DSA Made Easy: Master These 6 Math Essentials
The Most Important Math Topics for DSA: A Software Engineer’s Guide to Mastering Algorithms Hey there, fellow coder! If you’re diving into the world of Data Structures and Algorithms (DSA), you’ve probably realized that it’s not just about writing code—it’s about thinking smart. And guess what? Math is your secret weapon. I’ve been a software engineer for years, grinding through coding interviews, debugging late into the night, and building systems that hum like a well-tuned engine. Along the way, I’ve learned that a solid grip on certain math topics can make DSA feel less like a maze and more like a treasure map. So, let’s break it down together! In this article, I’ll walk you through the most important math topics for DSA, explain why they matter, and show you how they pop up in real coding challenges. Whether you’re prepping for a Big Tech interview or just want to level up your problem-solving skills, this guide is for you. Let’s get started! Why Math Matters in DSA Before we jump into the juicy stuff, let’s clear the air: you don’t need to be a math genius to ace DSA. Trust me, I’m no Pythagoras. But understanding key math concepts helps you see patterns, optimize solutions, and tackle problems with confidence. Think of it like this: math is the foundation, data structures are the tools, and algorithms are the blueprints. Nail the foundation, and the rest falls into place. Now, here are the math topics that’ll give you an edge in DSA—explained in a way that won’t make your brain hurt. 1. Discrete Mathematics: The DSA Backbone If there’s one math topic that screams “DSA,” it’s discrete mathematics. This isn’t the calculus you might’ve dreaded in school—it’s all about discrete (separate, countable) things like numbers, sets, and graphs. Why does it matter? Because computers deal with discrete data, and DSA is all about manipulating it efficiently. Key Concepts: Sets and Relations: Ever wondered how hash tables work? Sets are the idea behind them—unique elements, and fast lookups. Relations help you understand connections, like in graph algorithms. Logic: Writing a tricky if-else condition? Logic ensures your code makes sense and avoids infinite loops. Combinatorics: Counting possibilities—like how many ways can you arrange nodes in a tree—helps with algorithm design. Real-World DSA Example: Imagine you’re solving a problem like “Find all subsets of a set” (a classic recursion challenge). Combinatorics tells you there are 2ⁿ subsets for a set of size n. That’s your starting point for coding an efficient solution! 2. Number Theory: Cracking the Code Number theory studies integers and their properties—think prime numbers, divisors, and modular arithmetic. It’s like the detective work of math, and it shows everywhere in DSA. Key Concepts: Prime Numbers: Used in hashing algorithms and cryptography-inspired problems. GCD and LCM: Greatest Common Divisor and Least Common Multiple are clutch for optimizing loops and finding patterns. Modular Arithmetic: Ever seen “return result % 1000000007” in a problem? That’s modular arithmetic keeping numbers manageable. Real-World DSA Example: Take the “Two Sum” problem: given an array and a target, find two numbers that add up to it. Knowing divisibility and modular properties can help you optimize your approach beyond a brute-force O(n²) solution to a slick O(n) hash map method. 3. Algebra: Solving the Puzzle Algebra isn’t just about solving for x—it’s about manipulating equations and understanding relationships. In DSA, it’s your go-to for simplifying problems and spotting shortcuts. Key Concepts: Equations and Inequalities: Balance time and space complexity like an equation—trade-offs are everything. Exponents and Logarithms: Big O notation (like O(n log n)) is rooted here. Understanding logs helps you grok why binary search is so fast. Sequences: Arithmetic and geometric progressions pop up in array problems and dynamic programming. Real-World DSA Example: Ever tackled a binary search problem? The reason it’s O(log n) is because each step cuts your search space in half—pure logarithmic magic. Algebra lets you prove it and feel like a wizard. 