Chatbot Application: Comprehensive Documentation
1. Introduction This documentation provides a step-by-step guide for setting up, developing, and deploying a chatbot application using Next.js, Tailwind CSS, and OpenAI's API via OpenRouter. This guide is designed for beginners who are new to Next.js and will cover everything from project setup to API key retrieval and implementation. 2. Tech Stack Core Technologies: Next.js: A React framework for building server-rendered and statically generated web applications. Tailwind CSS: A utility-first CSS framework for styling the application. OpenAI API (via OpenRouter): Provides AI-generated responses by accessing multiple AI models, including DeepSeek. Lucide-react: A collection of beautiful, consistent icons. Shadcn: TailwindCSS UI Library 3. Project Setup Step 1: Initialize a Next.js Project npx create-next-app@latest Continue to name the application and select the app setup options Step 2: Install Dependencies npm install openai lucide-react npx shadcn@latest init npx shadcn@latest add button npx shadcn@latest add input npx shadcn@latest add card Step 3: Set Up Environment Variables Create a .env.local file in the root directory: Content: NEXT_PUBLIC_OPENROUTER_API_KEY=your_api_key_here 4. Obtaining OpenAI API Key from OpenRouter Visit OpenRouter. Sign up or log in. Navigate to the API keys section. Generate a new API key. Copy the key and paste it into your .env.local file. (Refer to project structure to see where to create this file) 5. Project Structure chatbot_1/ ├── .env.local ├── app/ │ ├── api/ │ │ └── chat/ │ │ └── route.ts # API route for handling chat requests │ ├── globals.css # Global CSS styles │ ├── layout.tsx # Root layout component │ └── page.tsx # Main page component (Chatbot UI) ├── components/ │ ├── Header.tsx # Header component │ ├── Spinner.tsx # Loading spinner │ ├── ui/ # Reusable UI components │ │ ├── button.tsx │ │ ├── card.tsx │ │ └── input.tsx ├── lib/ │ └── utils.ts # Utility functions ├── next.config.ts # Next.js configuration ├── package.json # Project dependencies ├── tailwind.config.ts # Tailwind CSS configuration ├── tsconfig.json # TypeScript configuration └── README.md # Project documentation 6. Implementing the Chatbot API Route app/api/chat/route.ts Section 1: Importing required modules import { NextRequest, NextResponse } from "next/server"; import OpenAI from "openai"; The NextRequest and NextResponse classes are imported from next/server, allowing the function to handle incoming HTTP requests and send responses in a Next.js API route. The OpenAI library is imported to interact with OpenAI’s API, enabling the chatbot to generate responses. Section 2: Defining your system prompt const systemPrompt = `You are a highly reliable AI job search assistant.` This system prompt is a predefined message that guides the AI model's behavior. It ensures the chatbot consistently provides job-related advice rather than random responses. Section 3: Defining the API Handler Function export async function POST(req: NextRequest) { //Initializing the OpenAI API Client //This block initializes an OpenAI client with a base API URL (openrouter.ai), which is an alternative API provider for OpenAI models. The apiKey is retrieved from environment variables to keep it secure. defaultHeaders include: "HTTP-Referer": Specifies the origin of the request (helps with authentication and security). "X-Title": A custom title for the API request, possibly used for tracking purposes. const openai = new OpenAI({ baseURL: "https://openrouter.ai/api/v1", apiKey: process.env.NEXT_PUBLIC_OPENROUTER_API_KEY, defaultHeaders: { "HTTP-Referer": "http://localhost:3000", "X-Title": "Headstarter Gh", }, }); //Extracting and Formatting the Request Data const data = await req.json(); const messages = Array.isArray(data) ? data : [data]; //Sending Data to OpenAI for a Chatbot Response const completion = await openai.chat.completions.create({ messages: [{ role: "system", content: systemPrompt }, ...messages], model: "deepseek/deepseek-chat:free",//model name from Openrouter stream: true, }); //Handling the Streamed Response let accumulatedResponse = ""; try { //Handling the Streamed Response for await (const chunk of completion) { const content = chunk.choices[0]?.delta?.content; if (content) { accumulatedResponse += content; } } } catch (error) { //Handling errors console.error("Error:", error); return new NextResponse("Internal Server Error", { status: 500 }); } //Sending the Final Response Back to the Client return new NextResponse(accumulatedResponse); } Final Summary This API route: Accepts user messages via a POST request. Initializes an OpenAI client with API credentials
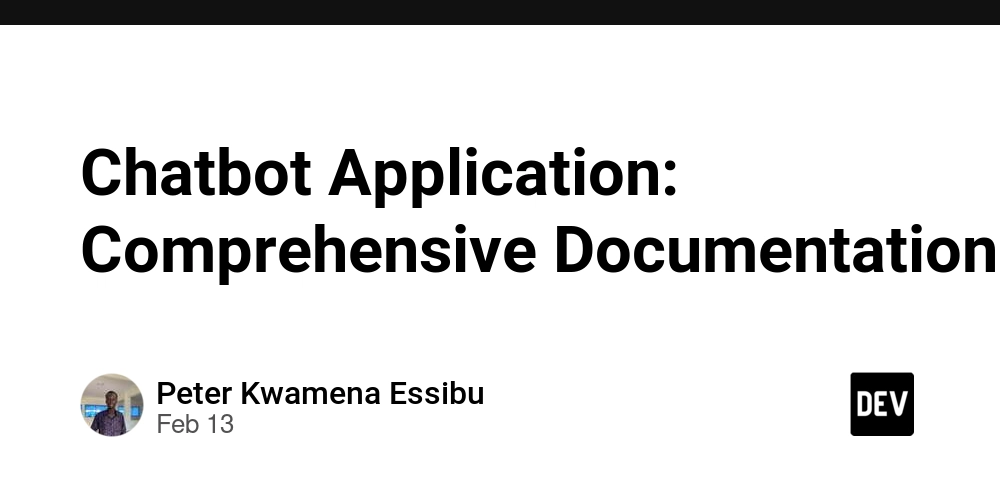
1. Introduction
This documentation provides a step-by-step guide for setting up, developing, and deploying a chatbot application using Next.js, Tailwind CSS, and OpenAI's API via OpenRouter. This guide is designed for beginners who are new to Next.js and will cover everything from project setup to API key retrieval and implementation.
