JS Object.assign(), Object.create(). Object.defineProperties() Methods.
Object.assign() - The Object.assign() method copies properties from one or more source objects to a target object. const obj1 = { a: 1, b: 2 }; const obj2 = { c: 3, d: 4 }; console.log(obj1, obj2) // output: 1, 3, 4 Object.create() - creates an object from an existing object. is a method in JavaScript that creates a new object, setting the prototype of the new object to an existing object. This allows the new object to inherit properties and methods from the prototype object. const person = { greet: function(){ console.log(`Hello my name is ${this.name}`); } } const john = Object.create(person); john.name = 'John'; john.greet() // Hello my name is John Object.create() - is a JavaScript method that allows you to define multiple properties on an object at once. You can specify each property's characteristics, such as whether it's writable, enumerable, or configurable. const user = {}; Object.defineProperties(user, { firstName: { value: 'John', writable: true, // Can be modified enumerable: true, // Will appear in loops configurable: true, // Can be deleted or change }, age: { Value: 30, writable: false, // Cannot be modified enumerable: true, // Will appear in loops configurable: false, // Cannot be deleted or changed }, }) user.firstName = 'Alex' // output: Alex user.age = 23 // output 30
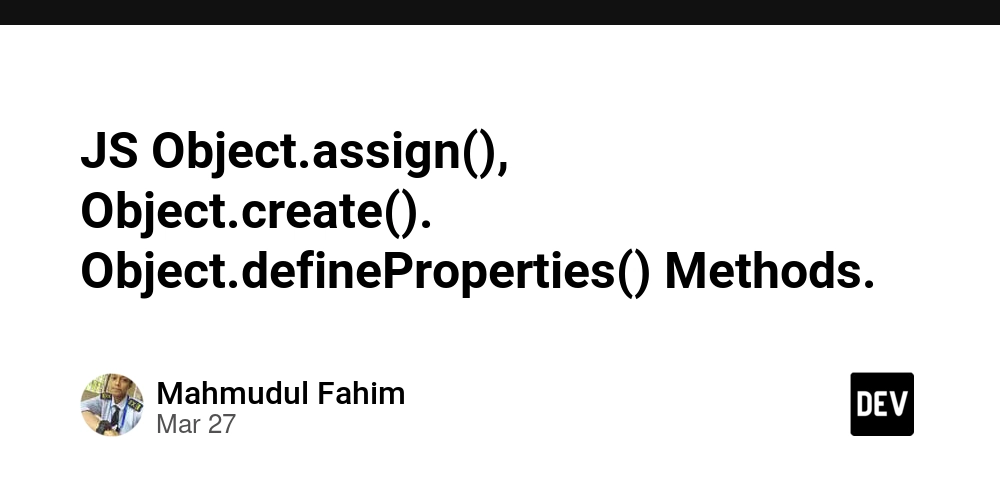
Object.assign() -
The Object.assign() method copies properties from one or more source objects to a target object.
const obj1 = { a: 1, b: 2 };
const obj2 = { c: 3, d: 4 };
console.log(obj1, obj2)
// output: 1, 3, 4
Object.create() -
creates an object from an existing object. is a method in JavaScript that creates a new object, setting the prototype of the new object to an existing object. This allows the new object to inherit properties and methods from the prototype object.
const person = {
greet: function(){
console.log(`Hello my name is ${this.name}`);
}
}
const john = Object.create(person);
john.name = 'John';
john.greet()
// Hello my name is John
Object.create() -
is a JavaScript method that allows you to define multiple properties on an object at once. You can specify each property's characteristics, such as whether it's writable, enumerable, or configurable.
const user = {};
Object.defineProperties(user, {
firstName: {
value: 'John',
writable: true, // Can be modified
enumerable: true, // Will appear in loops
configurable: true, // Can be deleted or change
},
age: {
Value: 30,
writable: false, // Cannot be modified
enumerable: true, // Will appear in loops
configurable: false, // Cannot be deleted or changed
},
})
user.firstName = 'Alex' // output: Alex
user.age = 23 // output 30