4. Probability and Statistics: The Luck of the Draw Okay, hear me out—probability isn’t just for gamblers. In DSA, it’s about making smart guesses and handling randomness, especially in algorithms like QuickSort or machine learning prep. Key Concepts: Basic Probability: What’s the chance this element is in the right spot? Useful for randomized algorithms. Expected Value: Helps you analyze average-case performance. Permutations and Combinations: Count ways to arrange or select items—like in graph traversals or string problems. Real-World DSA Example: In QuickSort, picking a pivot randomly uses probability to avoid worst-case O(n²) scenarios. Knowing this can make a shaky algorithm reliable. 5. Graph Theor
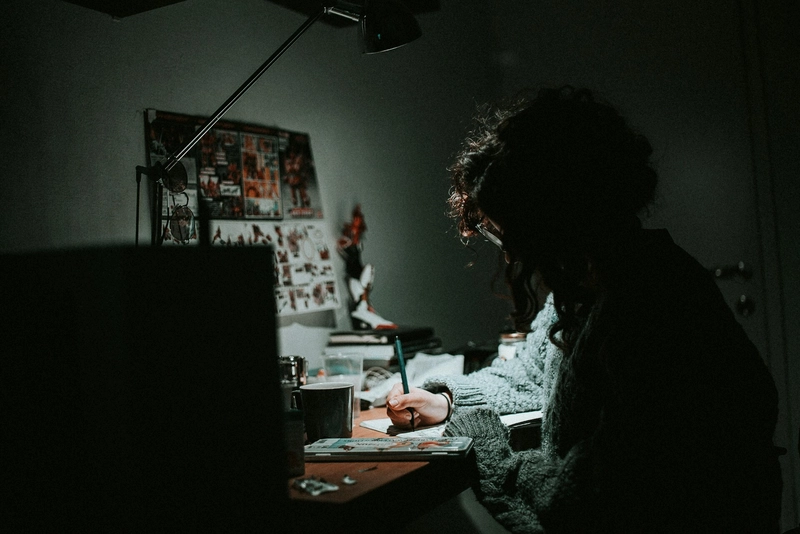
The Most Important Math Topics for DSA: A Software Engineer’s Guide to Mastering Algorithms
Hey there, fellow coder! If you’re diving into the world of Data Structures and Algorithms (DSA), you’ve probably realized that it’s not just about writing code—it’s about thinking smart. And guess what? Math is your secret weapon. I’ve been a software engineer for years, grinding through coding interviews, debugging late into the night, and building systems that hum like a well-tuned engine. Along the way, I’ve learned that a solid grip on certain math topics can make DSA feel less like a maze and more like a treasure map.
So, let’s break it down together! In this article, I’ll walk you through the most important math topics for DSA, explain why they matter, and show you how they pop up in real coding challenges. Whether you’re prepping for a Big Tech interview or just want to level up your problem-solving skills, this guide is for you. Let’s get started!
Why Math Matters in DSA
Before we jump into the juicy stuff, let’s clear the air: you don’t need to be a math genius to ace DSA. Trust me, I’m no Pythagoras. But understanding key math concepts helps you see patterns, optimize solutions, and tackle problems with confidence. Think of it like this: math is the foundation, data structures are the tools, and algorithms are the blueprints. Nail the foundation, and the rest falls into place.
Now, here are the math topics that’ll give you an edge in DSA—explained in a way that won’t make your brain hurt.
1. Discrete Mathematics: The DSA Backbone
If there’s one math topic that screams “DSA,” it’s discrete mathematics. This isn’t the calculus you might’ve dreaded in school—it’s all about discrete (separate, countable) things like numbers, sets, and graphs. Why does it matter? Because computers deal with discrete data, and DSA is all about manipulating it efficiently.
Key Concepts:
- Sets and Relations: Ever wondered how hash tables work? Sets are the idea behind them—unique elements, and fast lookups. Relations help you understand connections, like in graph algorithms.
- Logic: Writing a tricky if-else condition? Logic ensures your code makes sense and avoids infinite loops.
- Combinatorics: Counting possibilities—like how many ways can you arrange nodes in a tree—helps with algorithm design.
Real-World DSA Example:
Imagine you’re solving a problem like “Find all subsets of a set” (a classic recursion challenge). Combinatorics tells you there are 2ⁿ subsets for a set of size n. That’s your starting point for coding an efficient solution!
2. Number Theory: Cracking the Code
Number theory studies integers and their properties—think prime numbers, divisors, and modular arithmetic. It’s like the detective work of math, and it shows everywhere in DSA.
Key Concepts:
- Prime Numbers: Used in hashing algorithms and cryptography-inspired problems.
- GCD and LCM: Greatest Common Divisor and Least Common Multiple are clutch for optimizing loops and finding patterns.
