Building a System Information Fetch Tool in Bash
I once needed a quick system overview—CPU load, memory usage, disk space—but opening multiple commands felt inefficient. Instead of running top, df -h, and free -m separately, I decided to automate everything in a single Bash script. The result? A custom system fetch tool that provides essential system details at a glance. In this guide, you'll learn how to build your own step by step, improving your Bash scripting skills along the way. Step 1: The Basics (What We’re Fetching) Before we jump into coding, let’s outline what information we need: ✅ CPU Model & Load (/proc/cpuinfo, top) ✅ Memory Usage (free -m) ✅ Disk Space (df -h) ✅ System Uptime (uptime) ✅ Network Information (ip a, hostname -I) Step 2: Writing the Script (Fundamentals First) 1️⃣ Shebang & Setup Start by specifying Bash as the interpreter and clearing the screen: #!/bin/bash clear 2️⃣ Fetching CPU Info Extract the CPU model name using grep on /proc/cpuinfo: cpu_model=$(grep "model name" /proc/cpuinfo | head -1 | cut -d ':' -f2) Get the CPU load using top (or uptime for a lightweight alternative): cpu_load=$(top -bn1 | grep "load average" | awk '{print $10}') 3️⃣ Checking Memory Usage Use free -m to display RAM usage in MB: mem_usage=$(free -m | awk 'NR==2{printf "Used: %sMB / Total: %sMB", $3, $2}') 4️⃣ Fetching Disk Space List available disk space using df -h: disk_usage=$(df -h | awk '$NF=="/"{printf "Used: %d%%", $5}') 5️⃣ Displaying Network Info Fetch the current IP address: ip_address=$(hostname -I | awk '{print $1}') Step 3: Formatting Output for Readability Instead of printing raw text, format the output neatly using colors and spacing: echo -e "\033[32mSystem Information:\033[0m" echo "-------------------------------" echo -e "CPU Model: $cpu_model" echo -e "CPU Load: $cpu_load" echo -e "Memory: $mem_usage" echo -e "Disk Space: $disk_usage" echo -e "IP Address: $ip_address"
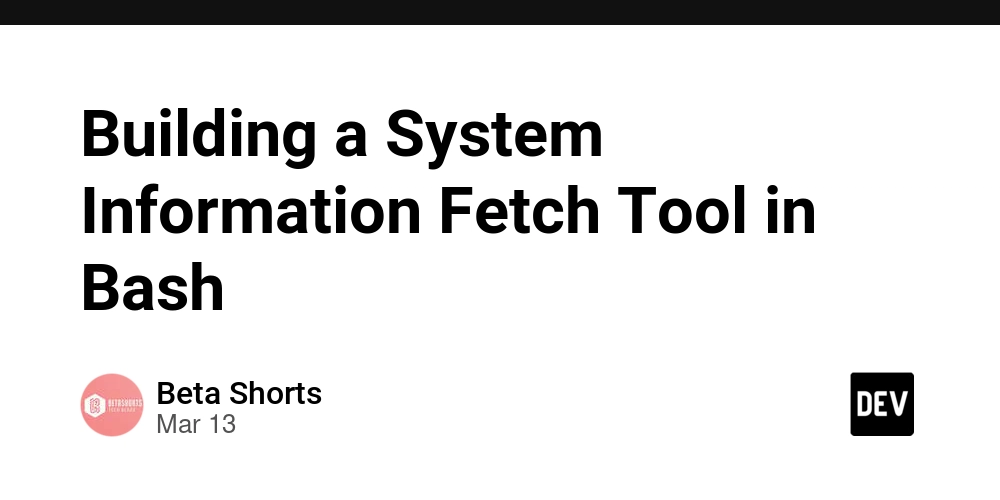
I once needed a quick system overview—CPU load, memory usage, disk space—but opening multiple commands felt inefficient. Instead of running top
, df -h
, and free -m
separately, I decided to automate everything in a single Bash script.
The result? A custom system fetch tool that provides essential system details at a glance. In this guide, you'll learn how to build your own step by step, improving your Bash scripting skills along the way.
Step 1: The Basics (What We’re Fetching)
Before we jump into coding, let’s outline what information we need:
✅ CPU Model & Load (/proc/cpuinfo
, top
)
✅ Memory Usage (free -m
)
✅ Disk Space (df -h
)
✅ System Uptime (uptime
)
✅ Network Information (ip a
, hostname -I
)
Step 2: Writing the Script (Fundamentals First)
1️⃣ Shebang & Setup
Start by specifying Bash as the interpreter and clearing the screen:
#!/bin/bash
clear
2️⃣ Fetching CPU Info
Extract the CPU model name using grep
on /proc/cpuinfo
:
cpu_model=$(grep "model name" /proc/cpuinfo | head -1 | cut -d ':' -f2)
Get the CPU load using top
(or uptime
for a lightweight alternative):
cpu_load=$(top -bn1 | grep "load average" | awk '{print $10}')
3️⃣ Checking Memory Usage
Use free -m
to display RAM usage in MB:
mem_usage=$(free -m | awk 'NR==2{printf "Used: %sMB / Total: %sMB", $3, $2}')
4️⃣ Fetching Disk Space
List available disk space using df -h
:
disk_usage=$(df -h | awk '$NF=="/"{printf "Used: %d%%", $5}')
5️⃣ Displaying Network Info
Fetch the current IP address:
ip_address=$(hostname -I | awk '{print $1}')
Step 3: Formatting Output for Readability
Instead of printing raw text, format the output neatly using colors and spacing:
echo -e "\033[32mSystem Information:\033[0m"
echo "-------------------------------"
echo -e "CPU Model: $cpu_model"
echo -e "CPU Load: $cpu_load"
echo -e "Memory: $mem_usage"
echo -e "Disk Space: $disk_usage"
echo -e "IP Address: $ip_address"