),
zap.String("version", serviceVersion),
)
defer log.Sync()
http.HandleFunc("/api/login", func(w http.ResponseWriter, r *http.Request) {
requestID := uuid.New().String()
ctx := context.WithValue(r.Context(), "request_id", requestID)
reqLogger := log.WithContext(ctx).With(
zap.String("endpoint", "/api/login"),
)
reqLogger.Info("Login attempt",
zap.String("user_id", r.FormValue("username")),
zap.String("client_ip", r.RemoteAddr),
)
// Process login...
reqLogger.Info("Login successful",
zap.String("user_id", r.FormValue("username")),
)
w.WriteHeader(http.StatusOK)
})
log.Info("Auth service starting", zap.Int("port", 8080))
http.ListenAndServe(":8080", nil)
}
Adding service name, version, and endpoint information helps with troubleshooting across microservices.
Integrating with Middleware
Middleware can automate request logging:
package middleware
import (
"net/http"
"time"
"github.com/google/uuid"
"go.uber.org/zap"
"yourapp/logger"
)
func LoggingMiddleware(log *logger.Logger) func(http.Handler) http.Handler {
return func(next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
start := time.Now()
// Create request ID and add to context
requestID := uuid.New().String()
ctx := context.WithValue(r.Context(), "request_id", requestID)
r = r.WithContext(ctx)
// Add request ID to response headers
w.Header().Set("X-Request-ID", requestID)
// Create wrapped response writer to capture status code
wrappedWriter := newResponseWriter(w)
// Process request
next.ServeHTTP(wrappedWriter, r)
// Log request details after completion
log.WithContext(ctx).Info("HTTP request",
zap.String("method", r.Method),
zap.String("path", r.URL.Path),
zap.String("remote_addr", r.RemoteAddr),
zap.String("user_agent", r.UserAgent()),
zap.Int("status", wrappedWriter.status),
zap.Duration("duration", time.Since(start)),
)
})
}
}
// responseWriter is a wrapper for http.ResponseWriter to capture status code
type responseWriter struct {
http.ResponseWriter
status int
}
func newResponseWriter(w http.ResponseWriter) *responseWriter {
return &responseWriter{w, http.StatusOK}
}
func (rw *responseWriter) WriteHeader(code int) {
rw.status = code
rw.ResponseWriter.WriteHeader(code)
}
Implementing this middleware automatically logs every HTTP request, making it easier to trace request flow.
Advanced Zap Configuration
Fine-tune Zap for production needs:
func createProductionLogger() (*zap.Logger, error) {
encoderConfig := zap.NewProductionEncoderConfig()
encoderConfig.TimeKey = "timestamp"
encoderConfig.EncodeTime = zapcore.ISO8601TimeEncoder
encoderConfig.EncodeDuration = zapcore.MillisDurationEncoder
encoderConfig.StacktraceKey = "stacktrace"
// Configure log level
level := zap.NewAtomicLevelAt(zapcore.InfoLevel)
// Create core
core := zapcore.NewCore(
zapcore.NewJSONEncoder(encoderConfig),
zapcore.AddSync(os.Stdout),
level,
)
// Add sampling - reduce repetitive logs
sampledCore := zapcore.NewSamplerWithOptions(core, time.Second, 100, 10)
// Add stack traces for errors and above
return zap.New(
sampledCore,
zap.AddCaller(),
zap.AddCallerSkip(1),
zap.AddStacktrace(zapcore.ErrorLevel),
), nil
}
This configuration enables log sampling (reducing high-volume logs) and adds stack traces to error logs automatically.
