5 core software design principles every developer should know (with examples).
Good software design is essential for creating systems that are maintainable, scalable, and easy to understand. Here are five core software design principles that every developer should know: Single Responsibility Principle The Single Responsibility Principle states that a class should have only one reason to change. This means that a class should have only one responsibility or functionality. If a class has multiple responsibilities, it can lead to tight coupling and make it difficult to modify or extend the class. For example, consider a class called “User” that has methods for authentication, data storage, and logging. This class has multiple responsibilities and can be difficult to maintain. Instead, you could break this class into separate classes, each with a single responsibility, such as “Authenticator”, “DataStore”, and “Logger”. Open-Closed Principle The Open-Closed Principle states that a class should be open for extension but closed for modification. This means that you should be able to add new functionality to a class without modifying its existing code. This principle helps to ensure that changes to a class do not break existing functionality. For example, consider a class called “PaymentGateway” that supports multiple payment methods, such as credit cards and PayPal. Instead of modifying the existing class to add a new payment method, you could create a new class that inherits from the “PaymentGateway” class and adds the new payment method. Liskov Substitution Principle The Liskov Substitution Principle states that subtypes should be substitutable for their base types. This means that any code that uses a base type should be able to work with a subtype without knowing the difference. This principle helps to ensure that inheritance is used correctly and that subtypes are truly substitutable for their base types. For example, consider a class called “Vehicle” and a subclass called “Car”. The “Car” class should be substitutable for the “Vehicle” class, meaning that any code that uses a “Vehicle” object should be able to work with a “Car” object without knowing the difference. Interface Segregation Principle The Interface Segregation Principle states that clients should not be forced to depend on interfaces they do not use. This means that a class should not be forced to implement an interface that has methods it does not need. Instead, the interface should be broken down into smaller, more focused interfaces that are specific to the needs of each client. For example, consider an interface called “Printable” that has methods for printing, scanning, and faxing. A class that only needs to print documents should not be forced to implement the “Printable” interface, which includes methods for scanning and faxing. Instead, the “Printable” interface could be broken down into separate interfaces, such as “Printable”, “Scannable”, and “Faxable”. Dependency Inversion Principle The Dependency Inversion Principle states that high-level modules should not depend on low-level modules, but both should depend on abstractions. This means that a high-level module should not be tightly coupled to a specific low-level module, but instead should depend on an abstraction that defines the interface for the low-level module. For example, consider a class called “Logger” that depends on a specific logging framework, such as Log4j. Instead of tightly coupling the “Logger” class to Log4j, you could define an abstraction, such as a “Logging” interface, that defines the logging methods. The “Logger” class can then depend on the “Logging” interface, which can be implemented by different logging frameworks, such as Log4j or Logback.
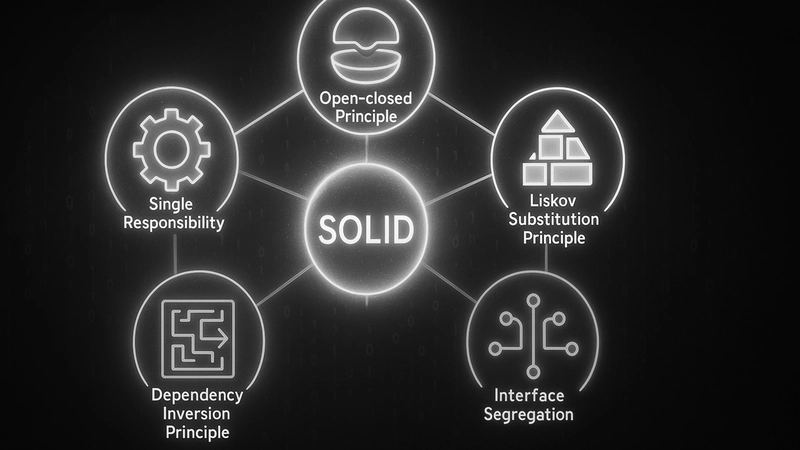
Good software design is essential for creating systems that are maintainable, scalable, and easy to understand. Here are five core software design principles that every developer should know:
Single Responsibility Principle
The Single Responsibility Principle states that a class should have only one reason to change. This means that a class should have only one responsibility or functionality. If a class has multiple responsibilities, it can lead to tight coupling and make it difficult to modify or extend the class.
For example, consider a class called “User” that has methods for authentication, data storage, and logging. This class has multiple responsibilities and can be difficult to maintain. Instead, you could break this class into separate classes, each with a single responsibility, such as “Authenticator”, “DataStore”, and “Logger”.
Open-Closed Principle
The Open-Closed Principle states that a class should be open for extension but closed for modification. This means that you should be able to add new functionality to a class without modifying its existing code. This principle helps to ensure that changes to a class do not break existing functionality.
For example, consider a class called “PaymentGateway” that supports multiple payment methods, such as credit cards and PayPal. Instead of modifying the existing class to add a new payment method, you could create a new class that inherits from the “PaymentGateway” class and adds the new payment method.
Liskov Substitution Principle
The Liskov Substitution Principle states that subtypes should be substitutable for their base types. This means that any code that uses a base type should be able to work with a subtype without knowing the difference. This principle helps to ensure that inheritance is used correctly and that subtypes are truly substitutable for their base types.
For example, consider a class called “Vehicle” and a subclass called “Car”. The “Car” class should be substitutable for the “Vehicle” class, meaning that any code that uses a “Vehicle” object should be able to work with a “Car” object without knowing the difference.
Interface Segregation Principle
The Interface Segregation Principle states that clients should not be forced to depend on interfaces they do not use. This means that a class should not be forced to implement an interface that has methods it does not need. Instead, the interface should be broken down into smaller, more focused interfaces that are specific to the needs of each client.
For example, consider an interface called “Printable” that has methods for printing, scanning, and faxing. A class that only needs to print documents should not be forced to implement the “Printable” interface, which includes methods for scanning and faxing. Instead, the “Printable” interface could be broken down into separate interfaces, such as “Printable”, “Scannable”, and “Faxable”.
Dependency Inversion Principle
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules, but both should depend on abstractions. This means that a high-level module should not be tightly coupled to a specific low-level module, but instead should depend on an abstraction that defines the interface for the low-level module.
For example, consider a class called “Logger” that depends on a specific logging framework, such as Log4j. Instead of tightly coupling the “Logger” class to Log4j, you could define an abstraction, such as a “Logging” interface, that defines the logging methods. The “Logger” class can then depend on the “Logging” interface, which can be implemented by different logging frameworks, such as Log4j or Logback.