Implementing a Simple Asphalt Calculator in Python: A Step-by-Step Tutorial
Introduction Hello, Python enthusiasts! Today, we're going to roll up our sleeves and build a practical tool: a simple asphalt calculator. If you've ever wondered how much asphalt you need for a driveway or parking lot, this tutorial is for you. We'll be inspired by this asphalt calculator tool and implement its core calculation logic using Python. Don't worry, you won't need a hard hat—just your coding skills! Why is this important? Because calculating the right amount of asphalt is crucial. Too little, and your project stalls; too much, and you're wasting money. This calculator will help you get it just right. The Basic Formula Our calculator is based on two simple formulas: Volume = Length × Width × Depth Weight = Volume × Density Here's what these terms mean: Length and Width: The dimensions of the area you're paving, in meters (m). Depth: The thickness of the asphalt layer, in centimeters (cm). Density: The density of the asphalt mix, in kilograms per cubic meter (kg/m³). Wait, why centimeters for depth? In construction, depth is often measured in centimeters, but since density is in cubic meters, we need to convert depth to meters for consistency in volume calculation. The Importance of Units Unit consistency is key. We're assuming: Length and Width: meters (m) Depth: centimeters (cm) Density: kilograms per cubic meter (kg/m³) Volume: cubic meters (m³) Weight: kilograms (kg) Before calculating volume, we must convert depth from centimeters to meters. It's a small detail but crucial for accuracy. Python Code Implementation Let's dive into the code! We'll build this step by step, explaining each part in detail. Step 1: Define the Volume Calculation Function First, we need a function to calculate the volume. It takes length, width, and depth (in cm) as inputs, converts depth to meters, and returns the volume in cubic meters. def calculate_volume(length, width, depth_cm): depth_m = depth_cm / 100 # Convert cm to meters return length * width * depth_m This function is straightforward: convert depth to meters by dividing by 100, then multiply length, width, and depth to get the volume. Using a function makes the code modular and easier to understand. Step 2: Define the Weight Calculation Function Next, we create a function to calculate the weight of the asphalt. It takes volume and density as inputs and returns the weight. def calculate_weight(volume, density): return volume * density Again, a simple multiplication. This separation of concerns keeps the code clean and maintainable. Step 3: Get User Input Now, we need to get inputs from the user: length, width, depth, and density. Since these are numbers, we'll use float(input()) to read them. We'll also add basic error handling to ensure the inputs are valid numbers and positive. try: length = float(input("Enter length (meters): ")) width = float(input("Enter width (meters): ")) depth_cm = float(input("Enter depth (centimeters): ")) density = float(input("Enter density (kg/m³): ")) if length
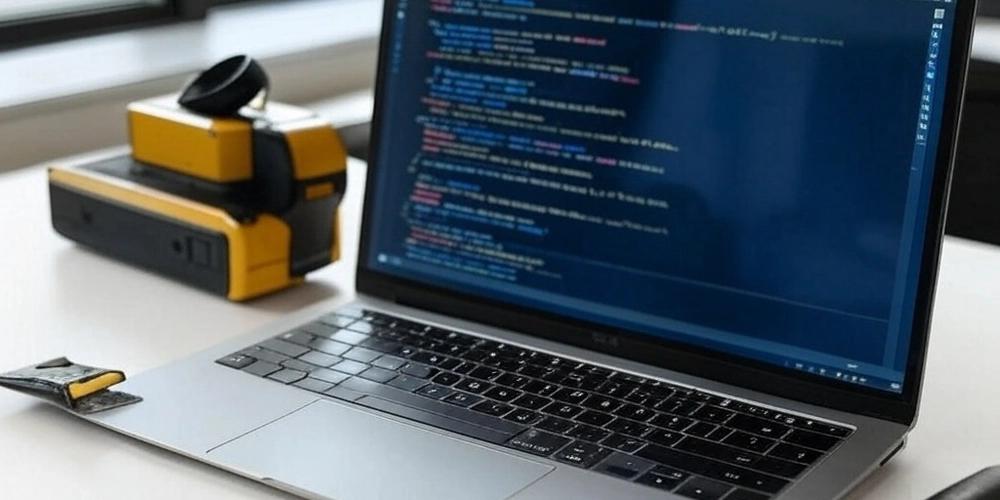
Introduction
Hello, Python enthusiasts! Today, we're going to roll up our sleeves and build a practical tool: a simple asphalt calculator. If you've ever wondered how much asphalt you need for a driveway or parking lot, this tutorial is for you. We'll be inspired by this asphalt calculator tool and implement its core calculation logic using Python. Don't worry, you won't need a hard hat—just your coding skills!
