How to Develop an Online Event Booking System Using PHP & MySQL
An online event booking system allows users to browse, register, and purchase tickets for events conveniently. Developing such a system using PHP and MySQL ensures efficiency, scalability, and ease of maintenance. In this guide, we will explore the step-by-step process of building an event booking system with essential features. Prerequisites Before we begin, ensure you have the following installed on your system: PHP (Latest Version) MySQL Database Apache Server (XAMPP or WAMP recommended) A text editor or IDE (e.g., VS Code, Sublime Text) Step 1: Set Up the Project Structure Create a directory for your project and organize it as follows: event-booking-system/ │── index.php │── db_config.php │── register.php │── login.php │── dashboard.php │── book_event.php │── events.php │── logout.php │── css/ │── js/ │── images/ Step 2: Database Design Create a database named event_booking and design the necessary tables using the following SQL commands: CREATE DATABASE event_booking; USE event_booking; CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(100) NOT NULL, email VARCHAR(100) UNIQUE NOT NULL, password VARCHAR(255) NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ); CREATE TABLE events ( id INT AUTO_INCREMENT PRIMARY KEY, title VARCHAR(255) NOT NULL, description TEXT NOT NULL, date DATE NOT NULL, time TIME NOT NULL, venue VARCHAR(255) NOT NULL, available_seats INT NOT NULL, price DECIMAL(10,2) NOT NULL ); CREATE TABLE bookings ( id INT AUTO_INCREMENT PRIMARY KEY, user_id INT NOT NULL, event_id INT NOT NULL, booking_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP, FOREIGN KEY (user_id) REFERENCES users(id), FOREIGN KEY (event_id) REFERENCES events(id) ); Step 3: Database Connection Create a file db_config.php to establish a connection to MySQL. Step 4: User Registration & Login System Create register.php for user registration.
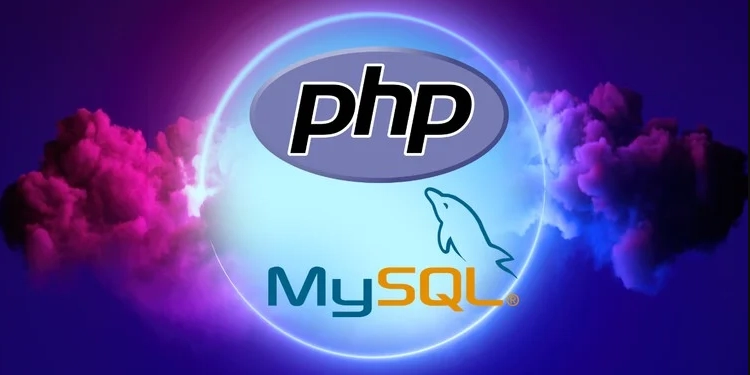
An online event booking system allows users to browse, register, and purchase tickets for events conveniently. Developing such a system using PHP and MySQL ensures efficiency, scalability, and ease of maintenance. In this guide, we will explore the step-by-step process of building an event booking system with essential features.
Prerequisites
Before we begin, ensure you have the following installed on your system:
PHP (Latest Version)
MySQL Database
Apache Server (XAMPP or WAMP recommended)
A text editor or IDE (e.g., VS Code, Sublime Text)
Step 1: Set Up the Project Structure
Create a directory for your project and organize it as follows:
event-booking-system/
│── index.php
│── db_config.php
│── register.php
│── login.php
│── dashboard.php
│── book_event.php
│── events.php
│── logout.php
│── css/
│── js/
│── images/
Step 2: Database Design
Create a database named event_booking and design the necessary tables using the following SQL commands:
CREATE DATABASE event_booking;
USE event_booking;
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) UNIQUE NOT NULL,
password VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
CREATE TABLE events (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL,
description TEXT NOT NULL,
date DATE NOT NULL,
time TIME NOT NULL,
venue VARCHAR(255) NOT NULL,
available_seats INT NOT NULL,
price DECIMAL(10,2) NOT NULL
);
CREATE TABLE bookings (
id INT AUTO_INCREMENT PRIMARY KEY,
user_id INT NOT NULL,
event_id INT NOT NULL,
booking_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (user_id) REFERENCES users(id),
FOREIGN KEY (event_id) REFERENCES events(id)
);
Step 3: Database Connection
Create a file db_config.php to establish a connection to MySQL.
connect_error) {
die("Connection failed: " . $conn->connect_error);
}
?>
Step 4: User Registration & Login System
Create register.php for user registration.
query($sql) === TRUE) {
echo "Registration successful!";
} else {
echo "Error: " . $conn->error;
}
}
?>
Create login.php for authentication.
query($sql);
if ($result->num_rows > 0) {
$row = $result->fetch_assoc();
if (password_verify($password, $row['password'])) {
$_SESSION['user_id'] = $row['id'];
header("Location: dashboard.php");
} else {
echo "Invalid credentials!";
}
} else {
echo "User not found!";
}
}
?>
Step 5: Display Events
Create events.php to list available events to make it easier for hosts to organize events on the event management system in PHP.
query($sql);
while ($row = $result->fetch_assoc()) {
echo "{$row['title']}
";
echo "{$row['description']}";
echo "
Price: \${$row['price']}";
echo "Book Now
";
}
?>
Step 6: Booking an Event
Create book_event.php to handle bookings.
query($sql) === TRUE) {
echo "Booking successful!";
} else {
echo "Error: " . $conn->error;
}
}
?>
Step 7: Dashboard
Create dashboard.php for users to view their bookings.
query($sql);
while ($row = $result->fetch_assoc()) {
echo "{$row['title']}";
}
?>
Conclusion
By following these steps, you have successfully built a functional event booking system using PHP and MySQL. You can further enhance it by adding features like payment integration, email notifications, and an admin panel for event management.
Would you like to add more features or need any modifications? Let us know in the comments!