2. Tech Stack
Core Technologies:
- Next.js: A React framework for building server-rendered and statically generated web applications.
- Tailwind CSS: A utility-first CSS framework for styling the application.
- OpenAI API (via OpenRouter): Provides AI-generated responses by accessing multiple AI models, including DeepSeek.
- Lucide-react: A collection of beautiful, consistent icons.
- Shadcn: TailwindCSS UI Library
3. Project Setup
Step 1: Initialize a Next.js Project
- npx create-next-app@latest
- Continue to name the application and select the app setup options
Step 2: Install Dependencies
- npm install openai lucide-react
- npx shadcn@latest init
- npx shadcn@latest add button
- npx shadcn@latest add input
- npx shadcn@latest add card
Step 3: Set Up Environment Variables
- Create a .env.local file in the root directory: Content: NEXT_PUBLIC_OPENROUTER_API_KEY=your_api_key_here
4. Obtaining OpenAI API Key from OpenRouter
- Visit OpenRouter.
- Sign up or log in.
- Navigate to the API keys section.
- Generate a new API key.
- Copy the key and paste it into your .env.local file. (Refer to project structure to see where to create this file)
5. Project Structure
chatbot_1/
├── .env.local
├── app/
│ ├── api/
│ │ └── chat/
│ │ └── route.ts # API route for handling chat requests
│ ├── globals.css # Global CSS styles
│ ├── layout.tsx # Root layout component
│ └── page.tsx # Main page component (Chatbot UI)
├── components/
│ ├── Header.tsx # Header component
│ ├── Spinner.tsx # Loading spinner
│ ├── ui/ # Reusable UI components
│ │ ├── button.tsx
│ │ ├── card.tsx
│ │ └── input.tsx
├── lib/
│ └── utils.ts # Utility functions
├── next.config.ts # Next.js configuration
├── package.json # Project dependencies
├── tailwind.config.ts # Tailwind CSS configuration
├── tsconfig.json # TypeScript configuration
└── README.md # Project documentation
6. Implementing the Chatbot API Route
app/api/chat/route.ts
Section 1: Importing required modules
import { NextRequest, NextResponse } from "next/server";
import OpenAI from "openai";
- The NextRequest and NextResponse classes are imported from next/server, allowing the function to handle incoming HTTP requests and send responses in a Next.js API route.
- The OpenAI library is imported to interact with OpenAI’s API, enabling the chatbot to generate responses.
Section 2: Defining your system prompt
const systemPrompt = `You are a highly reliable AI job search assistant.`
This system prompt is a predefined message that guides the AI model's behavior.
It ensures the chatbot consistently provides job-related advice rather than random responses.
Section 3: Defining the API Handler Function
export async function POST(req: NextRequest) {
//Initializing the OpenAI API Client
//This block initializes an OpenAI client with a base API URL (openrouter.ai), which is an alternative API provider for OpenAI models.
The apiKey is retrieved from environment variables to keep it secure.
defaultHeaders include:
"HTTP-Referer": Specifies the origin of the request (helps with authentication and security).
