Day 8 String & Return Datatype
String & Return Data Type A string is a fundamental data type in programming that represents a sequence of characters. Strings are used to store and manipulate text-based data. Characteristics of Strings: Enclosed in quotes (single ' ', double " ", or triple ''' '''/""" """) Immutable in many languages (cannot be changed after creation) Can contain letters, numbers, symbols, and whitespace Have a length property (number of characters) Examples in Different Languages: Python: message = "Hello, World!" multi_line = """This is a multi-line string""" JavaScript: let name = 'Alice'; let greeting = `Hello, ${name}!`; // Template literal Java: String s = "Java Strings"; C: char str[] = "C string"; // Array of characters Common String Operations: Concatenation (joining strings) Substring extraction Searching within strings Case conversion (upper/lower) Splitting and joining Formatting Strings are one of the most commonly used data types in programming, essential for everything from simple messages to complex text processing. Return Data Types in Java (In-Depth) In Java, every method (except constructors and void methods) must specify a return type, which defines the type of value the method returns when it is called. The return type is declared before the method name in the method signature. 1. Primitive Return Types Java has 8 primitive data types, and methods can return any of them: a) int (Integer) public int add(int a, int b) { return a + b; } Returns a 32-bit signed integer. b) long (Long Integer) public long getBigNumber() { return 10000000000L; // 'L' suffix for long } Returns a 64-bit signed integer. c) float (Floating-Point) public float divide(float a, float b) { return a / b; } Returns a 32-bit floating-point number. d) double (Double-Precision Float) public double computeSquareRoot(double num) { return Math.sqrt(num); } Returns a 64-bit floating-point number. e) boolean (True/False) public boolean isEven(int num) { return num % 2 == 0; } Returns true or false. f) char (Single Character) public char getFirstChar(String str) { return str.charAt(0); } Returns a single Unicode character. g) byte (8-bit Integer) public byte getByteValue() { return 127; // Max value for byte } Returns an 8-bit signed integer. h) short (16-bit Integer) public short getShortValue() { return 32000; } Returns a 16-bit signed integer. 2. Non-Primitive (Reference) Return Types Methods can also return objects, arrays, or String. a) String public String greet(String name) { return "Hello, " + name; } Returns a String object. b) Array Return public int[] getNumbers() { return new int[]{1, 2, 3}; } Returns an array of integers. c) Custom Class/Object Return public Person createPerson(String name, int age) { return new Person(name, age); } Returns an instance of a user-defined class. d) List, Set, Map (Collections) public List getNames() { return Arrays.asList("Alice", "Bob", "Charlie"); } Returns a collection. 3. Special Return Types a) void (No Return) public void printMessage(String msg) { System.out.println(msg); // No return statement needed } Used when a method does not return any value. b) Returning null public String findName(int id) { if (id == 1) return "Alice"; else return null; // No name found } Indicates "no value" for reference types. c) Returning this (Fluent APIs) public class Calculator { private int result; public Calculator add(int num) { this.result += num; return this; // Returns the current object } } Useful for method chaining. 4. Best Practices for Return Types in Java Be Consistent: If a method returns a collection, prefer returning an empty collection (Collections.emptyList()) instead of null. Use Optional (Java 8+) for methods that may not return a value: public Optional findUser(int id) { if (userExists(id)) return Optional.of("Alice"); else return Optional.empty(); } Avoid Returning Mutable Objects (unless intentional) to prevent unwanted modifications. Document Return Types using JavaDoc: /** * @return the sum of two numbers */ public int add(int a, int b) { ... } Conclusion Java enforces strict return type declarations, which helps in: Type Safety: Ensures methods return the expected data type. Readability: Makes code easier to understand. Compile-Time Checks: Prevents runtime errors due to incorrect return types. Understanding return types is cruci
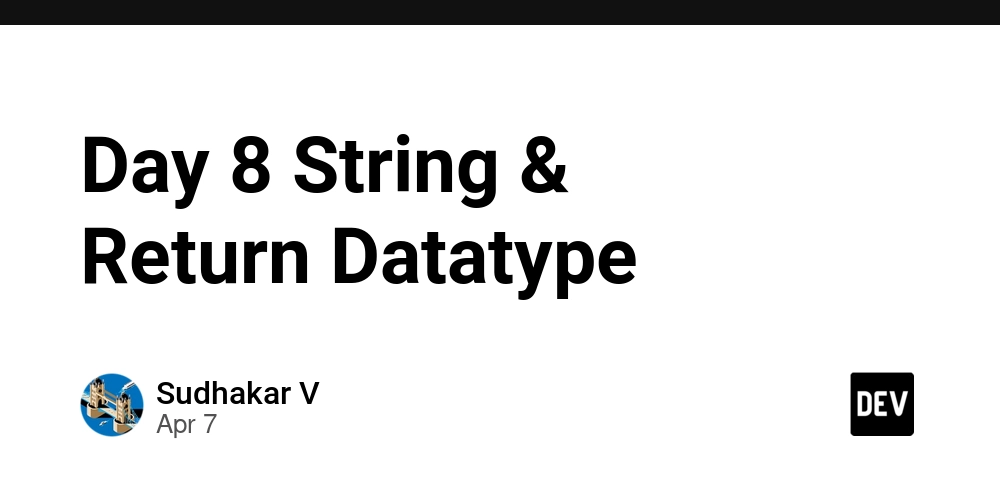
String & Return Data Type
A string is a fundamental data type in programming that represents a sequence of characters. Strings are used to store and manipulate text-based data.
