A Practical Guide to Robust Webhooks in Suphle
Introduction This article proposes a more elegant way to implement webhooks. The code examples are written in PHP using Suphle, a polished framework for building modern full-stack PHP applications. A robust webhook implementation—such as consuming a payment gateway—requires several key elements, which I’ll walk through below. But first, let’s examine some pseudocode representing the ideal outcome. Any implementation that goes far beyond these essentials risks becoming boilerplate-heavy and unnecessarily complex. Target Behavior A typical implementation of our webhook integration might follow this flow: Initiate payment Receive a response Parse and persist relevant data We generally expect this sequence to complete successfully—requests fire as expected, payloads are intact, and the application responds meaningfully. But real-world systems often grow beyond this simplistic ideal. Complex workflows may require observability, fault tolerance, and testability woven into the entire flow. Let’s explore what that entails. Bottlenecks in the Process There are several critical pressure points where things can go wrong, and it's unwise to treat webhook handling as purely a business logic concern. It belongs in the infrastructure layer. Some examples: The outgoing request might fail due to a missing parameter. Without graceful error handling, the user is stuck. At the application boundary, we must enforce static types to reliably intercept data. If not, failure will only surface on the provider’s end, leaving users confused until reconciliation (if any) occurs. Payload processing should happen in a decoupled business layer, enabling graceful failure and testability via mock payloads—all while respecting separation of concerns. Fleshing It Out Suphle helps handle infrastructure-layer concerns out of the box, offering tools for fail-safes at key points in the request lifecycle. Let’s start with outbound request initiation. Suphle provides a base class for such requests: Suphle\IO\Http\BaseHttpRequest. It wraps outgoing PSR-compliant requests with useful features like error-catching, telemetry reporting, fallback support, and domain-level object mapping (DTOs). Here’s a sample request borrowed from the official documentation: use Suphle\IO\Http\BaseHttpRequest; use Psr\Http\Message\ResponseInterface; class TriggerPayment extends BaseHttpRequest { public function getRequestUrl ():string { return "http://some-gateway.com/pay"; } protected function getHttpResponse ():ResponseInterface { return $this->requestClient->request( "post", $this->getRequestUrl()/*, $options*/ ); } protected function convertToDomainObject (ResponseInterface $response) { return $response; // filter to taste or cast to DSL } } We can then consume this in a coordinator like so: class HttpCoordinator extends ServiceCoordinator { public function __construct (protected readonly TriggerPayment $httpService) { // } public function makePayment ():iterable { $dslObject = $this->httpService->getDomainObject(); if ($this->httpService->hasErrors()) { // derive $dslObject some other way } return ["data" => $dslObject]; } This is both neat and powerful. With the outbound request sorted, let’s now explore the return leg of the process—handling incoming webhook payloads. Suphle provides a specialized request reader, Suphle\Services\Structures\ModellessPayload, which allows you to extract known domain objects (DSLs) from payloads. Validation failures at this level are sent to your telemetry service, without impacting the user journey. Here’s a practical example: use Suphle\Services\Structures\ModellessPayload; class ExtractPaymentFields extends ModellessPayload { protected function convertToDomainObject () { $data = $this->payloadStorage->getKey("data"); return new GenericPaidDSL($data["trx_id"]); } } And consumed in a coordinator like so: class BaseCoordinator extends ServiceCoordinator { public function __construct (protected readonly TransactionService $transactionService) { // } public function myCartItems () { return [ "data" => $this->transactionService->modelsToUpdate() ]; } public function genericWebhook (ExtractPaymentFields $payloadReader):array { return [ "data" => $this->transactionService->updateModels( $payloadReader->getDomainObject()/*returns instance of GenericPaidDSL*/ ) ]; } } The TransactionService encapsulates all the safety mechanisms we've introduced so far. When built correctly, extending Suphle\Services\UpdatefulService and implementing the SystemModelEdit interface guarantees mutative operations occur within safe, locked transactions—ensuring no two users
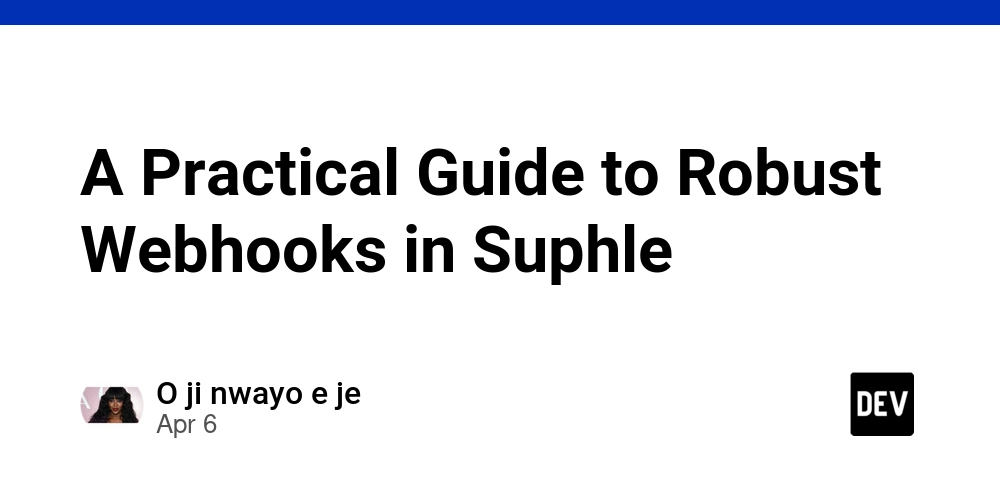
Introduction
This article proposes a more elegant way to implement webhooks. The code examples are written in PHP using Suphle, a polished framework for building modern full-stack PHP applications.
