Title: "From Chaos to Clarity: How I Mastered Clean Code in JavaScript"
As developers, we’ve all been there—staring at a tangled mess of JavaScript code, wondering where things went wrong. A year ago, my projects felt like a house of cards: one small change, and everything collapsed. Today, I write code that’s clean, maintainable, and even a little fun to revisit. Here’s how I went from chaos to clarity with clean code principles in JavaScript. Embrace Meaningful Naming Names matter. Early on, I’d write variables like x or temp. Big mistake. Now, I name things to reflect their purpose. Instead of data, I use userProfile or orderDetails. Functions get descriptive names too—like calculateTotalPrice instead of calc. Clear names reduce mental overhead and make your code self-documenting. // Bad let x = 100; function calc(a) { return a * 2; } // Good let maxRetries = 100; function calculateDoubleValue(number) { return number * 2; } Keep Functions Small and Focused I used to write monster functions that did everything—fetch data, process it, update the UI. Now, I follow the single-responsibility principle. Each function does one thing well. If it’s longer than 10-15 lines, I refactor. // Bad function handleUser() { fetchUserData(); updateUI(); logActivity(); } // Good function fetchUserData() { /* ... / } function updateUI(user) { / ... / } function logActivity(user) { / ... */ } Avoid Magic Numbers and Strings Hardcoding values like 7 or "admin" used to haunt me during debugging. Now, I use constants to give context to numbers and strings. It’s easier to update and understand. Example: // Bad if (user.role === "admin") { /* ... */ } // Good const ROLES = { ADMIN: "admin", USER: "user" }; if (user.role === ROLES.ADMIN) { /* ... */ }
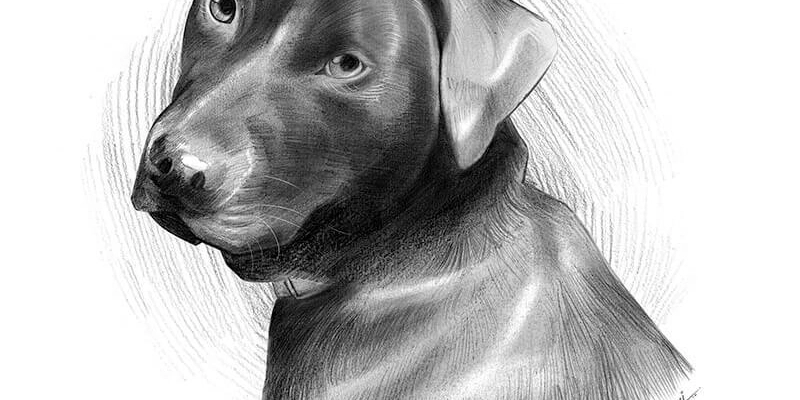
As developers, we’ve all been there—staring at a tangled mess of JavaScript code, wondering where things went wrong. A year ago, my projects felt like a house of cards: one small change, and everything collapsed. Today, I write code that’s clean, maintainable, and even a little fun to revisit. Here’s how I went from chaos to clarity with clean code principles in JavaScript.
- Embrace Meaningful Naming Names matter. Early on, I’d write variables like x or temp. Big mistake. Now, I name things to reflect their purpose. Instead of data, I use userProfile or orderDetails. Functions get descriptive names too—like calculateTotalPrice instead of calc. Clear names reduce mental overhead and make your code self-documenting. // Bad let x = 100; function calc(a) { return a * 2; }
// Good
let maxRetries = 100;
function calculateDoubleValue(number) { return number * 2; }
- Keep Functions Small and Focused I used to write monster functions that did everything—fetch data, process it, update the UI. Now, I follow the single-responsibility principle. Each function does one thing well. If it’s longer than 10-15 lines, I refactor. // Bad function handleUser() { fetchUserData(); updateUI(); logActivity(); }
// Good
function fetchUserData() { /* ... / }
function updateUI(user) { / ... / }
function logActivity(user) { / ... */ }
- Avoid Magic Numbers and Strings Hardcoding values like 7 or "admin" used to haunt me during debugging. Now, I use constants to give context to numbers and strings. It’s easier to update and understand.
Example:
// Bad
if (user.role === "admin") { /* ... */ }
// Good
const ROLES = { ADMIN: "admin", USER: "user" };
if (user.role === ROLES.ADMIN) { /* ... */ }