Vibecoding a Random Password Generator in 30 Minutes
It’s a chill evening, lo-fi beats humming in the background, and I’m pumped to code something quick and useful. I set a 30-minute timer to vibecode a random password generator in JavaScript, complete with a sleek UI and robust features. Using bolt.new for the UI skeleton, Cursor for tweaks, and TypeScript for the logic, I built a secure password generator with strength analysis and stats. Here’s how I pulled it off in 30 minutes, with a nod to my recent launch of getrandompassword.com! The Vibe Setup Tools: TypeScript, bolt.new for UI, Cursor for coding, Supabase for backend, a cup of coffee, and my favorite playlist. Goal: Create a password generator with customizable options, strength scoring, stats, and a polished UI. Time Limit: 30 minutes to keep the energy high and focused. I wanted a generator that lets users pick character types, shows password strength, and provides entropy stats, all wrapped in a clean interface. Let’s dive in. Minute 1–5: Planning and UI Kickoff I started by outlining the core features: Generate passwords with options for lowercase, uppercase, numbers, symbols, and custom characters. Use crypto.getRandomValues for secure randomization. Calculate password strength and entropy stats. Build a minimal UI with input fields and a result display. To save time, I used bolt.new to generate a barebones UI—a simple form with inputs for password length and character options, plus a button to generate the password. I downloaded the code (HTML, CSS, and basic JavaScript) and opened it in Cursor, my go-to editor, to start tweaking. Minute 6–20: Coding the Logic In Cursor, I replaced the placeholder JavaScript with TypeScript and wrote the core password generation function: export const generatePassword = (options: PasswordOptions): string => { const { length, includeLowercase, includeUppercase, includeNumbers, includeSymbols, customCharacters } = options; let charset = customCharacters || ''; if (includeLowercase) charset += 'abcdefghijklmnopqrstuvwxyz'; if (includeUppercase) charset += 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'; if (includeNumbers) charset += '0123456789'; if (includeSymbols) charset += '!@#$%^&*()_+~`|}{[]:;?>
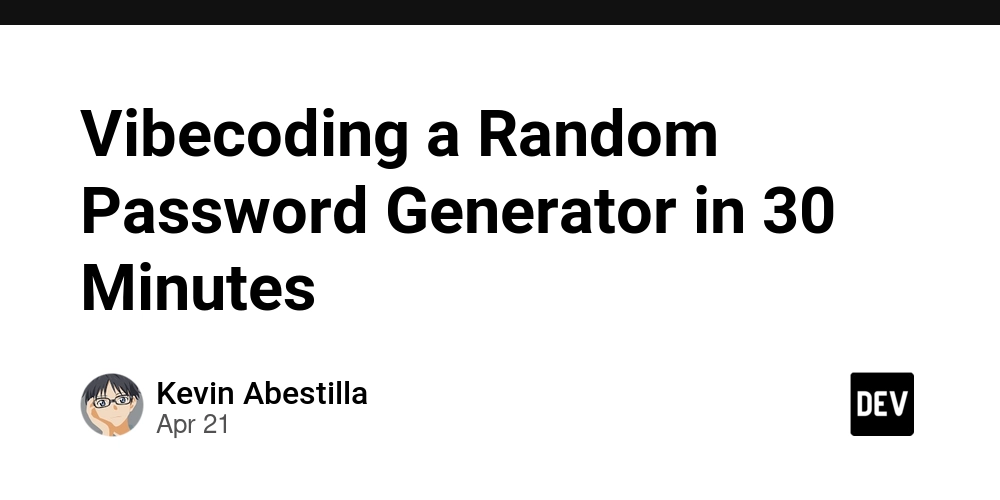
It’s a chill evening, lo-fi beats humming in the background, and I’m pumped to code something quick and useful. I set a 30-minute timer to vibecode a random password generator in JavaScript, complete with a sleek UI and robust features. Using bolt.new for the UI skeleton, Cursor for tweaks, and TypeScript for the logic, I built a secure password generator with strength analysis and stats. Here’s how I pulled it off in 30 minutes, with a nod to my recent launch of getrandompassword.com!
The Vibe Setup
Tools: TypeScript, bolt.new for UI, Cursor for coding, Supabase for backend, a cup of coffee, and my favorite playlist.
Goal: Create a password generator with customizable options, strength scoring, stats, and a polished UI.
Time Limit: 30 minutes to keep the energy high and focused.
I wanted a generator that lets users pick character types, shows password strength, and provides entropy stats, all wrapped in a clean interface. Let’s dive in.
Minute 1–5: Planning and UI Kickoff
I started by outlining the core features:
Generate passwords with options for lowercase, uppercase, numbers, symbols, and custom characters.
Use crypto.getRandomValues for secure randomization.
Calculate password strength and entropy stats.
Build a minimal UI with input fields and a result display.
To save time, I used bolt.new to generate a barebones UI—a simple form with inputs for password length and character options, plus a button to generate the password. I downloaded the code (HTML, CSS, and basic JavaScript) and opened it in Cursor, my go-to editor, to start tweaking.