A robust webhook implementation—such as consuming a payment gateway—requires several key elements, which I’ll walk through below. But first, let’s examine some pseudocode representing the ideal outcome. Any implementation that goes far beyond these essentials risks becoming boilerplate-heavy and unnecessarily complex.
Target Behavior
A typical implementation of our webhook integration might follow this flow:
- Initiate payment
- Receive a response
- Parse and persist relevant data
We generally expect this sequence to complete successfully—requests fire as expected, payloads are intact, and the application responds meaningfully. But real-world systems often grow beyond this simplistic ideal. Complex workflows may require observability, fault tolerance, and testability woven into the entire flow. Let’s explore what that entails.
Bottlenecks in the Process
There are several critical pressure points where things can go wrong, and it's unwise to treat webhook handling as purely a business logic concern. It belongs in the infrastructure layer. Some examples:
- The outgoing request might fail due to a missing parameter. Without graceful error handling, the user is stuck.
- At the application boundary, we must enforce static types to reliably intercept data. If not, failure will only surface on the provider’s end, leaving users confused until reconciliation (if any) occurs.
- Payload processing should happen in a decoupled business layer, enabling graceful failure and testability via mock payloads—all while respecting separation of concerns.
Fleshing It Out
Suphle helps handle infrastructure-layer concerns out of the box, offering tools for fail-safes at key points in the request lifecycle.
Let’s start with outbound request initiation. Suphle provides a base class for such requests: Suphle\IO\Http\BaseHttpRequest
. It wraps outgoing PSR-compliant requests with useful features like error-catching, telemetry reporting, fallback support, and domain-level object mapping (DTOs).
Here’s a sample request borrowed from the official documentation:
use Suphle\IO\Http\BaseHttpRequest;
use Psr\Http\Message\ResponseInterface;
class TriggerPayment extends BaseHttpRequest {
public function getRequestUrl ():string {
return "http://some-gateway.com/pay";
}
protected function getHttpResponse ():ResponseInterface {
return $this->requestClient->request(
"post", $this->getRequestUrl()/*, $options*/
);
}
protected function convertToDomainObject (ResponseInterface $response) {
return $response; // filter to taste or cast to DSL
}
}
We can then consume this in a coordinator like so:
class HttpCoordinator extends ServiceCoordinator {
public function __construct (protected readonly TriggerPayment $httpService) {
//
}
public function makePayment ():iterable {
$dslObject = $this->httpService->getDomainObject();
if ($this->httpService->hasErrors()) {
// derive $dslObject some other way
}
return ["data" => $dslObject];
}
This is both neat and powerful. With the outbound request sorted, let’s now explore the return leg of the process—handling incoming webhook payloads.
Suphle provides a specialized request reader, Suphle\Services\Structures\ModellessPayload
, which allows you to extract known domain objects (DSLs) from payloads. Validation failures at this level are sent to your telemetry service, without impacting the user journey.
Here’s a practical example:
use Suphle\Services\Structures\ModellessPayload;
class ExtractPaymentFields extends ModellessPayload {
protected function convertToDomainObject () {
$data = $this->payloadStorage->getKey("data");
return new GenericPaidDSL($data["trx_id"]);
}
}
And consumed in a coordinator like so:
class BaseCoordinator extends ServiceCoordinator {
public function __construct (protected readonly TransactionService $transactionService) {
//
}
public function myCartItems () {
return [
"data" => $this->transactionService->modelsToUpdate()
];
}
public function genericWebhook (ExtractPaymentFields $payloadReader):array {
return [
"data" => $this->transactionService->updateModels(
$payloadReader->getDomainObject()/*returns instance of GenericPaidDSL*/
)
];
}
}
The TransactionService
encapsulates all the safety mechanisms we've introduced so far. When built correctly, extending Suphle\Services\UpdatefulService
and implementing the SystemModelEdit
interface guarantees mutative operations occur within safe, locked transactions—ensuring no two users mutate the same resource concurrently.
