Getting Started with Blockchain Application Development
Blockchain technology is transforming industries by providing a secure, transparent, and decentralized way to record transactions. As the demand for blockchain applications increases, developers are presented with new opportunities to create innovative solutions. In this post, we’ll explore the fundamentals of blockchain application development and how you can get started building your own dApps. What is Blockchain? Blockchain is a distributed ledger technology that records transactions across multiple computers in a way that ensures the security and transparency of data. Each block in the chain contains a list of transactions, and once a block is added to the chain, it cannot be altered without consensus from the network. Key Features of Blockchain Decentralization: No central authority; data is distributed across a network of nodes. Transparency: All transactions are visible to participants, ensuring accountability. Security: Cryptographic techniques secure data, making it difficult to tamper with. Immutability: Once recorded, transactions cannot be changed or deleted. Common Blockchain Platforms Ethereum: The most popular platform for building decentralized applications (dApps) using smart contracts. Hyperledger Fabric: A permissioned blockchain framework for enterprise solutions. Binance Smart Chain: A blockchain network running smart contract-based applications. Solana: Known for its high throughput and low transaction costs, suitable for scalable dApps. Cardano: A blockchain platform focused on sustainability and scalability through a layered architecture. Understanding Smart Contracts Smart contracts are self-executing contracts with the terms directly written into code. They automatically execute actions when predefined conditions are met, eliminating the need for intermediaries. Smart contracts are primarily used on platforms like Ethereum. Example of a Simple Smart Contract (Solidity) pragma solidity ^0.8.0; contract SimpleStorage { uint public storedData; function set(uint x) public { storedData = x; } function get() public view returns (uint) { return storedData; } } Building Your First dApp Set Up Your Development Environment: Install Node.js and npm. Use frameworks like Truffle or Hardhat for development. Install a wallet like MetaMask for interacting with the blockchain. Write a Smart Contract: Use Solidity to define the contract's logic. Deploy the Contract: Deploy to a test network (e.g., Rinkeby, Kovan) using tools like Remix. Create a Frontend: Use libraries like web3.js or ethers.js to interact with the smart contract from a web application. Test and Iterate: Test your dApp thoroughly on test networks before deploying to the mainnet. Best Practices for Blockchain Development Write modular, reusable, and easily understandable code for smart contracts. Implement proper error handling and validation to prevent vulnerabilities. Conduct thorough testing, including unit tests and integration tests. Stay updated with the latest security practices and common vulnerabilities (e.g., reentrancy, overflow). Utilize code audit tools to review your smart contracts before deployment. Conclusion Blockchain application development offers exciting opportunities to create decentralized solutions that can change the way we interact and transact online. By understanding the basics of blockchain, smart contracts, and development tools, you can begin your journey into this innovative field. Start small, experiment, and build your way up to more complex dApps!
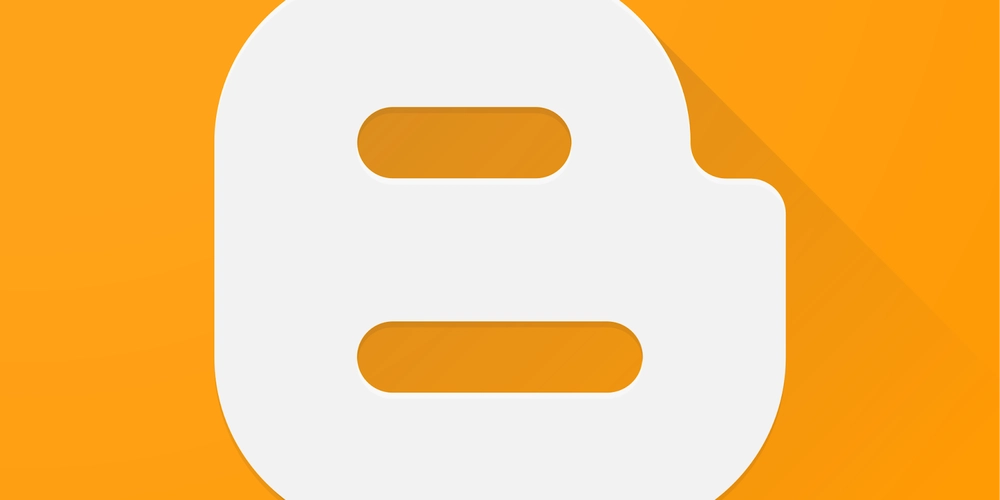
Blockchain technology is transforming industries by providing a secure, transparent, and decentralized way to record transactions. As the demand for blockchain applications increases, developers are presented with new opportunities to create innovative solutions. In this post, we’ll explore the fundamentals of blockchain application development and how you can get started building your own dApps.
What is Blockchain?
Blockchain is a distributed ledger technology that records transactions across multiple computers in a way that ensures the security and transparency of data. Each block in the chain contains a list of transactions, and once a block is added to the chain, it cannot be altered without consensus from the network.
Key Features of Blockchain
- Decentralization: No central authority; data is distributed across a network of nodes.
- Transparency: All transactions are visible to participants, ensuring accountability.
- Security: Cryptographic techniques secure data, making it difficult to tamper with.
- Immutability: Once recorded, transactions cannot be changed or deleted.
Common Blockchain Platforms
- Ethereum: The most popular platform for building decentralized applications (dApps) using smart contracts.
- Hyperledger Fabric: A permissioned blockchain framework for enterprise solutions.
- Binance Smart Chain: A blockchain network running smart contract-based applications.
- Solana: Known for its high throughput and low transaction costs, suitable for scalable dApps.
- Cardano: A blockchain platform focused on sustainability and scalability through a layered architecture.
Understanding Smart Contracts
Smart contracts are self-executing contracts with the terms directly written into code. They automatically execute actions when predefined conditions are met, eliminating the need for intermediaries. Smart contracts are primarily used on platforms like Ethereum.
Example of a Simple Smart Contract (Solidity)
pragma solidity ^0.8.0;
contract SimpleStorage {
uint public storedData;
function set(uint x) public {
storedData = x;
}
function get() public view returns (uint) {
return storedData;
}
}
Building Your First dApp
-
Set Up Your Development Environment:
- Install Node.js and npm.
- Use frameworks like Truffle or Hardhat for development.
- Install a wallet like MetaMask for interacting with the blockchain.
- Write a Smart Contract: Use Solidity to define the contract's logic.
- Deploy the Contract: Deploy to a test network (e.g., Rinkeby, Kovan) using tools like Remix.
- Create a Frontend: Use libraries like web3.js or ethers.js to interact with the smart contract from a web application.
- Test and Iterate: Test your dApp thoroughly on test networks before deploying to the mainnet.
Best Practices for Blockchain Development
- Write modular, reusable, and easily understandable code for smart contracts.
- Implement proper error handling and validation to prevent vulnerabilities.
- Conduct thorough testing, including unit tests and integration tests.
- Stay updated with the latest security practices and common vulnerabilities (e.g., reentrancy, overflow).
- Utilize code audit tools to review your smart contracts before deployment.
Conclusion
Blockchain application development offers exciting opportunities to create decentralized solutions that can change the way we interact and transact online. By understanding the basics of blockchain, smart contracts, and development tools, you can begin your journey into this innovative field. Start small, experiment, and build your way up to more complex dApps!