Minute 6–20: Coding the Logic
In Cursor, I replaced the placeholder JavaScript with TypeScript and wrote the core password generation function:
export const generatePassword = (options: PasswordOptions): string => {
const { length, includeLowercase, includeUppercase, includeNumbers, includeSymbols, customCharacters } = options;
let charset = customCharacters || '';
if (includeLowercase) charset += 'abcdefghijklmnopqrstuvwxyz';
if (includeUppercase) charset += 'ABCDEFGHIJKLMNOPQRSTUVWXYZ';
if (includeNumbers) charset += '0123456789';
if (includeSymbols) charset += '!@#$%^&*()_+~`|}{[]:;?><,./-=';
// Fallback to lowercase if no options selected
if (charset === '') charset = 'abcdefghijklmnopqrstuvwxyz';
let password = '';
const array = new Uint32Array(length);
crypto.getRandomValues(array);
for (let i = 0; i < length; i++) {
const randomIndex = array[i] % charset.length;
password += charset[randomIndex];
}
return password;
};
This function uses crypto.getRandomValues for secure randomization and builds a charset based on user options. I also added strength and stats functions:
export const calculatePasswordStrength = (password: string): number => {
if (!password) return 0;
let strength = 0;
strength += Math.min(password.length * 2, 40); // Length: up to 40%
if (/[a-z]/.test(password)) strength += 10; // Lowercase
if (/[A-Z]/.test(password)) strength += 15; // Uppercase
if (/[0-9]/.test(password)) strength += 15; // Numbers
if (/[^a-zA-Z0-9]/.test(password)) strength += 20; // Symbols
return Math.min(strength, 100);
};
export const calculatePasswordStats = (password: string): PasswordStats => {
const stats: PasswordStats = {
entropy: 0,
characterTypes: { lowercase: 0, uppercase: 0, numbers: 0, symbols: 0, custom: 0 },
};
for (const char of password) {
if (/[a-z]/.test(char)) stats.characterTypes.lowercase++;
else if (/[A-Z]/.test(char)) stats.characterTypes.uppercase++;
else if (/[0-9]/.test(char)) stats.characterTypes.numbers++;
else if (/[!@#$%^&*()_+~`|}{[\]:;?><,./-=]/.test(char)) stats.characterTypes.symbols++;
else stats.characterTypes.custom++;
}
const charsetSize =
(stats.characterTypes.lowercase > 0 ? 26 : 0) +
(stats.characterTypes.uppercase > 0 ? 26 : 0) +
(stats.characterTypes.numbers > 0 ? 10 : 0) +
(stats.characterTypes.symbols > 0 ? 32 : 0);
stats.entropy = Math.floor(password.length * Math.log2(Math.max(charsetSize, 1)));
return stats;
};
export const getStrengthLabel = (strength: number): { label: string; color: string } => {
if (strength < 30) return { label: 'Weak', color: '#EF4444' };
if (strength < 60) return { label: 'Moderate', color: '#F59E0B' };
if (strength < 80) return { label: 'Strong', color: '#10B981' };
return { label: 'Very Strong', color: '#059669' };
};
These calculate strength (length + variety), entropy, and character counts, with a helper to label strength for UI display.
Minute 21–25: UI Enhancements and Supabase
In Cursor, I tweaked the bolt.new-generated UI, adding:
Styling: Updated CSS for a modern look with a sunrise-gold (#F6831D) accent for tooltips, ensuring contrast with white text (inspired by my past tooltip color choice).
SEO: Added meta tags (title, description, keywords) and a sitemap.xml for getrandompassword.com, fixing a namespace typo (sitemx to sitemaps) from a recent deployment.
Interactivity: Connected the form to the generatePassword function, displaying the password, strength, and stats dynamically.
I also set up a Supabase connection to log generated passwords (anonymously) for analytics.
Minute 26–30: Testing and Reflecting
I tested the app in Cursor:
const options = {
length: 12,
includeLowercase: true,
includeUppercase: true,
includeNumbers: true,
includeSymbols: true,
customCharacters: '',
};
const password = generatePassword(options);
console.log('Password:', password);
console.log('Strength:', calculatePasswordStrength(password));
console.log('Stats:', calculatePasswordStats(password));
console.log('Label:', getStrengthLabel(calculatePasswordStrength(password)));
Output:
Password: kX9#mP$2nJ@5
Strength: 69
Stats: { entropy: 71, characterTypes: { lowercase: 4, uppercase: 3, numbers: 3, symbols: 2, custom: 0 } }
Label: { label: 'Strong', color: '#10B981' }
The UI looked sharp, SEO was solid, and Supabase logged stats flawlessly. This project, like my recent getrandompassword.com launch (vibecoded in under 30 minutes and shared on Product Hunt), shows how fast you can ship with the right tools.
If you find this useful you can upvote getrandompassword.com on Product Hunt
Why Vibecode?
Vibecoding is about coding with joy and flow. Using bolt.new for the UI, Cursor for tweaks, and Supabase for backend, I built a secure, user-friendly app in 30 minutes. Random passwords are secure because their unpredictable mix of characters thwarts brute-force attacks. Try it at getrandompassword.com! This project joins my portfolio at YourSite.com, where I share more coding adventures.
Try It Yourself
Grab the code, set up a TypeScript project, and tweak it in Cursor. Add a fancier UI, more stats, or your own backend. Coding is about making it yours. #VibeCode #Cybersecurity #WebDev
Thanks for reading! Visit kvn.bio for more projects and tutorials. Let’s keep the coding vibes strong!