"X-Title": A custom title for the API request, possibly used for tracking purposes.
const openai = new OpenAI({
baseURL: "https://openrouter.ai/api/v1",
apiKey: process.env.NEXT_PUBLIC_OPENROUTER_API_KEY,
defaultHeaders: {
"HTTP-Referer": "http://localhost:3000",
"X-Title": "Headstarter Gh",
},
});
//Extracting and Formatting the Request Data
const data = await req.json();
const messages = Array.isArray(data) ? data : [data];
//Sending Data to OpenAI for a Chatbot Response
const completion = await openai.chat.completions.create({
messages: [{ role: "system", content: systemPrompt }, ...messages],
model: "deepseek/deepseek-chat:free",//model name from Openrouter
stream: true,
});
//Handling the Streamed Response
let accumulatedResponse = "";
try {
//Handling the Streamed Response
for await (const chunk of completion) {
const content = chunk.choices[0]?.delta?.content;
if (content) {
accumulatedResponse += content;
}
}
} catch (error) {
//Handling errors
console.error("Error:", error);
return new NextResponse("Internal Server Error", { status: 500 });
}
//Sending the Final Response Back to the Client
return new NextResponse(accumulatedResponse);
}
Final Summary
This API route:
- Accepts user messages via a POST request.
- Initializes an OpenAI client with API credentials.
- Processes incoming messages and formats them correctly.
- Sends the messages to OpenAI along with a system prompt.
- Streams the response back to the client piece by piece.
- Returns the AI-generated response to the frontend chatbot.
- Handles any errors and returns an appropriate status code if something goes wrong.
7. Creating the Chatbot UI
app/page.js
Section 1
Importing components and react hooks initialization
"use client";
import { useState } from "react";
import { Button } from "@/components/ui/button";
import { Input } from "@/components/ui/input";
import {
Card,
CardContent,
CardHeader,
CardTitle,
CardFooter,
} from "@/components/ui/card";
import { Spinner } from "../components/Spinner";
import Header from "../components/Header";
Section 2
- This React component, ChatBot, defines a simple chatbot interface using the useState hook to manage state.
- The messages state holds the conversation history, while input stores the current user input.
- isTyping is a boolean state that indicates whether the chatbot is currently generating a response.
- The handleInputChange function updates the input state whenever the user types in the chat input field.
- When the form is submitted, handleSubmit prevents the default form behavior and sets isTyping to true.
- A new message from the user is added to the messages state, and a request is sent to /api/chat with this message.
- The response from the server is streamed, meaning the chatbot’s response arrives in chunks instead of all at once.
- Using a TextDecoder, the component decodes these chunks and updates messages dynamically as data arrives.
- If an error occurs during the fetch request, it is caught and logged to the console.
- Finally, isTyping is set to false, and input is cleared, resetting the chat input field for the next message.
export default function ChatBot() {
const [messages, setMessages] = useState([]);
const [input, setInput] = useState("");
const [isTyping, setIsTyping] = useState(false);
const handleInputChange = (e) => {
setInput(e.target.value);
};
const handleSubmit = async (e) => {
e.preventDefault();
setIsTyping(true);
const newMessage = { role: "user", content: input };
setMessages([...messages, newMessage]);
try {
const response = await fetch("/api/chat", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify(newMessage),
});
const reader = response.body.getReader();
const decoder = new TextDecoder();
let done = false;
while (!done) {
const { value, done: readerDone } = await reader.read();
done = readerDone;
const chunk = decoder.decode(value, { stream: true });
setMessages((prevMessages) => [
...prevMessages,
{ role: "assistant", content: chunk },
]);
}
} catch (error) {
console.error("Error:", error);
} finally {
setIsTyping(false);
setInput("");
}
};
Section 3:
Building the UI frame of our application
- This JSX code defines the UI layout for an AI chatbot assistant inside a structured, styled container.
- The outer is a flex container that ensures a full-screen height layout with a light gray background.
- The component is rendered at the top, likely containing a navigation bar or branding.
- Inside a centered , a component is used to create a structured chat interface with a maximum width of 2xl.
- The contains the title "AI Chatbot for Job Assistant", providing context for the user.
- The section is set to a height of 60% of the viewport (60vh) and allows scrolling for long conversations.
- Messages are dynamically displayed from the messages array, with user messages aligned to the right and assistant messages to the left.
- If the chatbot is processing a response (isTyping is true), a loading indicator () appears with the text "AI is thinking...".
- The contains a form where users can type messages using an field and submit them with a Send button.
- The Send button is disabled while the AI is generating a response to prevent multiple submissions.
return (
AI Chatbot for Job assistant
{messages.map((m, index) => (
{m.content}
))}
{isTyping && (
AI is thinking...
)}
);
8. Building the Header component
export default function Header() {
return (
Chat + chatbot
);
}
9. Building the Spinner component
export function Spinner() {
return (
);
}
10. Running the Application
npm run dev
- Visit http://localhost:3000 to see your chatbot in action.
- Sign up https://openrouter.ai/settings/keys to get your API keys
11. Deploying to Vercel
https://youtu.be/2HBIzEx6IZA
12. Conclusion
This guide walks you through setting up and building a chatbot application with Next.js. You now have a functional AI chatbot using OpenAI’s API via OpenRouter.