Mastering Java Garbage Collection: A Deep Dive into Memory Management
Introduction Ever wondered how Java applications manage memory so efficiently without developers manually allocating and freeing memory? The secret lies in Garbage Collection (GC) a core feature of Java's runtime environment that automatically removes unused objects, preventing memory leaks and improving performance. In this article, we’ll break down the mechanics of garbage collection, explore different types of garbage collectors, and provide expert tips for optimizing GC performance. How Garbage Collection Works in Java Java applications run on the Java Virtual Machine (JVM), which takes care of memory management by allocating and freeing heap space as needed. When objects are no longer referenced, they become eligible for garbage collection, ensuring efficient memory utilization. The Three-Step Garbage Collection Process: Marking – The GC identifies and marks objects that are still reachable (i.e., in use). Sweeping – Unreachable objects are removed, freeing up memory. Compacting – The memory is reorganized to reduce fragmentation and improve allocation efficiency. Types of Garbage Collectors in Java The JVM provides different garbage collectors to cater to various application needs. Choosing the right GC strategy can significantly impact application performance. 1. Serial Garbage Collector Uses a single thread for garbage collection. Best suited for small applications with single-threaded environments. 2. Parallel Garbage Collector (Throughput Collector) Utilizes multiple threads to perform GC tasks. Ideal for high-throughput applications. 3. G1 (Garbage First) Garbage Collector Divides the heap into regions and prioritizes garbage-heavy regions first. Optimized for applications requiring low-pause times. 4. Z Garbage Collector (ZGC) Performs most GC tasks concurrently with application execution. Designed for large-scale applications with minimal pause times. 5. Shenandoah Garbage Collector A low-latency GC designed for real-time applications. Works in parallel with running threads, ensuring ultra-low pauses. Best Practices for Optimizing Garbage Collection Performance Want to keep your Java applications running smoothly? Here are some proven strategies to optimize GC efficiency: Choose the right GC algorithm based on your application's needs. Minimize unnecessary object creation to reduce GC workload. Use object pooling for frequently used objects instead of creating new ones. Tune JVM parameters such as -Xms, -Xmx, and -XX:+UseG1GC to optimize memory usage. Analyze GC logs using tools like VisualVM, JConsole, and GCViewer to monitor performance. Prevent memory leaks by closing unused resources and removing unnecessary references. Conclusion Garbage Collection in Java is more than just an automated memory manager—it’s a powerful tool that ensures applications remain efficient and responsive. Understanding how different GC algorithms work and following best practices can help developers reduce latency, improve performance, and optimize resource usage. Are you leveraging the best GC strategy for your Java applications? Share your thoughts and experiences in the comments below!
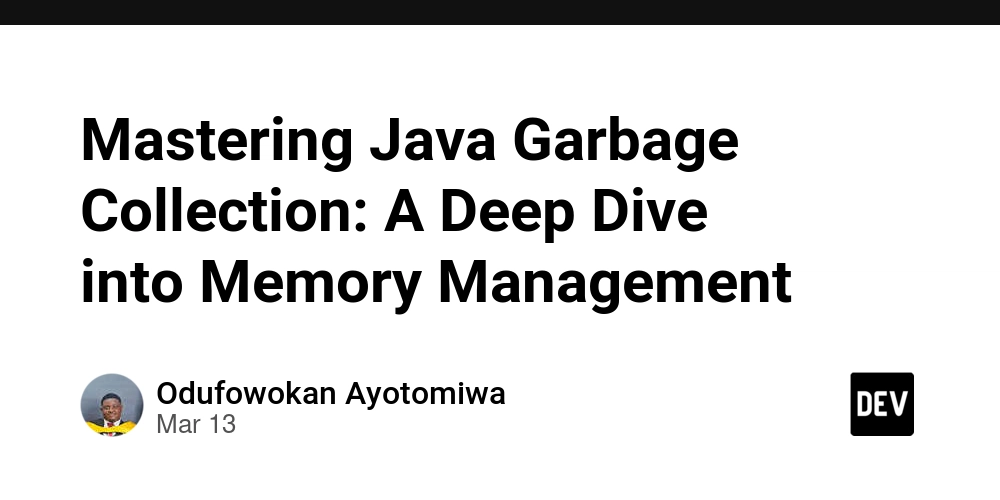
Introduction
Ever wondered how Java applications manage memory so efficiently without developers manually allocating and freeing memory? The secret lies in Garbage Collection (GC) a core feature of Java's runtime environment that automatically removes unused objects, preventing memory leaks and improving performance.
In this article, we’ll break down the mechanics of garbage collection, explore different types of garbage collectors, and provide expert tips for optimizing GC performance.
How Garbage Collection Works in Java
Java applications run on the Java Virtual Machine (JVM), which takes care of memory management by allocating and freeing heap space as needed. When objects are no longer referenced, they become eligible for garbage collection, ensuring efficient memory utilization.
The Three-Step Garbage Collection Process:
- Marking – The GC identifies and marks objects that are still reachable (i.e., in use).
- Sweeping – Unreachable objects are removed, freeing up memory.
- Compacting – The memory is reorganized to reduce fragmentation and improve allocation efficiency.
Types of Garbage Collectors in Java
The JVM provides different garbage collectors to cater to various application needs. Choosing the right GC strategy can significantly impact application performance.
1. Serial Garbage Collector
- Uses a single thread for garbage collection.
- Best suited for small applications with single-threaded environments.
2. Parallel Garbage Collector (Throughput Collector)
- Utilizes multiple threads to perform GC tasks.
- Ideal for high-throughput applications.
3. G1 (Garbage First) Garbage Collector
- Divides the heap into regions and prioritizes garbage-heavy regions first.
- Optimized for applications requiring low-pause times.
4. Z Garbage Collector (ZGC)
- Performs most GC tasks concurrently with application execution.
- Designed for large-scale applications with minimal pause times.
5. Shenandoah Garbage Collector
- A low-latency GC designed for real-time applications.
- Works in parallel with running threads, ensuring ultra-low pauses.
Best Practices for Optimizing Garbage Collection Performance
Want to keep your Java applications running smoothly? Here are some proven strategies to optimize GC efficiency:
- Choose the right GC algorithm based on your application's needs.
- Minimize unnecessary object creation to reduce GC workload.
- Use object pooling for frequently used objects instead of creating new ones.
-
Tune JVM parameters such as
-Xms
,-Xmx
, and-XX:+UseG1GC
to optimize memory usage. - Analyze GC logs using tools like VisualVM, JConsole, and GCViewer to monitor performance.
- Prevent memory leaks by closing unused resources and removing unnecessary references.
Conclusion
Garbage Collection in Java is more than just an automated memory manager—it’s a powerful tool that ensures applications remain efficient and responsive. Understanding how different GC algorithms work and following best practices can help developers reduce latency, improve performance, and optimize resource usage.
Are you leveraging the best GC strategy for your Java applications? Share your thoughts and experiences in the comments below!