Introducing WBORM: A lightweight ORM with automatic introspection for JDBC databases
While support for modern databases is growing in the Python ecosystem, it is still common to face challenges when integrating with legacy systems like Informix, DB2, or Firebird. WBORM was created with that in mind: a lightweight, secure, and automated ORM for developers who want productivity without sacrificing clarity. WBORM is designed to work seamlessly with wbjdbc, which handles JVM initialization and JDBC connections to databases like Informix. Key Features Automatic introspection: generate Python models from real tables using generate_model() Powerful queries: full support for filter, filter_in, not_in, group_by, having, joins (automatic or manual), distinct() and subqueries Built-in safety: updates and deletes require both confirm=True and explicit where clause Lightweight hooks: before_add, after_update, validate() Optional caching for introspection and lazy-loaded relationships Data export as dictionary, JSON, or formatted table via tabulate Eager and lazy loading using .preload('relation') Why WBORM was created Popular ORMs like SQLAlchemy and Django ORM work great for modern SQL databases, but they tend to be complex or incompatible with JDBC and legacy systems. WBORM offers a simple and direct solution focused on what matters: Interact with databases via JDBC (using wbjdbc) No heavy frameworks or migration layers Reflect the real database schema, allowing safe and dynamic model mapping Easy to learn and highly productive Installation pip install wborm wbjdbc Quick Example from wborm import generate_model from wbjdbc import connect_to_db conn = connect_to_db(...) Cliente = generate_model("clientes", conn) clientes = Cliente.filter(ativo=True).order_by("nome").all() for c in clientes: c.show() Expected Output: +----+--------------+--------+ | id | nome | ativo | +====+==============+========+ | 1 | Ana Martins | True | +----+--------------+--------+ | 2 | João Rocha | True | +----+--------------+--------+ Learn More Repository: github.com/wanderbatistaf/wborm Documentation: wanderbatistaf.github.io/wborm Related project: wbjdbc on PyPI If you work with legacy databases and need a safe, introspective, and productive way to manage your data using Python, give WBORM a try. Feedback and contributions are very welcome.
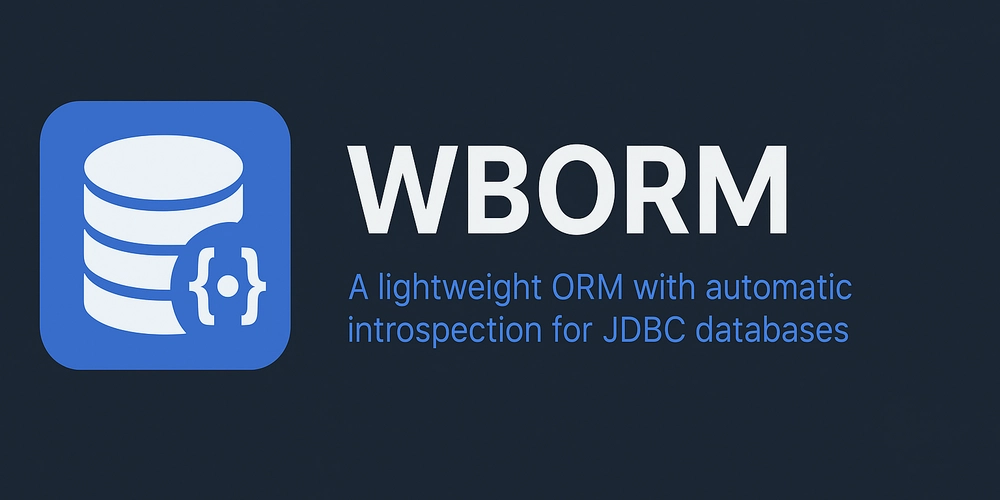
While support for modern databases is growing in the Python ecosystem, it is still common to face challenges when integrating with legacy systems like Informix, DB2, or Firebird. WBORM was created with that in mind: a lightweight, secure, and automated ORM for developers who want productivity without sacrificing clarity.
WBORM is designed to work seamlessly with wbjdbc, which handles JVM initialization and JDBC connections to databases like Informix.
Key Features
-
Automatic introspection: generate Python models from real tables using
generate_model()
-
Powerful queries: full support for
filter
,filter_in
,not_in
,group_by
,having
,joins
(automatic or manual),distinct()
and subqueries -
Built-in safety: updates and deletes require both
confirm=True
and explicitwhere
clause -
Lightweight hooks:
before_add
,after_update
,validate()
- Optional caching for introspection and lazy-loaded relationships
-
Data export as dictionary, JSON, or formatted table via
tabulate
-
Eager and lazy loading using
.preload('relation')
Why WBORM was created
Popular ORMs like SQLAlchemy and Django ORM work great for modern SQL databases, but they tend to be complex or incompatible with JDBC and legacy systems.
WBORM offers a simple and direct solution focused on what matters:
- Interact with databases via JDBC (using
wbjdbc
) - No heavy frameworks or migration layers
- Reflect the real database schema, allowing safe and dynamic model mapping
- Easy to learn and highly productive
Installation
pip install wborm wbjdbc
Quick Example
from wborm import generate_model
from wbjdbc import connect_to_db
conn = connect_to_db(...)
Cliente = generate_model("clientes", conn)
clientes = Cliente.filter(ativo=True).order_by("nome").all()
for c in clientes:
c.show()
Expected Output:
+----+--------------+--------+
| id | nome | ativo |
+====+==============+========+
| 1 | Ana Martins | True |
+----+--------------+--------+
| 2 | João Rocha | True |
+----+--------------+--------+
Learn More
- Repository: github.com/wanderbatistaf/wborm
- Documentation: wanderbatistaf.github.io/wborm
- Related project: wbjdbc on PyPI
If you work with legacy databases and need a safe, introspective, and productive way to manage your data using Python, give WBORM a try. Feedback and contributions are very welcome.