Java continues to evolve, and leveraging advanced techniques can significantly enhance the efficiency and scalability of applications. Here are some cutting-edge approaches every Java developer should explore: 1️⃣ Fork-Join Model for Parallel Processing The Fork-Join framework enables parallel execution by breaking tasks into smaller subtasks, maximizing CPU efficiency. Example: Parallel Sum Calculation using Fork-Join: import java.util.concurrent.RecursiveTask; import java.util.concurrent.ForkJoinPool; public class ParallelSum extends RecursiveTask { private final int[] array; private final int start, end; private static final int THRESHOLD = 1000; public ParallelSum(int[] array, int start, int end) { this.array = array; this.start = start; this.end = end; } @Override protected Integer compute() { if (end - start
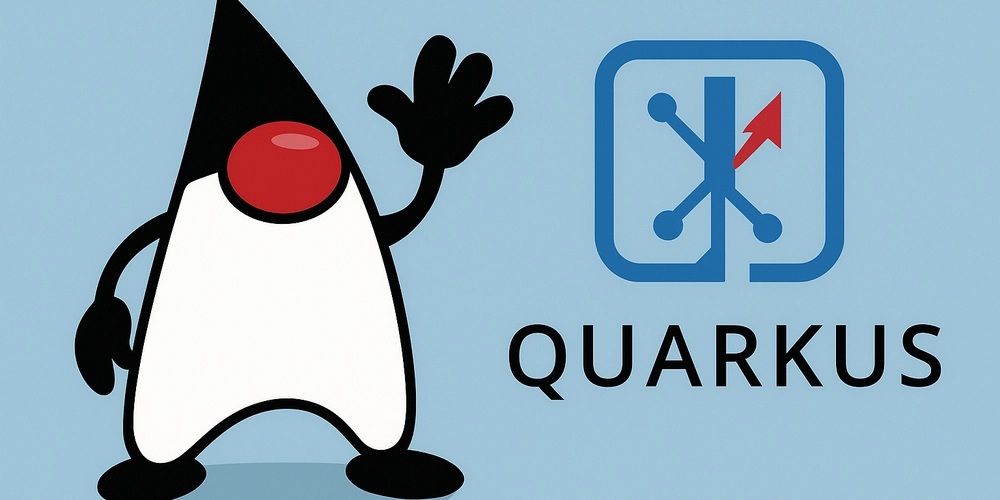
Java continues to evolve, and leveraging advanced techniques can significantly enhance the efficiency and scalability of applications. Here are some cutting-edge approaches every Java developer should explore:
1️⃣ Fork-Join Model for Parallel Processing
The Fork-Join framework enables parallel execution by breaking tasks into smaller subtasks, maximizing CPU efficiency.
Example: Parallel Sum Calculation using Fork-Join:
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class ParallelSum extends RecursiveTask {
private final int[] array;
private final int start, end;
private static final int THRESHOLD = 1000;
public ParallelSum(int[] array, int start, int end) {
this.array = array;
this.start = start;
this.end = end;
}
@Override
protected Integer compute() {
if (end - start <= THRESHOLD) {
int sum = 0;
for (int i = start; i < end; i++) sum += array[i];
return sum;
} else {
int mid = (start + end) / 2;
ParallelSum task1 = new ParallelSum(array, start, mid);
ParallelSum task2 = new ParallelSum(array, mid, end);
task1.fork();
int result2 = task2.compute();
int result1 = task1.join();
return result1 + result2;
}
}
public static void main(String[] args) {
int[] array = new int[10000]; // Initialize with values
ForkJoinPool pool = new ForkJoinPool();
int result = pool.invoke(new ParallelSum(array, 0, array.length));
System.out.println("Total sum: " + result);
}
}
✅ Benefit: Improved performance by utilizing multiple CPU cores efficiently.
2️⃣ Aspect-Oriented Programming (AOP) with AspectJ
AOP allows separation of cross-cutting concerns such as logging, security, and exception handling, leading to cleaner and more maintainable code.
Example: Logging with AspectJ:
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
@Aspect
public class LoggingAspect {
@Before("execution(* com.yourapp.service.*.*(..))")
public void logMethodCall() {
System.out.println("Method executed!");
}
}
✅ Benefit: Reduces code duplication and enhances modularity.
3️⃣ Microservices with Quarkus
Quarkus is optimized for Kubernetes and cloud-native development, offering fast startup times and minimal memory footprint.
Example: Simple REST API with Quarkus:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class HelloResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
return "Hello, world!";
}
}
✅ Benefit: Build high-performance, cloud-ready Java applications.