- Modular Arithmetic: Ever seen “return result % 1000000007” in a problem? That’s modular arithmetic keeping numbers manageable.
Real-World DSA Example:
Take the “Two Sum” problem: given an array and a target, find two numbers that add up to it. Knowing divisibility and modular properties can help you optimize your approach beyond a brute-force O(n²) solution to a slick O(n) hash map method.
3. Algebra: Solving the Puzzle
Algebra isn’t just about solving for x—it’s about manipulating equations and understanding relationships. In DSA, it’s your go-to for simplifying problems and spotting shortcuts.
Key Concepts:
- Equations and Inequalities: Balance time and space complexity like an equation—trade-offs are everything.
- Exponents and Logarithms: Big O notation (like O(n log n)) is rooted here. Understanding logs helps you grok why binary search is so fast.
- Sequences: Arithmetic and geometric progressions pop up in array problems and dynamic programming.
Real-World DSA Example:
Ever tackled a binary search problem? The reason it’s O(log n) is because each step cuts your search space in half—pure logarithmic magic. Algebra lets you prove it and feel like a wizard.
4. Probability and Statistics: The Luck of the Draw
Okay, hear me out—probability isn’t just for gamblers. In DSA, it’s about making smart guesses and handling randomness, especially in algorithms like QuickSort or machine learning prep.
Key Concepts:
- Basic Probability: What’s the chance this element is in the right spot? Useful for randomized algorithms.
- Expected Value: Helps you analyze average-case performance.
- Permutations and Combinations: Count ways to arrange or select items—like in graph traversals or string problems.
Real-World DSA Example:
In QuickSort, picking a pivot randomly uses probability to avoid worst-case O(n²) scenarios. Knowing this can make a shaky algorithm reliable.
5. Graph Theory: Connecting the Dots
Graphs are the rockstars of DSA—think social networks, maps, or dependency trees. Graph theory is the math behind them, and it’s a goldmine for problem-solving.
Key Concepts:
- Vertices and Edges: The building blocks of graphs.
- Paths and Cycles: Shortest path (Dijkstra’s) or detecting cycles (DFS) rely on these.
- Trees: A special graph type that’s everywhere—binary trees, heaps, you name it.
Real-World DSA Example:
Solving “Number of Islands” (a grid-based problem) is all about graph traversal. Flood fill with DFS or BFS? Graph theory guides you to the answer.
6. Recurrence Relations: The Art of Breaking It Down
This one’s a bit sneaky but oh-so-powerful. Recurrence relations describe how problems break into smaller subproblems—like in recursion or dynamic programming (DP).
Key Concepts:
- Base Cases: Where recursion stops.
- Recurrence Equations: Think T(n) = T(n-1) + 1 for a simple loop, or T(n) = 2T(n/2) + n for merge sort.
- Master Theorem: A cheat code to analyze time complexity of divide-and-conquer algorithms.
Real-World DSA Example:
Fibonacci with DP—without recurrence, you’re stuck with exponential time. Memoize it, and boom, it’s O(n). That’s recurrence relations saving the day.
How to Learn These Topics (Without Losing Your Mind)
I get it—math can feel overwhelming. But here’s how I tackled it, and you can too:
- Start Small: Pick one topic (say, modular arithmetic) and solve 5-10 problems on it.
- Code It Out: Math clicks when you see it in action. Write a program to find GCD or traverse a graph.
- Use Visuals: Sketch a tree or graph. Seeing is believing.
- Practice on Platforms: LeetCode, HackerRank, and Codeforces are your playgrounds. Filter by topic and grind.
Final Thoughts: Math + DSA = Your Superpower
As a software engineer, I’ve seen firsthand how these math topics turn “ugh, another problem” into “I’ve got this.” Discrete math gives you structure, number theory sharpens your tools, algebra speeds you up, probability keeps you flexible, graph theory maps the terrain, and recurrence relations tie it all together. You don’t need to master everything overnight—just build a little each day.
So, next time you’re staring down a DSA problem, remember: you’re not just coding—you’re wielding math like a pro. Got a favourite topic or a killer problem you’ve solved with these? Drop it in the comments—I’d love to geek out with you!
Happy coding,
Shubhajit Paul