Building a Currency Converter App: A Step-by-Step Guide Using Exchange Rates APIs
Currency conversion is a vital feature for businesses, travelers, and financial professionals who deal with international transactions. With real-time data availability, developers can integrate exchange rates API into applications to provide accurate conversions. This blog will guide you through building a currency converter app step by step using an API for exchange rates, making it valuable for developers, SaaS platforms, and API communities. Why Build a Currency Converter App? A currency converter app simplifies global transactions by providing instant exchange rate calculations. It is beneficial for: *E-commerce businesses *– Enabling customers to view product prices in their local currency. Travel applications – Helping travelers estimate expenses in different countries. Financial tools – Assisting in forex trading and investment decision-making. By leveraging an exchange rates API, developers can ensure the accuracy and efficiency of their applications while reducing manual efforts. Tools and Technologies Needed Before diving into the development process, here are the essential tools and technologies required: Programming Languages: JavaScript (Node.js), Python, or PHP Frameworks: React, Vue.js (for frontend), Flask, or Express.js (for backend) APIs: A reliable API for exchange rates Hosting Services: AWS, Heroku, or Vercel Database: Firebase, MongoDB, or MySQL (if storing transaction history) Step-by-Step Guide to Building a Currency Converter App Step 1: Choose a Reliable Exchange Rates API The core component of a currency converter app is the exchange rates API. A robust API ensures: Real-time currency exchange updates High uptime and reliability Secure and scalable performance Some well-known API for exchange rates options include: APILayer's Exchange Rates API – Provides real-time and historical exchange rates with enterprise-level security. Open Exchange Rates – Offers a free plan with limited access. Fixer.io – A lightweight API for basic conversion needs. Step 2: Set Up Your Development Environment To get started, install the necessary tools based on your chosen tech stack. If using Node.js, initialize your project: mkdir currency-converter cd currency-converter npm init -y Install required dependencies: npm install express axios dotenv Step 3: Fetch Real-Time Exchange Rates Create a .env file to store your API key securely: API_KEY=your_api_key_here Then, set up an API request in your backend: const express = require('express'); const axios = require('axios'); require('dotenv').config(); const app = express(); const PORT = 5000; app.get('/convert', async (req, res) => { const { from, to, amount } = req.query; try { const response = await axios.get(https://api.apilayer.com/exchangerates?base=${from}&symbols=${to}&apikey=${process.env.API_KEY}); const rate = response.data.rates[to]; const convertedAmount = (amount * rate).toFixed(2); res.json({ from, to, amount, convertedAmount }); } catch (error) { res.status(500).json({ error: 'Unable to fetch exchange rates' }); } }); app.listen(PORT, () => console.log(Server running on port ${PORT})); This will set up a simple API endpoint to fetch and convert currency rates dynamically. Step 4: Create a Frontend Interface A user-friendly frontend allows users to enter amounts and view conversions. Using React: import { useState } from 'react'; import axios from 'axios'; function CurrencyConverter() { const [from, setFrom] = useState('USD'); const [to, setTo] = useState('EUR'); const [amount, setAmount] = useState(1); const [result, setResult] = useState(null); const convertCurrency = async () => { const response = await axios.get(`/convert?from=${from}&to=${to}&amount=${amount}`); setResult(response.data.convertedAmount); }; return ( setAmount(e.target.value)} /> Convert {result && Converted Amount: {result} {to}} ); } export default CurrencyConverter; Step 5: Deploy and Optimize Once your app is ready, deploy it using a platform like Vercel, Netlify, or Heroku. Monitor performance and optimize API requests to avoid excessive calls and reduce latency. Best Practices for Building a Currency Converter App To ensure a seamless experience, consider the following best practices: Cache API responses to minimize unnecessary requests and improve performance. Implement error handling to manage API failures effectively. Optimize for mobile users by ensuring a responsive UI. Use secure API authentication to protect your application from unauthorized access. Conclusion Building a currency converter app is an excellent project for developers, API communities, and SaaS businesses. By integrating a reliable exchange rates API, you can provide real-time currency conversions, enhancing user experience and functionality. Whether you're creating an e-commerce platform, a travel app, or a financial tool,
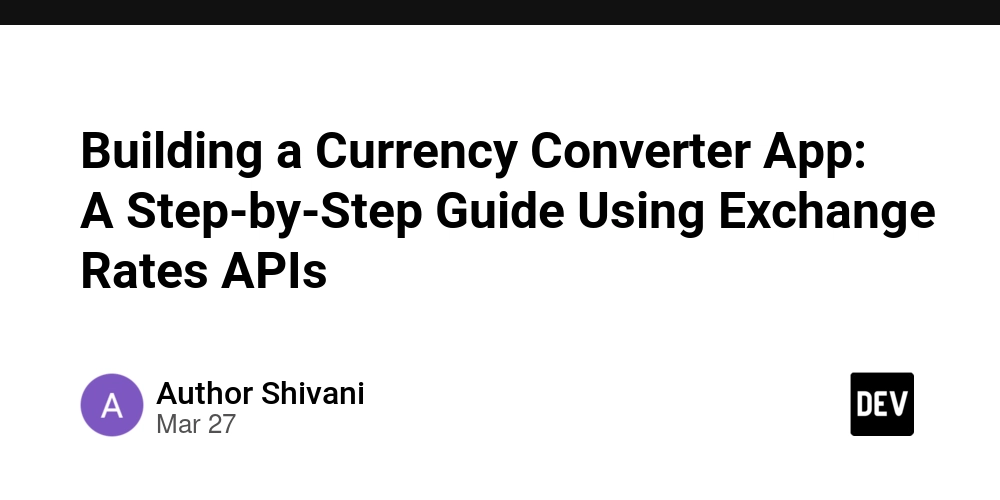
Currency conversion is a vital feature for businesses, travelers, and financial professionals who deal with international transactions. With real-time data availability, developers can integrate exchange rates API into applications to provide accurate conversions. This blog will guide you through building a currency converter app step by step using an API for exchange rates, making it valuable for developers, SaaS platforms, and API communities.