Integrating with Third-Party Systems
For log aggregation systems like ELK or cloud providers:
func initCloudLogger() (*zap.Logger, error) {
encoderConfig := zap.NewProductionEncoderConfig()
encoderConfig.TimeKey = "timestamp"
encoderConfig.EncodeTime = zapcore.ISO8601TimeEncoder
// Add cloud-specific fields
serviceName := os.Getenv("SERVICE_NAME")
region := os.Getenv("REGION")
environment := os.Getenv("ENVIRONMENT")
logger := zap.New(
zapcore.NewCore(
zapcore.NewJSONEncoder(encoderConfig),
zapcore.AddSync(os.Stdout),
zap.NewAtomicLevelAt(zapcore.InfoLevel),
),
)
// Add standard fields for all logs
return logger.With(
zap.String("service", serviceName),
zap.String("region", region),
zap.String("environment", environment),
), nil
}
Adding cloud metadata to logs makes filtering and tracing easier in distributed environments.
Logging Best Practices
I've learned several key principles for effective logging:
- Log actionable information: Focus on what's needed to understand system behavior
- Consistent field names: Standardize field names across your organization
- Appropriate log levels: Use DEBUG for development details, INFO for normal operations, WARN for potential issues, ERROR for failures
- Include context: Add user IDs, request IDs, service names, etc.
- Sensitive data handling: Never log passwords, tokens, or PII
Here's a practical implementation of these practices:
// UserService.go
func (s *UserService) CreateUser(ctx context.Context, user User) (string, error) {
log := s.logger.WithContext(ctx).With(
zap.String("operation", "CreateUser"),
)
log.Debug("Validating user input")
if err := user.Validate(); err != nil {
log.Warn("User validation failed", zap.Error(err))
return "", err
}
log.Debug("Checking if user exists",
zap.String("email", user.Email),
)
exists, err := s.repository.UserExists(ctx, user.Email)
if err != nil {
log.Error("Database error checking user existence", err)
return "", err
}
if exists {
log.Info("User already exists",
zap.String("email", user.Email),
)
return "", ErrUserExists
}
log.Debug("Hashing password")
hashedPassword, err := s.passwordService.HashPassword(user.Password)
if err != nil {
log.Error("Failed to hash password", err)
return "", err
}
// Never log sensitive data
user.Password = "[REDACTED]"
log.Debug("Creating user in database",
zap.Any("user", user),
)
userID, err := s.repository.CreateUser(ctx, user.WithPassword(hashedPassword))
if err != nil {
log.Error("Failed to create user in database", err)
return "", err
}
log.Info("User created successfully",
zap.String("user_id", userID),
zap.String("email", user.Email),
)
return userID, nil
}
The logs provide a clear path through the function's execution while avoiding sensitive data exposure.
Performance Considerations
Logging impacts performance, so consider these optimizations:
-
Avoid expensive serialization: Don't use
zap.Object()
for large structures - Use the appropriate logger: Use Zap's standard Logger for production performance
- Implement sampling: Reduce high-volume logs with sampling
- Log asynchronously: Consider async logging for performance-critical paths
- Benchmark your logging: Test your application with and without logging
Here's a performant logging setup:
func createHighPerformanceLogger() *zap.Logger {
config := zap.NewProductionConfig()
// Adjust these settings for performance
config.DisableCaller = true
config.DisableStacktrace = true
// Increase buffering
config.OutputPaths = []string{"stdout"}
logger, _ := config.Build(
zap.WithClock(zapcore.DefaultClock),
zap.AddCallerSkip(1),
)
return logger
}
// When logging in tight loops, check level first
func logInTightLoop(logger *zap.Logger, items []string) {
for _, item := range items {
// Avoid unnecessary allocations by checking if level is enabled
if logger.Core().Enabled(zapcore.DebugLevel) {
logger.Debug("Processing item", zap.String("item", item))
}
// Process item...
}
}
These techniques significantly reduce the performance impact of logging in high-throughput scenarios.
