Enterprise Design Pattern: Transaction Script
Introduction In enterprise applications, organizing business logic clearly and simply is key to creating maintainable systems. One classic pattern for this is the Transaction Script. It structures business logic as a series of procedural scripts, where each script handles a specific transaction or request. Transaction Script is ideal for simple or small systems where the domain logic is not complex. Real-world Analogy Imagine a cashier at a grocery store. Each customer transaction — scanning items, applying discounts, processing payment — is handled step-by-step without involving complex objects or workflows. Similarly, in Transaction Script, we handle each business task step-by-step through a function or procedure. Real-World Example in Code Let's build a simple banking system where users can deposit and withdraw money. We’ll use Python because it's simple to read and write. # transaction_script.py # A simple in-memory 'database' of accounts accounts = { 'alice': 1000, 'bob': 500 } def deposit(account_name, amount): if account_name not in accounts: raise ValueError("Account not found.") if amount
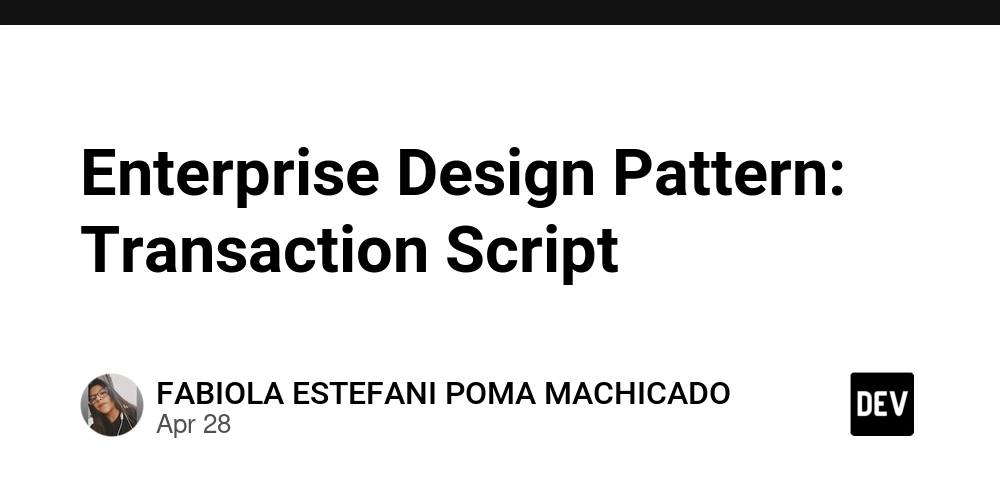
Introduction
In enterprise applications, organizing business logic clearly and simply is key to creating maintainable systems.
One classic pattern for this is the Transaction Script.
It structures business logic as a series of procedural scripts, where each script handles a specific transaction or request.
Transaction Script is ideal for simple or small systems where the domain logic is not complex.
Real-world Analogy
Imagine a cashier at a grocery store.
Each customer transaction — scanning items, applying discounts, processing payment — is handled step-by-step without involving complex objects or workflows.
Similarly, in Transaction Script, we handle each business task step-by-step through a function or procedure.
Real-World Example in Code
Let's build a simple banking system where users can deposit and withdraw money.
We’ll use Python because it's simple to read and write.
# transaction_script.py
# A simple in-memory 'database' of accounts
accounts = {
'alice': 1000,
'bob': 500
}
def deposit(account_name, amount):
if account_name not in accounts:
raise ValueError("Account not found.")
if amount <= 0:
raise ValueError("Amount must be positive.")
accounts[account_name] += amount
print(f"Deposited {amount} to {account_name}. New balance: {accounts[account_name]}")
def withdraw(account_name, amount):
if account_name not in accounts:
raise ValueError("Account not found.")
if amount <= 0:
raise ValueError("Amount must be positive.")
if accounts[account_name] < amount:
raise ValueError("Insufficient funds.")
accounts[account_name] -= amount
print(f"Withdrew {amount} from {account_name}. New balance: {accounts[account_name]}")
def main():
deposit('alice', 200)
withdraw('bob', 100)
if __name__ == "__main__":
main()
Explanation
Each function (deposit, withdraw) is a transaction script that:
Validates input
Updates the "database" (dictionary accounts)
Prints the result
There's no need for complex classes or objects.
Each business operation is directly coded as a simple procedure.
Conclusion
Transaction Script is a great pattern when:
- You have straightforward logic.
- You want fast, simple development.
- The system is small or not very complicated.
For bigger or more complex applications, you might eventually move to a more structured approach like Domain Model.
You can find the full code for this article in my GitHub repository: https://github.com/fabipm/Research02_SI889_U1_Poma.git