Why Build a Currency Converter App?
A currency converter app simplifies global transactions by providing instant exchange rate calculations. It is beneficial for:
*E-commerce businesses *– Enabling customers to view product prices in their local currency.
Travel applications – Helping travelers estimate expenses in different countries.
Financial tools – Assisting in forex trading and investment decision-making.
By leveraging an exchange rates API, developers can ensure the accuracy and efficiency of their applications while reducing manual efforts.
Tools and Technologies Needed
Before diving into the development process, here are the essential tools and technologies required:
Programming Languages: JavaScript (Node.js), Python, or PHP
Frameworks: React, Vue.js (for frontend), Flask, or Express.js (for backend)
APIs: A reliable API for exchange rates
Hosting Services: AWS, Heroku, or Vercel
Database: Firebase, MongoDB, or MySQL (if storing transaction history)
Step-by-Step Guide to Building a Currency Converter App
Step 1: Choose a Reliable Exchange Rates API
The core component of a currency converter app is the exchange rates API. A robust API ensures:
Real-time currency exchange updates
High uptime and reliability
Secure and scalable performance
Some well-known API for exchange rates options include:
APILayer's Exchange Rates API – Provides real-time and historical exchange rates with enterprise-level security.
Open Exchange Rates – Offers a free plan with limited access.
Fixer.io – A lightweight API for basic conversion needs.
Step 2: Set Up Your Development Environment
To get started, install the necessary tools based on your chosen tech stack. If using Node.js, initialize your project:
mkdir currency-converter
cd currency-converter
npm init -y
Install required dependencies:
npm install express axios dotenv
Step 3: Fetch Real-Time Exchange Rates
Create a .env file to store your API key securely:
API_KEY=your_api_key_here
Then, set up an API request in your backend:
const express = require('express');
const axios = require('axios');
require('dotenv').config();
const app = express();
const PORT = 5000;
app.get('/convert', async (req, res) => {
const { from, to, amount } = req.query;
try {
const response = await axios.get(https://api.apilayer.com/exchangerates?base=${from}&symbols=${to}&apikey=${process.env.API_KEY}
);
const rate = response.data.rates[to];
const convertedAmount = (amount * rate).toFixed(2);
res.json({ from, to, amount, convertedAmount });
} catch (error) {
res.status(500).json({ error: 'Unable to fetch exchange rates' });
}
});
app.listen(PORT, () => console.log(Server running on port ${PORT}
));
This will set up a simple API endpoint to fetch and convert currency rates dynamically.
Step 4: Create a Frontend Interface
A user-friendly frontend allows users to enter amounts and view conversions. Using React:
import { useState } from 'react';
import axios from 'axios';
function CurrencyConverter() {
const [from, setFrom] = useState('USD');
const [to, setTo] = useState('EUR');
const [amount, setAmount] = useState(1);
const [result, setResult] = useState(null);
const convertCurrency = async () => {
const response = await axios.get(`/convert?from=${from}&to=${to}&amount=${amount}`);
setResult(response.data.convertedAmount);
};
return (
setAmount(e.target.value)} />
{result && Converted Amount: {result} {to}}
);
}
export default CurrencyConverter;
Step 5: Deploy and Optimize
Once your app is ready, deploy it using a platform like Vercel, Netlify, or Heroku. Monitor performance and optimize API requests to avoid excessive calls and reduce latency.
Best Practices for Building a Currency Converter App
To ensure a seamless experience, consider the following best practices:
Cache API responses to minimize unnecessary requests and improve performance.
Implement error handling to manage API failures effectively.
Optimize for mobile users by ensuring a responsive UI.
Use secure API authentication to protect your application from unauthorized access.
Conclusion
Building a currency converter app is an excellent project for developers, API communities, and SaaS businesses. By integrating a reliable exchange rates API, you can provide real-time currency conversions, enhancing user experience and functionality. Whether you're creating an e-commerce platform, a travel app, or a financial tool, incorporating an API for exchange rates ensures accuracy and efficiency.