Testing with Structured Logs
Testing structured logging requires special approaches:
package logger_test
import (
"bytes"
"encoding/json"
"testing"
"go.uber.org/zap"
"go.uber.org/zap/zapcore"
"yourapp/logger"
)
func TestStructuredLogging(t *testing.T) {
// Create in-memory buffer for testing
var buf bytes.Buffer
// Create encoder that writes to buffer
encoder := zapcore.NewJSONEncoder(zap.NewProductionEncoderConfig())
core := zapcore.NewCore(encoder, zapcore.AddSync(&buf), zapcore.InfoLevel)
testLogger := zap.New(core)
// Create test logger
log := &logger.Logger{Zap: testLogger}
// Log a test message
log.Info("Test message",
zap.String("test_field", "test_value"),
zap.Int("count", 42),
)
// Verify log output
var logMap map[string]interface{}
if err := json.Unmarshal(buf.Bytes(), &logMap); err != nil {
t.Fatalf("Failed to parse log output: %v", err)
}
// Assert log fields
if msg, ok := logMap["msg"].(string); !ok || msg != "Test message" {
t.Errorf("Expected message 'Test message', got %v", logMap["msg"])
}
if val, ok := logMap["test_field"].(string); !ok || val != "test_value" {
t.Errorf("Expected test_field 'test_value', got %v", logMap["test_field"])
}
if count, ok := logMap["count"].(float64); !ok || int(count) != 42 {
t.Errorf("Expected count 42, got %v", logMap["count"])
}
}
This approach validates both log structure and content.
Implementing a Complete Solution
Bringing everything together, here's a comprehensive logging solution for a Go application:
package logger
import (
"context"
"os"
"time"
"go.uber.org/zap"
"go.uber.org/zap/zapcore"
)
// Application-wide field keys
const (
KeyRequestID = "request_id"
KeyUserID = "user_id"
KeyService = "service"
KeyComponent = "component"
KeyEnvironment = "environment"
KeyVersion = "version"
)
// Logger wraps zap logger
type Logger struct {
zap *zap.Logger
}
// New creates a new logger
func New() *Logger {
var config zap.Config
env := os.Getenv("GO_ENV")
if env == "production" {
config = zap.NewProductionConfig()
config.EncoderConfig.TimeKey = "timestamp"
config.EncoderConfig.EncodeTime = zapcore.ISO8601TimeEncoder
// Sample logs in production to prevent flooding
config.Sampling = &zap.SamplingConfig{
Initial: 100,
Thereafter: 100,
Hook: func(entry zapcore.Entry, dropped int) {
if dropped > 0 {
// Log a message about dropped logs if needed
}
},
}
} else {
config = zap.NewDevelopmentConfig()
config.EncoderConfig.EncodeLevel = zapcore.CapitalColorLevelEncoder
}
// Add app-wide fields
logger, _ := config.Build(
zap.AddCaller(),
zap.AddCallerSkip(1),
zap.AddStacktrace(zapcore.ErrorLevel),
zap.Fields(
zap.String(KeyService, os.Getenv("SERVICE_NAME")),
zap.String(KeyEnvironment, env),
zap.String(KeyVersion, os.Getenv("APP_VERSION")),
),
)
return &Logger{zap: logger}
}
// With creates a child logger with additional fields
func (l *Logger) With(fields ...zap.Field) *Logger {
return &Logger{zap: l.zap.With(fields...)}
}
// WithComponent adds component name to the logger
func (l *Logger) WithComponent(component string) *Logger {
return &Logger{zap: l.zap.With(zap.String(KeyComponent, component))}
}
// WithContext extracts context values and adds them to the logger
func (l *Logger) WithContext(ctx context.Context) *Logger {
newLogger := l.zap
// Add request ID if present
if requestID, ok := ctx.Value(KeyRequestID).(string); ok {
newLogger = newLogger.With(zap.String(KeyRequestID, requestID))
}
// Add user ID if present
if userID, ok := ctx.Value(KeyUserID).(string); ok {
newLogger = newLogger.With(zap.String(KeyUserID, userID))
}
return &Logger{zap: newLogger}
}
// Debug logs a debug message
func (l *Logger) Debug(msg string, fields ...zap.Field) {
l.zap.Debug(msg, fields...)
}
// Info logs an info message
func (l *Logger) Info(msg string, fields ...zap.Field) {
l.zap.Info(msg, fields...)
