How JavaScript Gets Updated: ECMAScript, V8 and Your Runtime Explained
I remember when I started coding in JavaScript, I used to think: "Okay... so JavaScript gets updated like any language... just update and that's it." But that's not exactly how things work here. JavaScript runs in different environments like browser, Node.js, Deno — and what actually decides if you can use that new fancy feature you saw on X (the old Twitter) is your runtime. In this post, I’ll explain how new JavaScript features are released, how ECMAScript fits into this process and why your runtime decides what features you can use (or not). What is ECMAScript? ECMAScript is the official specification of the language and JavaScript is just an implementation of that spec. Every year, a group called TC39 (the committee that defines the language) releases a new ECMAScript version — adding new features, syntax, and behavior. Examples of features introduced by ECMAScript versions: Version Year Features example ES6 2015 let, const, arrow functions, classes ES2020 2020 Optional chaining ?., Nullish coalescing ?? ES2022 2022 #privateFields in classes, top-level await But How Do We Get Those Features? That's the point. It's not about updating JavaScript. It depends on the JavaScript Engine you’re running. What is a JavaScript Engine? A JavaScript Engine is what reads, parses, and executes your JavaScript code. Each environment (browser, Node.js) uses an engine. Popular engines: V8 → used by Chrome and Node.js SpiderMonkey → used by Firefox JavaScriptCore (Nitro) → used by Safari When Do You Get New JavaScript Features? Environment How do you get new features? Browser By updating the browser Node.js By updating your Node.js version It's simple as that. If you want to use new features just need to update the browser or the Node.js version Why Is This Important? Because when you’re writing code locally — in your machine — you can probably use everything that your Node.js or browser supports. But when you're writing code for production (especially frontend), you need to care about your users' environment. Example: If your users still use old browsers like IE11 (hope not) or very old Android versions — you can’t use optional chaining ?. directly. The browser wouldn’t even understand that syntax. That’s why tools like: Babel, TypeScript, Webpack, Vite and others exist — to transpile your code and convert modern JavaScript to old compatible syntax. Final Thoughts JavaScript is updated every year through ECMAScript but you only get those new features when your environment supports them — either by updating the engine (browser, Node.js) or transpiling your code for compatibility. Now every time you see a new JavaScript feature, remember: It’s not about "updating JavaScript" — it’s about your engine. Console You Later!
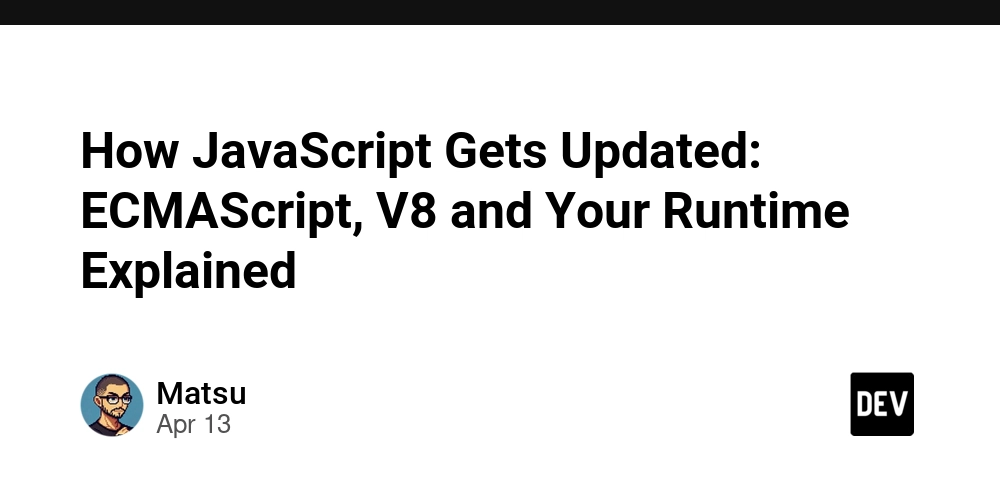
I remember when I started coding in JavaScript, I used to think:
"Okay... so JavaScript gets updated like any language... just update and that's it."
But that's not exactly how things work here. JavaScript runs in different environments like browser, Node.js, Deno — and what actually decides if you can use that new fancy feature you saw on X (the old Twitter) is your runtime.
In this post, I’ll explain how new JavaScript features are released, how ECMAScript fits into this process and why your runtime decides what features you can use (or not).
What is ECMAScript?
ECMAScript is the official specification of the language and JavaScript is just an implementation of that spec.
Every year, a group called TC39 (the committee that defines the language) releases a new ECMAScript version — adding new features, syntax, and behavior.
Examples of features introduced by ECMAScript versions:
Version | Year | Features example |
---|---|---|
ES6 | 2015 |
let , const , arrow functions, classes |
ES2020 | 2020 | Optional chaining ?. , Nullish coalescing ??
|
ES2022 | 2022 |
#privateFields in classes, top-level await
|
But How Do We Get Those Features?
That's the point. It's not about updating JavaScript. It depends on the JavaScript Engine you’re running.
What is a JavaScript Engine?
A JavaScript Engine is what reads, parses, and executes your JavaScript code. Each environment (browser, Node.js) uses an engine.
Popular engines:
- V8 → used by Chrome and Node.js
- SpiderMonkey → used by Firefox
- JavaScriptCore (Nitro) → used by Safari
When Do You Get New JavaScript Features?
Environment | How do you get new features? |
---|---|
Browser | By updating the browser |
Node.js | By updating your Node.js version |
It's simple as that. If you want to use new features just need to update the browser or the Node.js version
Why Is This Important?
Because when you’re writing code locally — in your machine — you can probably use everything that your Node.js or browser supports. But when you're writing code for production (especially frontend), you need to care about your users' environment.
Example:
If your users still use old browsers like IE11 (hope not) or very old Android versions — you can’t use optional chaining ?.
directly.
The browser wouldn’t even understand that syntax.
That’s why tools like: Babel, TypeScript, Webpack, Vite and others exist — to transpile your code and convert modern JavaScript to old compatible syntax.
Final Thoughts
JavaScript is updated every year through ECMAScript but you only get those new features when your environment supports them — either by updating the engine (browser, Node.js) or transpiling your code for compatibility.
Now every time you see a new JavaScript feature, remember:
It’s not about "updating JavaScript" — it’s about your engine.
Console You Later!