You can read more about these guarantees in the documentation on mutative decorators.
use Suphle\Services\{UpdatefulService, Structures\BaseErrorCatcherService};
use Suphle\Services\Decorators\{InterceptsCalls, VariableDependencies};
use Suphle\Contracts\{Events, Services\CallInterceptors\SystemModelEdit};
use Suphle\Events\EmitProxy;
use Illuminate\Support\Collection;
#[InterceptsCalls(SystemModelEdit::class)]
#[VariableDependencies([
"setPayloadStorage", "setPlaceholderStorage"
])]
class TransactionService extends UpdatefulService implements SystemModelEdit {
use BaseErrorCatcherService, EmitProxy;
public const EMPTIED_CART = "cart_empty";
public function __construct (
protected readonly CartService $cartService,
protected readonly TransactionVerifier $verifyPayment,
private readonly Events $eventManager
) {
//
}
public function updateModels (object $genericPaidDSL) {
$products = $this->modelsToUpdate();
$this->shouldDispenseService($genericPaidDSL, $products);
$products->each->update(["sold" => true]);
$this->emitHelper(self::EMPTIED_CART, $products); // received by payment, order modules etc
return $this->cartService->delete();
}
public function modelsToUpdate ():iterable {
return $this->cartService->authProducts;
}
public function shouldDispenseService(GenericPaidDSL $genericPaidDSL, Collection $products):void {
$this->verifyPayment->setTrxId($genericPaidDSL->trxId); // used to fetch request details
$dslObject = $this->verifyPayment->getDomainObject();
if (
$this->verifyPayment->hasErrors() ||
!$dslObject->matchAmount($products->sum("price"))
)
throw new Exception("Fraudulent Payment");
}
}
Much of the code above is standard boilerplate with dedicated documentation chapters. We’ve included it here for realism and completeness. What's most relevant to our webhook flow is how naturally GenericPaidDSL
is consumed throughout the layers—without relying on brittle scaffolding or random PHP wiring.
Bonus: Handling Multiple Providers
Suppose your application integrates with multiple payment gateways. You could adjust ExtractPaymentFields
to dynamically map incoming payloads to their appropriate DSLs:
use Suphle\Services\Structures\ModellessPayload;
class ExtractPaymentFields extends ModellessPayload {
protected function convertToDomainObject () {
$data = $this->payloadStorage->getKey("data");
return match ($data["provider_indicator"]) {
"paystack" => new PaystackDSL($data["trx_id"]),
default => new FlutterwaveDSL($data["trx_id"]),
};
}
}
Alternatively, you could delegate this responsibility to Suphle’s Condition factories
for even tighter encapsulation—ideal when DSLs require container hydration.
Testing
Testing is integral to engineering confidence in your implementation. Fortunately, Suphle’s architecture makes this easy:
- You can skip all
BaseHttpRequest
subclasses and inject DSLs directly into domain classes. - Optionally test
ModellessPayload
subclasses if they contain complex conditionals. - Focus most of your testing efforts on
TransactionService::updateModels
.
Here’s how a test could look:
use AllModules\CartModule\{Meta\CartModuleDescriptor, Services\TransactionService};
use AppModels\{CartProduct, User as EloquentUser, Product};
use Suphle\Testing\{TestTypes\ModuleLevelTest, Proxies\WriteOnlyContainer, Condiments\EmittedEventsCatcher, Condiments\BaseDatabasePopulator};
class TransactionTest extends ModuleLevelTest {
use BaseDatabasePopulator, EmittedEventsCatcher;
protected function getActiveEntity ():string {
return EloquentUser::class;
}
protected function getModules(): array {
return [
$this->replicateModule(CartModuleDescriptor::class, function (WriteOnlyContainer $container) {
$container->replaceWithMock(TransactionService::class, TransactionService::class, [
"shouldDispenseService" => null // or simulate failure if you wish: $this->throwException(InsufficientBalance::class)
]);
})
];
}
public function test_can_update_models () {
// given
$genericPaidDSL = new GenericPaidDSL("nmeri");
$user = $this->replicator->modifyInsertion(
1, [], function ($builder) {
return $builder->has(
CartProducts::factory()->has(
Product::factory()->count(5)
)
);
}
)[0];
$this->actingAs($user);
$products = $user->cart->products;
$this->getContainer()->getClass(TransactionService::class)
->updateModels($genericPaidDSL); // when
// then
$this->assertHandledEvent(
TransactionService::class, TransactionService::EMPTIED_CART
);
foreach ($products as $product)
$this->databaseApi->assertDatabaseHas(
Product::TABLE_NAME, [
"id" => $product->id,
"sold" => true
]);
}
}
Conclusion
In this post, we walked through building and consuming webhooks in a robust, testable way—leveraging Suphle’s rich features to handle common pitfalls with elegance and minimal boilerplate. From outbound request handling to payload parsing, from DSL consistency to transactional safety, Suphle has your back.
If you found this helpful, please consider sharing the article and dropping a star on GitHub. Cheers!