}
// Warn logs a warning message
func (l *Logger) Warn(msg string, fields ...zap.Field) {
l.zap.Warn(msg, fields...)
}
// Error logs an error message
func (l *Logger) Error(msg string, err error, fields ...zap.Field) {
if err != nil {
fields = append(fields, zap.Error(err))
}
l.zap.Error(msg, fields...)
}
// Fatal logs a fatal message and exits
func (l *Logger) Fatal(msg string, fields ...zap.Field) {
l.zap.Fatal(msg, fields...)
}
// Trace logs the start and end of a function with timing
func (l *Logger) Trace(ctx context.Context, funcName string) func() {
logger := l.WithContext(ctx)
startTime := time.Now()
logger.Debug("Starting function", zap.String("function", funcName))
return func() {
duration := time.Since(startTime)
logger.Debug("Function completed",
zap.String("function", funcName),
zap.Duration("duration", duration),
)
}
}
// Sync flushes any buffered logs
func (l *Logger) Sync() error {
return l.zap.Sync()
}
Usage in an application:
package main
import (
"context"
"net/http"
"github.com/google/uuid"
"go.uber.org/zap"
"yourapp/logger"
)
func main() {
log := logger.New()
defer log.Sync()
userService := NewUserService(log.WithComponent("user_service"))
authService := NewAuthService(log.WithComponent("auth_service"))
// Configure HTTP server with logging middleware
server := &http.Server{
Addr: ":8080",
Handler: loggingMiddleware(log, http.DefaultServeMux),
}
http.HandleFunc("/api/users", userHandler(userService, log))
http.HandleFunc("/api/login", loginHandler(authService, log))
log.Info("Server starting", zap.String("address", server.Addr))
if err := server.ListenAndServe(); err != nil {
log.Fatal("Server failed", zap.Error(err))
}
}
func loggingMiddleware(log *logger.Logger, next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
requestID := uuid.New().String()
ctx := context.WithValue(r.Context(), logger.KeyRequestID, requestID)
// Add request ID to response headers
w.Header().Set("X-Request-ID", requestID)
reqLogger := log.WithContext(ctx)
reqLogger.Info("Request started",
zap.String("method", r.Method),
zap.String("path", r.URL.Path),
zap.String("remote_addr", r.RemoteAddr),
)
start := time.Now()
next.ServeHTTP(w, r.WithContext(ctx))
reqLogger.Info("Request completed",
zap.String("method", r.Method),
zap.String("path", r.URL.Path),
zap.Duration("duration", time.Since(start)),
)
})
}
func userHandler(userService *UserService, log *logger.Logger) http.HandlerFunc {
return func(w http.ResponseWriter, r *http.Request) {
defer log.WithContext(r.Context()).Trace(r.Context(), "userHandler")()
// Handler implementation...
}
}
func loginHandler(authService *AuthService, log *logger.Logger) http.HandlerFunc {
return func(w http.ResponseWriter, r *http.Request) {
ctx := r.Context()
reqLogger := log.WithContext(ctx)
username := r.FormValue("username")
reqLogger.Info("Login attempt",
zap.String("username", username),
zap.String("ip", r.RemoteAddr),
)
// Authentication logic...
// Set user ID in context after successful authentication
userID := "user-123" // From authentication
ctx = context.WithValue(ctx, logger.KeyUserID, userID)
log.WithContext(ctx).Info("Login successful")
// Continue handling request...
}
}
This implementation provides comprehensive structured logging with context awareness, component tagging, and performance optimization.
In my work with Go microservices, I've found structured logging to be transformative for system observability. The initial setup requires careful thought, but the payoff in debugging efficiency is enormous. By following these patterns, you'll gain deeper insights into your application behavior while maintaining high performance.
Remember that logging is a key component of observability, alongside metrics and tracing. A well-implemented structured logging solution forms the foundation for effective monitoring and troubleshooting in any Go application.
101 Books
101 Books is an AI-driven publishing company co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Our Creations
Be sure to check out our creations:
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva