Get Private Packages in Go
When working with private repositories in Go, fetching dependencies from private GitHub repositories requires some additional configuration. By default, go get expects public repositories, but you can instruct Go to access private ones using the GOPRIVATE environment variable. Steps to Access Private Packages 1. Set the GOPRIVATE Environment Variable This tells Go that the specified repository is private and should not be verified against proxy.golang.org. export GOPRIVATE=github.com/USERNAME/REPOSITORY Alternatively, you can add this permanently to your shell configuration file (e.g., ~/.bashrc, ~/.zshrc): echo 'export GOPRIVATE=github.com/USERNAME/REPOSITORY' >> ~/.bashrc source ~/.bashrc For Windows (PowerShell): [System.Environment]::SetEnvironmentVariable("GOPRIVATE", "github.com/USERNAME/REPOSITORY", [System.EnvironmentVariableTarget]::User) 2. Authenticate GitHub for Private Access If your repository is private, Go needs authentication to fetch it. There are two main ways to authenticate: Using a Personal Access Token (PAT) Generate a GitHub Personal Access Token with repo scope. Use the token in the go get command: git config --global url."https://YOUR_GITHUB_USERNAME:TOKEN@github.com/".insteadOf "https://github.com/" This ensures that when Go attempts to fetch the repository, it includes your credentials. Using SSH Keys If you prefer SSH authentication: Generate an SSH key if you haven't already: ssh-keygen -t rsa -b 4096 -C "your-email@example.com" Add the key to your GitHub account under Settings > SSH and GPG keys. Configure Git to use SSH: git config --global url."git@github.com:".insteadOf "https://github.com/" 3. Fetch the Private Package Now you can run: go get -u github.com/USERNAME/REPOSITORY or, if you need a specific module version: go get github.com/USERNAME/REPOSITORY@v1.2.3 4. Verify the Module To ensure that the module is recognized and properly fetched, run: go list -m all | grep USERNAME/REPOSITORY Conclusion Using private packages in Go requires setting GOPRIVATE, configuring authentication, and ensuring your repository is accessible. Whether using PAT or SSH, these steps help you seamlessly integrate private dependencies into your Go projects.
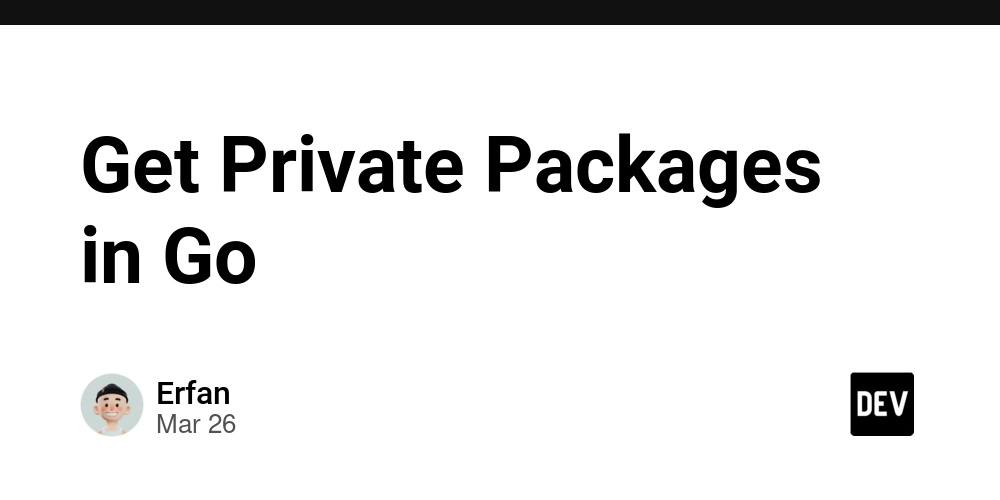
When working with private repositories in Go, fetching dependencies from private GitHub repositories requires some additional configuration. By default, go get
expects public repositories, but you can instruct Go to access private ones using the GOPRIVATE
environment variable.
Steps to Access Private Packages
1. Set the GOPRIVATE
Environment Variable
This tells Go that the specified repository is private and should not be verified against proxy.golang.org
.
export GOPRIVATE=github.com/USERNAME/REPOSITORY
Alternatively, you can add this permanently to your shell configuration file (e.g., ~/.bashrc
, ~/.zshrc
):
echo 'export GOPRIVATE=github.com/USERNAME/REPOSITORY' >> ~/.bashrc
source ~/.bashrc
For Windows (PowerShell):
[System.Environment]::SetEnvironmentVariable("GOPRIVATE", "github.com/USERNAME/REPOSITORY", [System.EnvironmentVariableTarget]::User)
2. Authenticate GitHub for Private Access
If your repository is private, Go needs authentication to fetch it. There are two main ways to authenticate:
Using a Personal Access Token (PAT)
- Generate a GitHub Personal Access Token with
repo
scope. - Use the token in the
go get
command:
git config --global url."https://YOUR_GITHUB_USERNAME:TOKEN@github.com/".insteadOf "https://github.com/"
This ensures that when Go attempts to fetch the repository, it includes your credentials.
Using SSH Keys
If you prefer SSH authentication:
- Generate an SSH key if you haven't already:
ssh-keygen -t rsa -b 4096 -C "your-email@example.com"
- Add the key to your GitHub account under
Settings > SSH and GPG keys
. - Configure Git to use SSH:
git config --global url."git@github.com:".insteadOf "https://github.com/"
3. Fetch the Private Package
Now you can run:
go get -u github.com/USERNAME/REPOSITORY
or, if you need a specific module version:
go get github.com/USERNAME/REPOSITORY@v1.2.3
4. Verify the Module
To ensure that the module is recognized and properly fetched, run:
go list -m all | grep USERNAME/REPOSITORY
Conclusion
Using private packages in Go requires setting GOPRIVATE
, configuring authentication, and ensuring your repository is accessible. Whether using PAT or SSH, these steps help you seamlessly integrate private dependencies into your Go projects.