Building a FastAPI Book API with CI/CD Pipelines (Using Github Actions) and Docker Deployment
In this article, I’ll walk you through the development of a FastAPI-based book API, complete with a Continuous Integration (CI) and Continuous Deployment (CD) pipeline. This project is part of a Devops internship challenge i'm doing with HNG, and is aimed to retrieve book details by ID, and is deployed to an AWS ec2 instance using Docker. Project Overview The goal of this project is to create a simple yet robust API that retrieves book details by its ID. The repository provided already included a predefined structure, and we had to ensure that all endpoints were correctly implemented and accessible via Nginx. These were the goals to be achieved in this challenge: Add an endpoint to retrieve a book by its ID, so that it is accessible via this url path /api/v1/books/{book_id}. Set up a CI pipeline that automates tests whenever a pull request is made to the main branch. Set up a CD pipeline that automates the deployment process whenever changes are pushed to the main branch. Project Structure fastapi-book-project/ ├── api/ │ ├── db/ │ │ ├── __init__.py │ │ └── schemas.py # Data models and in-memory database │ ├── routes/ │ │ ├── __init__.py │ │ └── books.py # Book route handlers │ └── router.py # API router configuration ├── core/ │ ├── __init__.py │ └── config.py # Application settings ├── tests/ │ ├── __init__.py │ └── test_books.py # API endpoint tests ├── main.py # Application entry point ├── requirements.txt # Project dependencies └── README.md Setting Up The initial step was cloning the provided git repo, which contained the basic project structure. Here's a link to the project's git repo, https://github.com/NonsoEchendu/fastapi-book-project. To clone: git clone https://github.com/NonsoEchendu/fastapi-book-project.git So let's start with the first task in the challenge:
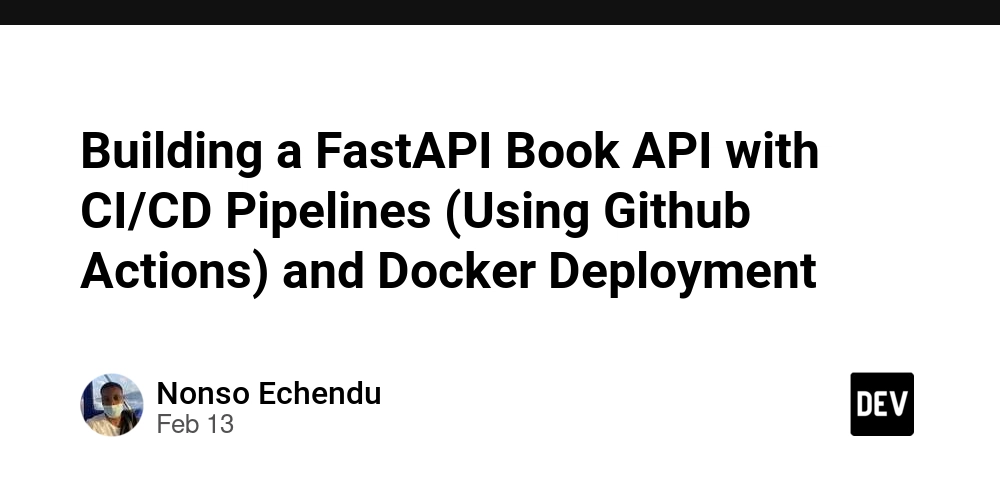
In this article, I’ll walk you through the development of a FastAPI-based book API, complete with a Continuous Integration (CI) and Continuous Deployment (CD) pipeline. This project is part of a Devops internship challenge i'm doing with HNG, and is aimed to retrieve book details by ID, and is deployed to an AWS ec2 instance using Docker.
Project Overview
The goal of this project is to create a simple yet robust API that retrieves book details by its ID.
The repository provided already included a predefined structure, and we had to ensure that all endpoints were correctly implemented and accessible via Nginx.
These were the goals to be achieved in this challenge:
Add an endpoint to retrieve a book by its ID, so that it is accessible via this url path
/api/v1/books/{book_id}
.Set up a CI pipeline that automates tests whenever a pull request is made to the
main
branch.Set up a CD pipeline that automates the deployment process whenever changes are pushed to the
main
branch.
Project Structure
fastapi-book-project/
├── api/
│ ├── db/
│ │ ├── __init__.py
│ │ └── schemas.py # Data models and in-memory database
│ ├── routes/
│ │ ├── __init__.py
│ │ └── books.py # Book route handlers
│ └── router.py # API router configuration
├── core/
│ ├── __init__.py
│ └── config.py # Application settings
├── tests/
│ ├── __init__.py
│ └── test_books.py # API endpoint tests
├── main.py # Application entry point
├── requirements.txt # Project dependencies
└── README.md
Setting Up
The initial step was cloning the provided git repo, which contained the basic project structure. Here's a link to the project's git repo, https://github.com/NonsoEchendu/fastapi-book-project.
To clone:
git clone https://github.com/NonsoEchendu/fastapi-book-project.git
So let's start with the first task in the challenge: