cPanel MySQL database remotely from your local VS Code
✅ Step 1: Allow Remote MySQL Access in cPanel Login to your cPanel. Go to Remote MySQL under the Databases section. Add your local machine’s IP address (you can find it here). Example: 123.45.67.89 You can also use % to allow access from all IPs (not secure in production). Click Add Host. ✅ Step 2: Find Database Connection Info in cPanel In your cPanel, go to MySQL Databases and note: Database name Database username Password Hostname (might be yourdomain.com, localhost, or a remote IP like 123.45.67.89) ⚠️ Important: localhost won’t work from VS Code, because it points to your local machine. You need to use the public hostname or IP address of your server (e.g., server123.webhost.com or 203.0.113.7). ✅ Step 3: Update Your Code in VS Code import mysql from "mysql2/promise"; const db = mysql.createPool({ host: "your-hostname-or-ip", // e.g., "203.0.113.7" or "server123.webhost.com" user: "your_cpanel_db_user", // e.g., "yourcpaneluser_dbuser" password: "your_db_password", database: "yourcpanel_db_name", // e.g., "yourcpaneluser_dbname" waitForConnections: true, connectionLimit: 10, queueLimit: 0, }); export default db; ✅ Step 4: Allow Port 3306 (If Needed) If you’re using a firewall or your host blocks incoming MySQL connections: Make sure port 3306 (MySQL default) is open. Contact your hosting support if unsure.
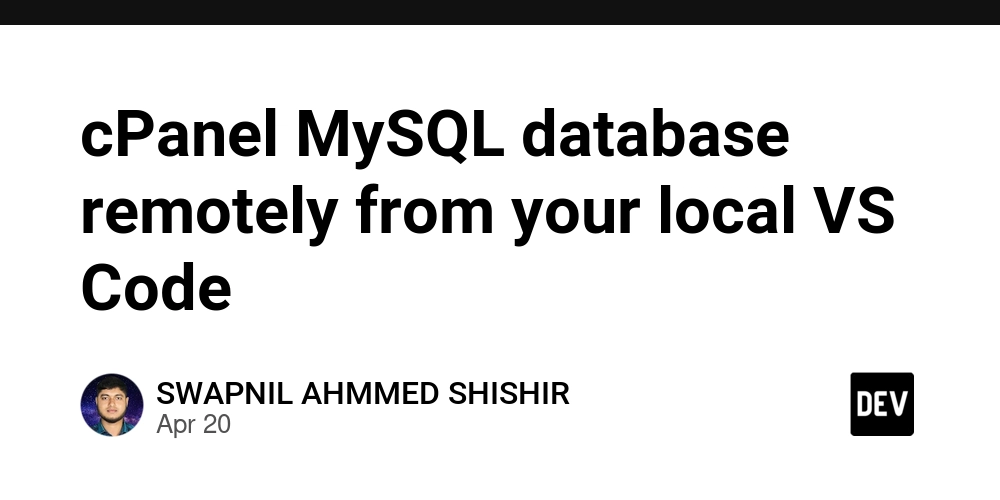
✅ Step 1: Allow Remote MySQL Access in cPanel
- Login to your cPanel.
- Go to Remote MySQL under the Databases section.
- Add your local machine’s IP address (you can find it here).
- Example:
123.45.67.89
- You can also use
%
to allow access from all IPs (not secure in production).
- Example:
- Click Add Host.
✅ Step 2: Find Database Connection Info in cPanel
In your cPanel, go to MySQL Databases and note:
- Database name
- Database username
- Password
-
Hostname (might be
yourdomain.com
,localhost
, or a remote IP like123.45.67.89
)
⚠️ Important:
localhost
won’t work from VS Code, because it points to your local machine. You need to use the public hostname or IP address of your server (e.g.,server123.webhost.com
or203.0.113.7
).
✅ Step 3: Update Your Code in VS Code
import mysql from "mysql2/promise";
const db = mysql.createPool({
host: "your-hostname-or-ip", // e.g., "203.0.113.7" or "server123.webhost.com"
user: "your_cpanel_db_user", // e.g., "yourcpaneluser_dbuser"
password: "your_db_password",
database: "yourcpanel_db_name", // e.g., "yourcpaneluser_dbname"
waitForConnections: true,
connectionLimit: 10,
queueLimit: 0,
});
export default db;
✅ Step 4: Allow Port 3306 (If Needed)
If you’re using a firewall or your host blocks incoming MySQL connections:
- Make sure port 3306 (MySQL default) is open.
- Contact your hosting support if unsure.