Automation Programming Basics
In today’s fast-paced world, automation programming is a vital skill for developers, IT professionals, and even hobbyists. Whether it's automating file management, data scraping, or repetitive tasks, automation saves time, reduces errors, and boosts productivity. This post covers the basics to get you started in automation programming. What is Automation Programming? Automation programming involves writing scripts or software that perform tasks without manual intervention. It’s widely used in system administration, web testing, data processing, DevOps, and more. Benefits of Automation Efficiency: Complete tasks faster than doing them manually. Accuracy: Reduce the chances of human error. Scalability: Automate tasks at scale (e.g., managing hundreds of files or websites). Consistency: Ensure tasks are done the same way every time. Popular Languages for Automation Python: Simple syntax and powerful libraries like os, shutil, requests, selenium, and pandas. Bash: Great for system and server-side scripting on Linux/Unix systems. PowerShell: Ideal for Windows system automation. JavaScript (Node.js): Used in automating web services, browsers, or file tasks. Common Automation Use Cases Renaming and organizing files/folders Automating backups Web scraping and data collection Email and notification automation Testing web applications Scheduling repetitive system tasks (cron jobs) Basic Python Automation Example Here’s a simple script to move files from one folder to another based on file extension: import os import shutil source = 'Downloads' destination = 'Images' for file in os.listdir(source): if file.endswith('.jpg') or file.endswith('.png'): shutil.move(os.path.join(source, file), os.path.join(destination, file)) Tools That Help with Automation Task Schedulers: cron (Linux/macOS), Task Scheduler (Windows) Web Automation Tools: Selenium, Puppeteer CI/CD Tools: GitHub Actions, Jenkins File Watchers: watchdog (Python) for reacting to file system changes Best Practices Always test your scripts in a safe environment before production. Add logs to track script actions and errors. Use virtual environments to manage dependencies. Keep your scripts modular and well-documented. Secure your scripts if they deal with sensitive data or credentials. Conclusion Learning automation programming can drastically enhance your workflow and problem-solving skills. Start small, automate daily tasks, and explore advanced tools as you grow. The world of automation is wide open—begin automating today!
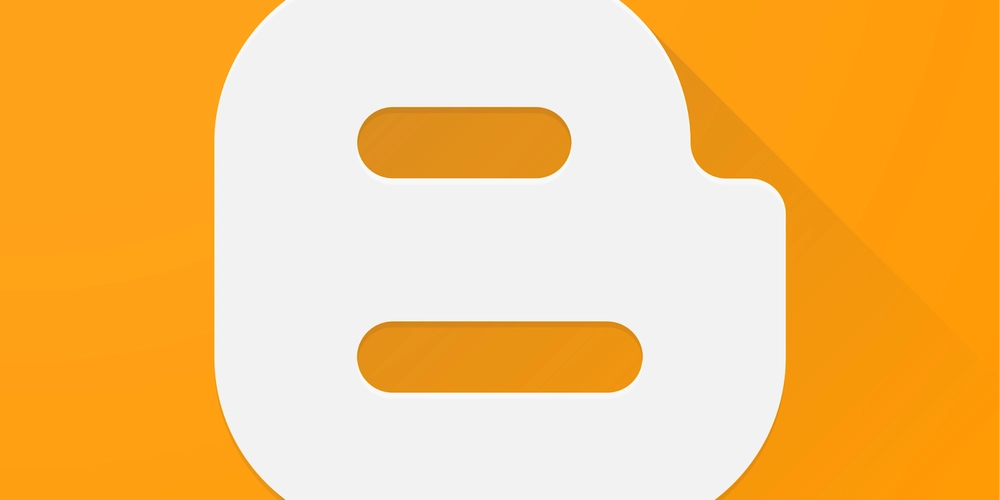
In today’s fast-paced world, automation programming is a vital skill for developers, IT professionals, and even hobbyists. Whether it's automating file management, data scraping, or repetitive tasks, automation saves time, reduces errors, and boosts productivity. This post covers the basics to get you started in automation programming.
What is Automation Programming?
Automation programming involves writing scripts or software that perform tasks without manual intervention. It’s widely used in system administration, web testing, data processing, DevOps, and more.
Benefits of Automation
- Efficiency: Complete tasks faster than doing them manually.
- Accuracy: Reduce the chances of human error.
- Scalability: Automate tasks at scale (e.g., managing hundreds of files or websites).
- Consistency: Ensure tasks are done the same way every time.
Popular Languages for Automation
-
Python: Simple syntax and powerful libraries like
os
,shutil
,requests
,selenium
, andpandas
. - Bash: Great for system and server-side scripting on Linux/Unix systems.
- PowerShell: Ideal for Windows system automation.
- JavaScript (Node.js): Used in automating web services, browsers, or file tasks.
Common Automation Use Cases
- Renaming and organizing files/folders
- Automating backups
- Web scraping and data collection
- Email and notification automation
- Testing web applications
- Scheduling repetitive system tasks (cron jobs)
Basic Python Automation Example
Here’s a simple script to move files from one folder to another based on file extension:
import os
import shutil
source = 'Downloads'
destination = 'Images'
for file in os.listdir(source):
if file.endswith('.jpg') or file.endswith('.png'):
shutil.move(os.path.join(source, file), os.path.join(destination, file))
Tools That Help with Automation
-
Task Schedulers:
cron
(Linux/macOS), Task Scheduler (Windows) - Web Automation Tools: Selenium, Puppeteer
- CI/CD Tools: GitHub Actions, Jenkins
-
File Watchers:
watchdog
(Python) for reacting to file system changes
Best Practices
- Always test your scripts in a safe environment before production.
- Add logs to track script actions and errors.
- Use virtual environments to manage dependencies.
- Keep your scripts modular and well-documented.
- Secure your scripts if they deal with sensitive data or credentials.
Conclusion
Learning automation programming can drastically enhance your workflow and problem-solving skills. Start small, automate daily tasks, and explore advanced tools as you grow. The world of automation is wide open—begin automating today!