Characteristics of Strings:
- Enclosed in quotes (single
' '
, double" "
, or triple''' '''
/""" """
) - Immutable in many languages (cannot be changed after creation)
- Can contain letters, numbers, symbols, and whitespace
- Have a length property (number of characters)
Examples in Different Languages:
Python:
message = "Hello, World!"
multi_line = """This is a
multi-line string"""
JavaScript:
let name = 'Alice';
let greeting = `Hello, ${name}!`; // Template literal
Java:
String s = "Java Strings";
C:
char str[] = "C string"; // Array of characters
Common String Operations:
- Concatenation (joining strings)
- Substring extraction
- Searching within strings
- Case conversion (upper/lower)
- Splitting and joining
- Formatting
Strings are one of the most commonly used data types in programming, essential for everything from simple messages to complex text processing.
Return Data Types in Java (In-Depth)
In Java, every method (except constructors and void methods) must specify a return type, which defines the type of value the method returns when it is called. The return type is declared before the method name in the method signature.
1. Primitive Return Types
Java has 8 primitive data types, and methods can return any of them:
a) int
(Integer)
public int add(int a, int b) {
return a + b;
}
- Returns a 32-bit signed integer.
b) long
(Long Integer)
public long getBigNumber() {
return 10000000000L; // 'L' suffix for long
}
- Returns a 64-bit signed integer.
c) float
(Floating-Point)
public float divide(float a, float b) {
return a / b;
}
- Returns a 32-bit floating-point number.
d) double
(Double-Precision Float)
public double computeSquareRoot(double num) {
return Math.sqrt(num);
}
- Returns a 64-bit floating-point number.
e) boolean
(True/False)
public boolean isEven(int num) {
return num % 2 == 0;
}
- Returns
true
orfalse
.
f) char
(Single Character)
public char getFirstChar(String str) {
return str.charAt(0);
}
- Returns a single Unicode character.
g) byte
(8-bit Integer)
public byte getByteValue() {
return 127; // Max value for byte
}
- Returns an 8-bit signed integer.
h) short
(16-bit Integer)
public short getShortValue() {
return 32000;
}
- Returns a 16-bit signed integer.
2. Non-Primitive (Reference) Return Types
Methods can also return objects, arrays, or String
.
a) String
public String greet(String name) {
return "Hello, " + name;
}
- Returns a
String
object.
b) Array Return
public int[] getNumbers() {
return new int[]{1, 2, 3};
}
- Returns an array of integers.
c) Custom Class/Object Return
public Person createPerson(String name, int age) {
return new Person(name, age);
}
- Returns an instance of a user-defined class.
d) List
, Set
, Map
(Collections)
public List<String> getNames() {
return Arrays.asList("Alice", "Bob", "Charlie");
}
- Returns a collection.
3. Special Return Types
a) void
(No Return)
public void printMessage(String msg) {
System.out.println(msg);
// No return statement needed
}
- Used when a method does not return any value.
b) Returning null
public String findName(int id) {
if (id == 1) return "Alice";
else return null; // No name found
}
- Indicates "no value" for reference types.
c) Returning this
(Fluent APIs)
public class Calculator {
private int result;
public Calculator add(int num) {
this.result += num;
return this; // Returns the current object
}
}
- Useful for method chaining.
4. Best Practices for Return Types in Java
-
Be Consistent: If a method returns a collection, prefer returning an empty collection (
Collections.emptyList()
) instead ofnull
. -
Use
Optional
(Java 8+) for methods that may not return a value:
public Optional<String> findUser(int id) {
if (userExists(id)) return Optional.of("Alice");
else return Optional.empty();
}
- Avoid Returning Mutable Objects (unless intentional) to prevent unwanted modifications.
- Document Return Types using JavaDoc:
/**
* @return the sum of two numbers
*/
public int add(int a, int b) { ... }
Conclusion
Java enforces strict return type declarations, which helps in:
- Type Safety: Ensures methods return the expected data type.
- Readability: Makes code easier to understand.
- Compile-Time Checks: Prevents runtime errors due to incorrect return types.
Understanding return types is crucial for writing correct and maintainable Java code.