Why is this important? Because calculating the right amount of asphalt is crucial. Too little, and your project stalls; too much, and you're wasting money. This calculator will help you get it just right.
The Basic Formula
Our calculator is based on two simple formulas:
- Volume = Length × Width × Depth
- Weight = Volume × Density
Here's what these terms mean:
- Length and Width: The dimensions of the area you're paving, in meters (m).
- Depth: The thickness of the asphalt layer, in centimeters (cm).
- Density: The density of the asphalt mix, in kilograms per cubic meter (kg/m³).
Wait, why centimeters for depth? In construction, depth is often measured in centimeters, but since density is in cubic meters, we need to convert depth to meters for consistency in volume calculation.
The Importance of Units
Unit consistency is key. We're assuming:
- Length and Width: meters (m)
- Depth: centimeters (cm)
- Density: kilograms per cubic meter (kg/m³)
- Volume: cubic meters (m³)
- Weight: kilograms (kg)
Before calculating volume, we must convert depth from centimeters to meters. It's a small detail but crucial for accuracy.
Python Code Implementation
Let's dive into the code! We'll build this step by step, explaining each part in detail.
Step 1: Define the Volume Calculation Function
First, we need a function to calculate the volume. It takes length, width, and depth (in cm) as inputs, converts depth to meters, and returns the volume in cubic meters.
def calculate_volume(length, width, depth_cm):
depth_m = depth_cm / 100 # Convert cm to meters
return length * width * depth_m
This function is straightforward: convert depth to meters by dividing by 100, then multiply length, width, and depth to get the volume. Using a function makes the code modular and easier to understand.
Step 2: Define the Weight Calculation Function
Next, we create a function to calculate the weight of the asphalt. It takes volume and density as inputs and returns the weight.
def calculate_weight(volume, density):
return volume * density
Again, a simple multiplication. This separation of concerns keeps the code clean and maintainable.
Step 3: Get User Input
Now, we need to get inputs from the user: length, width, depth, and density. Since these are numbers, we'll use float(input()) to read them. We'll also add basic error handling to ensure the inputs are valid numbers and positive.
try:
length = float(input("Enter length (meters): "))
width = float(input("Enter width (meters): "))
depth_cm = float(input("Enter depth (centimeters): "))
density = float(input("Enter density (kg/m³): "))
if length <= 0 or width <= 0 or depth_cm <= 0 or density <= 0:
print("All values must be positive.")
else:
volume = calculate_volume(length, width, depth_cm)
weight = calculate_weight(volume, density)
print(f"Volume: {volume} cubic meters")
print(f"Weight: {weight} kilograms")
except ValueError:
print("Invalid input, please enter numbers.")
Let's break this down:
- try-except block: Catches ValueError if the user enters non-numeric input.
- Input validation: Checks if all values are greater than zero, as dimensions and density can't be negative or zero.
- Calculation and output: If inputs are valid, calculate volume and weight using the functions, then print the results.
This is also a great opportunity to introduce some Python basics:
- Functions: Reusable blocks of code for specific tasks.
- User input: Using input() to get data from the user and float() to convert strings to numbers.
- Error handling: Using try-except to gracefully handle invalid inputs.
- Conditionals: Using if-else to check if values are positive.
Example Calculation
Suppose you're paving a driveway that's 10 meters long, 3 meters wide, with a 5 cm thick asphalt layer, and the asphalt density is 2400 kg/m³. Here's how the calculation works:
- Convert depth to meters: 5 cm = 0.05 m
- Calculate volume: 10 m × 3 m × 0.05 m = 1.5 m³
- Calculate weight: 1.5 m³ × 2400 kg/m³ = 3600 kg So, you need 3600 kilograms of asphalt. Running the code and entering these values would output:
Volume: 1.5 cubic meters
Weight: 3600.0 kilograms
Perfect! Now you can confidently order just the right amount of asphalt.
Extending the Calculator
This is a basic calculator, but you could extend it with features like:
- Unit conversions for different measurement systems.
- Preset density options for different types of asphalt.
- Cost calculation based on weight and price per kilogram.
- Handling calculations for circular or other non-rectangular areas. If you want to add a user interface, consider frameworks like Flet, which lets you build web or mobile apps with Python.
Conclusion
In this tutorial, we've built a simple asphalt calculator using Python. We've learned how to:
- Define functions for modularity.
- Handle user input and validation.
- Perform calculations with unit conversions.
- Use basic error handling to make the program robust.
Whether you're new to programming or just exploring Python, I hope this tutorial has been helpful. Now, go ahead and try it out—pave the way for your next project, quite literally!